How to implement AJAX?
Implementing AJAX (Asynchronous JavaScript and XML) in PHP allows you to create dynamic and interactive web applications where data can be loaded or updated without requiring a full page refresh. Here’s a step-by-step guide on how to implement AJAX in PHP:
- Include jQuery or JavaScript Library:
To simplify AJAX requests, it’s common to use a JavaScript library like jQuery. Include the library in your HTML file:
```html <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> ```
- Create an HTML Structure:
Design your HTML structure with elements that will be updated or loaded dynamically. For example, you can have a `<div>` to display fetched data:
```html <div id="result"></div> ```
- Write JavaScript Code:
Use JavaScript to handle the AJAX request and update the HTML elements. In this example, we use jQuery to send a GET request to a PHP script and update the `<div>` with the response:
```javascript $(document).ready(function () { $("#loadData").click(function () { $.ajax({ url: "ajax.php", // PHP script URL type: "GET", success: function (response) { $("#result").html(response); // Update the result div }, error: function () { alert("Error fetching data."); } }); }); }); ```
- Create a PHP Script:
Create a PHP script (`ajax.php` in this case) that processes the AJAX request and returns data. You can use PHP to query a database, process data, and generate a response:
```php <?php // Your PHP code to fetch or process data $data = "Data fetched from the server."; echo $data; ?> ```
- Trigger the AJAX Request:
Trigger the AJAX request by interacting with an element, such as a button, as shown in the JavaScript code above.
- Handle AJAX Response:
The response from the PHP script is displayed in the `<div>` with the `id=”result”` in this example. You can customize how the response is displayed based on your application’s requirements.
Implementing AJAX in PHP enables you to build more responsive and user-friendly web applications by asynchronously loading or updating content. You can extend this basic example to handle more complex scenarios, including sending data to the server, error handling, and handling different types of HTTP requests (GET, POST, etc.).
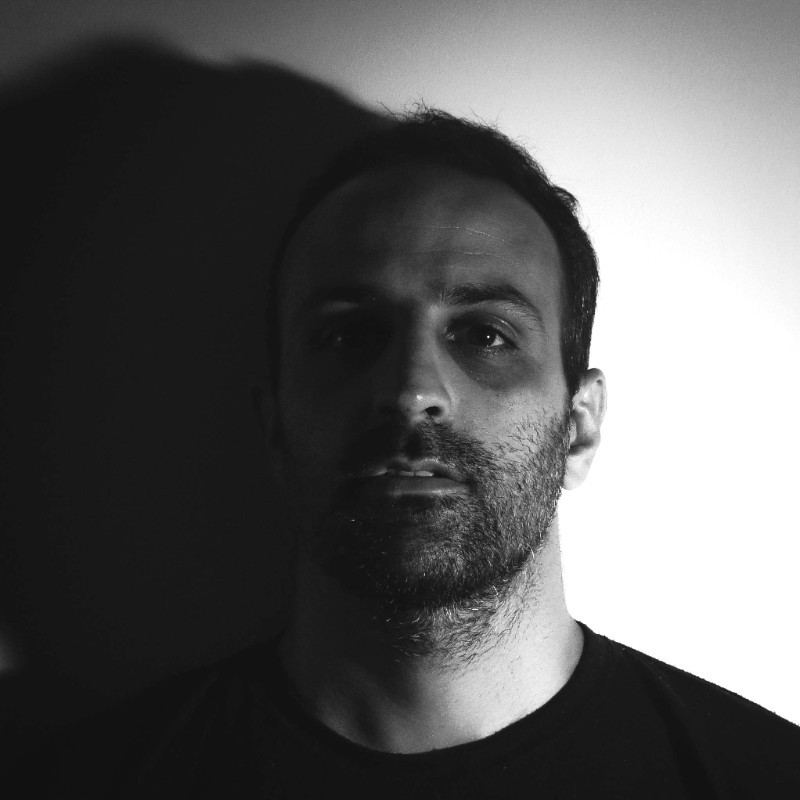
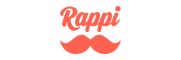