PHP’s intval() Function: Converting Values to Integers
PHP’s intval() function is a powerful tool for converting various types of values into integers. Whether you’re dealing with strings, floats, or other data types, intval() simplifies the process of obtaining an integer representation. This function is particularly useful in scenarios where type consistency is crucial, such as in database operations or numerical calculations.
Why Use intval()?
The primary purpose of intval() is to ensure that a value is safely converted into an integer. This is especially important in PHP, which is a loosely typed language. Without strict type enforcement, it’s easy to encounter unexpected behaviors when dealing with different data types. intval() mitigates these issues by providing a consistent way to handle numbers.
Syntax and Basic Usage
The syntax for the intval() function is straightforward:
```php int intval ( mixed $value [, int $base = 10 ] ) ```
- $value: The value to be converted to an integer. This can be a string, float, boolean, or even null.
- $base: An optional parameter that specifies the base of the number in the string. It ranges from 2 to 36 and defaults to 10.
Example: Converting a String to an Integer
```php <?php $stringValue = "123"; $integerValue = intval($stringValue); echo $integerValue; // Outputs: 123 ?> ```
In this example, the string “123” is converted to the integer 123.
Handling Different Data Types
Strings
When dealing with strings, intval() processes the string and stops at the first non-numeric character. This means only the numeric portion at the beginning of the string is considered.
```php <?php $stringValue = "123abc"; $integerValue = intval($stringValue); echo $integerValue; // Outputs: 123 ?> ```
Floats
When a float is passed to intval(), the function truncates the decimal portion and returns the integer part.
```php <?php $floatValue = 123.456; $integerValue = intval($floatValue); echo $integerValue; // Outputs: 123 ?> ```
Booleans
For boolean values, intval() converts true to 1 and false to 0.
```php <?php $trueValue = true; $falseValue = false; echo intval($trueValue); // Outputs: 1 echo intval($falseValue); // Outputs: 0 ?> ```
Common Use Cases
User Input Validation
When processing user input, it’s crucial to ensure that the data conforms to expected types. intval() can help sanitize inputs by converting them to integers, preventing potential security issues or errors.
```php <?php $userInput = $_GET['id']; $validatedId = intval($userInput); ?> ```
Database Queries
In SQL queries, integer values are often required for certain operations. Using intval() ensures that values passed into the query are integers, avoiding SQL injection vulnerabilities.
```php <?php $userId = intval($_GET['user_id']); $query = "SELECT * FROM users WHERE id = $userId"; ?> ```
Limitations and Considerations
While intval() is useful, it’s important to be aware of its limitations:
- Base Conversion: The function can handle base conversions, but this feature is often overlooked. Be cautious when converting from bases other than 10.
- Precision Loss: For floats, the function truncates the decimal part, which can lead to precision loss.
- Empty Values: If the input value is empty or non-numeric, intval() returns 0. This behavior can be misleading if not properly handled.
Conclusion
PHP’s intval() function is an essential tool for developers who need to ensure type consistency in their applications. By understanding its functionality and limitations, you can effectively use intval() to handle various data types and prevent common issues. Whether you’re a beginner or an experienced developer, mastering intval() will enhance your PHP coding skills.
Further Reading
Table of Contents
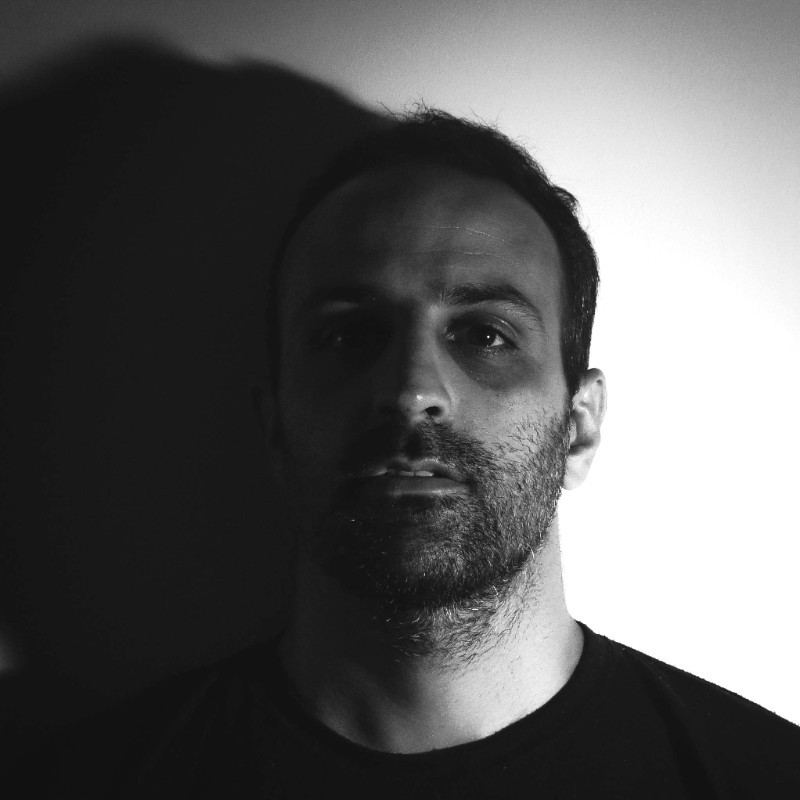
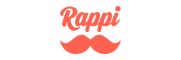