How to use loops (for, while, foreach)?
Loops are essential in PHP for repetitive tasks, and they allow you to execute a block of code multiple times. PHP provides three main types of loops: “for,” “while,” and “foreach.” Here’s a comprehensive guide on how to use these loops in PHP:
- “for” Loop:
A “for” loop is used when you know in advance how many times you want to execute a block of code. It consists of three parts: initialization, condition, and increment.
```php for (initialization; condition; increment) { // Code to be repeated } ```
Example:
```php for ($i = 1; $i <= 5; $i++) { echo "Iteration $i<br>"; } ```
This “for” loop will execute the code block five times, printing “Iteration 1” through “Iteration 5.”
- “while” Loop:
A “while” loop is used when you want to execute a block of code as long as a specific condition is true. It checks the condition before each iteration.
```php while (condition) { // Code to be repeated } ```
Example:
```php $num = 1; while ($num <= 5) { echo "Iteration $num<br>"; $num++; } ```
This “while” loop will execute the code block as long as the condition `$num <= 5` is true, printing “Iteration 1” through “Iteration 5.”
- “foreach” Loop:
A “foreach” loop is designed specifically for iterating over arrays and objects. It simplifies the process of accessing each element in the array or object.
```php foreach ($array as $element) { // Code to work with $element } ```
Example:
```php $colors = ["red", "green", "blue"]; foreach ($colors as $color) { echo "Color: $color<br>"; } ```
This “foreach” loop will iterate over the `$colors` array, printing each color.
These loops are crucial for automating repetitive tasks, iterating through arrays, and processing data in PHP. Depending on the specific task and the type of data you’re working with, you can choose the most appropriate loop for the job to efficiently manage and manipulate data within your PHP scripts.
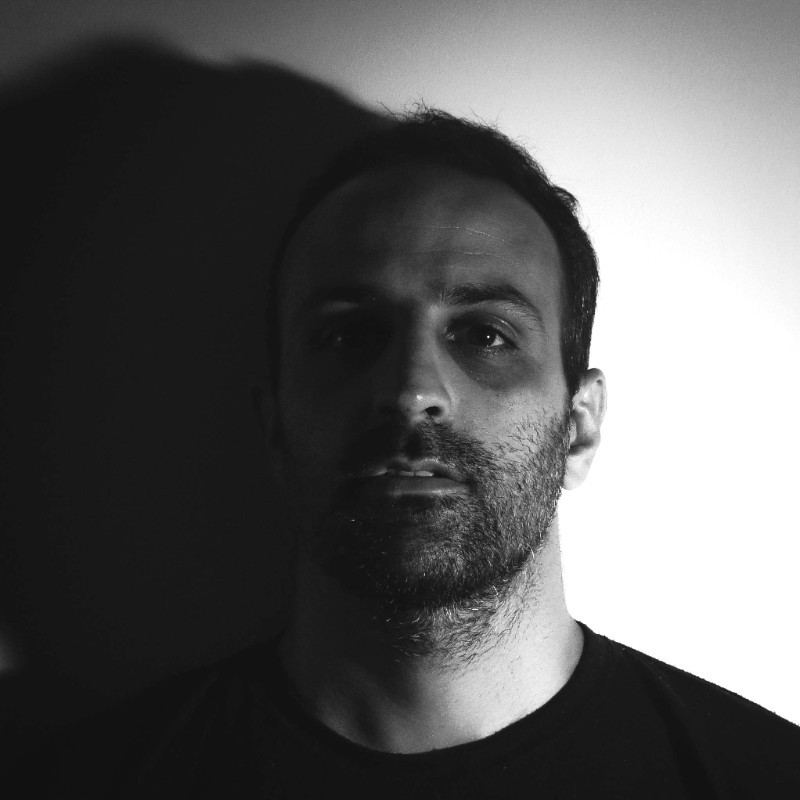
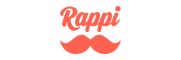