How to use namespaces?
Namespaces in PHP are a way to organize and encapsulate classes, functions, and constants to prevent naming conflicts in large projects or when integrating third-party libraries. Namespaces provide a hierarchical system for grouping related code under a unique identifier. Here’s how to use namespaces effectively in PHP:
- Declaring a Namespace:
– To declare a namespace in PHP, use the `namespace` keyword, followed by the namespace name, at the beginning of your PHP file. Namespace names follow a similar convention to file paths, using backslashes `\` as separators.
```php namespace MyNamespace; ```
- Defining Classes within a Namespace:
– You can define classes within a namespace by specifying the namespace at the beginning of the class definition.
```php namespace MyNamespace; class MyClass { // Class members here } ```
- Using Namespaced Classes:
– To use a namespaced class in your code, you need to either specify the fully qualified class name (including the namespace) or import the namespace with the `use` statement.
```php // Using fully qualified class name $obj = new \MyNamespace\MyClass(); // Importing the namespace use MyNamespace\MyClass; $obj = new MyClass(); ```
- Aliasing Namespaces:
– You can also provide an alias for a namespace or class to simplify usage, especially if you’re working with long namespaces or multiple namespaces with the same name.
```php use MyNamespace\MyClass as CustomClass; $obj = new CustomClass(); ```
- Global Namespace:
– Code that doesn’t specify a namespace resides in the global namespace. You can access global namespace classes by prefixing them with a backslash `\`.
```php $obj = new \GlobalNamespace\MyClass(); ```
Namespaces are valuable for structuring code in large PHP projects and for avoiding naming collisions when incorporating third-party libraries. They improve code readability, maintainability, and help prevent unexpected conflicts, making them an essential feature of modern PHP development.
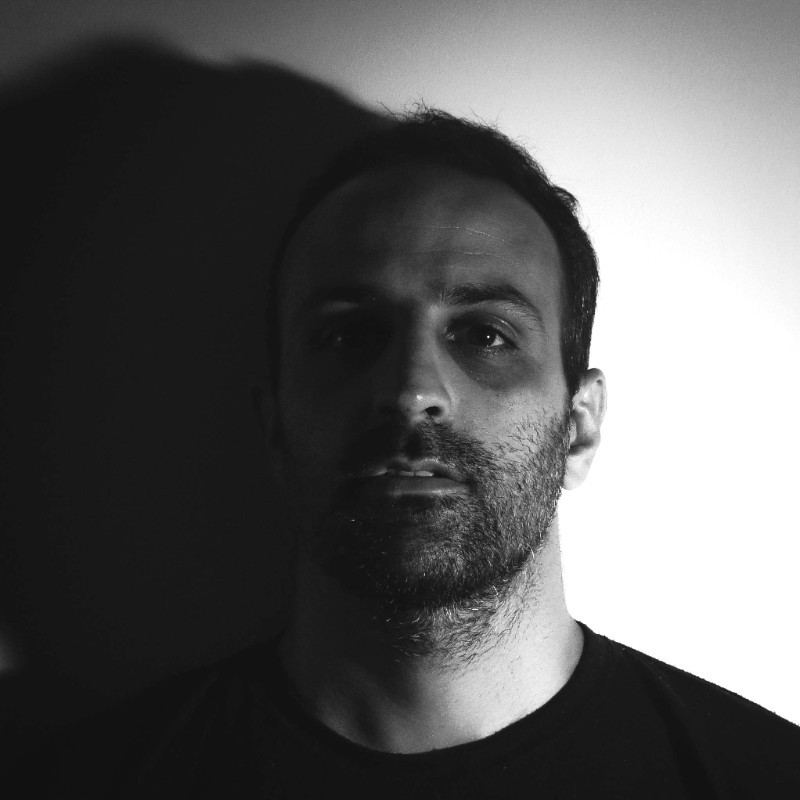
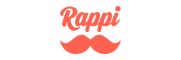