PHP’s strstr() Function: Searching Within Strings
In PHP, the strstr() function is a powerful tool for searching within strings. This function allows you to locate the first occurrence of a substring within a string and can be quite useful in various scenarios, from simple text processing to more complex string manipulations. In this blog post, we’ll delve into how strstr() works, its different use cases, and provide practical examples to illustrate its capabilities.
Understanding strstr()
The strstr() function searches for the first occurrence of a string inside another string. It returns the portion of the string starting from the first occurrence of the search string to the end of the string. If the search string is not found, strstr() returns false.
Syntax
```php strstr($haystack, $needle, $before_needle = false); ```
- $haystack: The string to search within.
- $needle: The substring to search for.
- $before_needle: Optional. If set to true, strstr() returns the part of the haystack before the needle.
Basic Example
Let’s look at a basic example to understand how strstr() works:
```php <?php $haystack = "Hello, welcome to the world of PHP!"; $needle = "welcome"; $result = strstr($haystack, $needle); echo $result; ?> ```
Output:
``` welcome to the world of PHP! ```
In this example, strstr() finds the substring “welcome” in the haystack and returns the remainder of the string starting from “welcome”.
Using the before_needle Parameter
The before_needle parameter allows you to get the part of the haystack before the needle. When this parameter is set to true, strstr() returns everything before the first occurrence of the needle.
Example with before_needle
```php <?php $haystack = "Hello, welcome to the world of PHP!"; $needle = "welcome"; $result = strstr($haystack, $needle, true); echo $result; ?> ```
Output:
``` Hello, ```
Here, strstr() returns everything before the substring “welcome”.
Case Sensitivity
The strstr() function is case-sensitive. If you need a case-insensitive search, consider using stristr(), which works similarly but ignores case differences.
Case Insensitive Search Example
```php <?php $haystack = "Hello, Welcome to the world of PHP!"; $needle = "welcome"; $result = stristr($haystack, $needle); echo $result; ?> ```
Output:
``` Welcome to the world of PHP! ```
In this case, stristr() finds the needle regardless of the case and returns the remaining string.
Practical Use Cases
- Extracting Parts of a String: Use strstr() to get specific parts of a string for further processing or display.
- Validating Input: Check if a certain substring exists within user input or data before processing it.
- String Manipulation: Combine strstr() with other string functions to perform complex text manipulations.
Conclusion
The strstr() function in PHP is a versatile tool for searching and extracting substrings within strings. Whether you need to get a portion of a string from a specific point or search in a case-insensitive manner, strstr() and its counterpart stristr() can help you achieve your goals effectively.
For more information on string handling in PHP, consider exploring the following resources:
Further Reading
Table of Contents
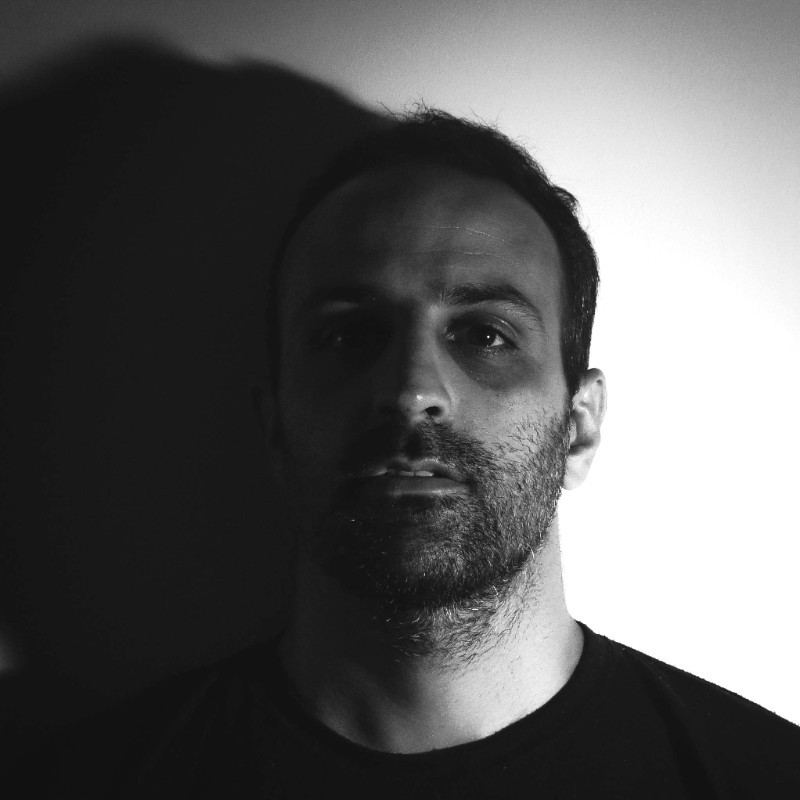
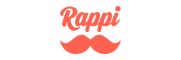