PHP’s substr_replace() Function: Replacing Parts of Strings
String manipulation is a common task in PHP development. Whether you’re sanitizing user input, formatting data, or dynamically generating content, string operations are essential. One powerful function in PHP for modifying strings is substr_replace(). This function allows you to replace a portion of a string with another string, providing fine control over the manipulation process.
In this guide, we’ll explore the substr_replace() function, including its syntax, parameters, and practical examples. By the end, you’ll understand how to effectively use this function in your PHP projects.
Understanding substr_replace()
Syntax
The substr_replace() function has the following syntax:
```php substr_replace(string $string, string $replacement, int $start[, int $length = null]): string ```
Parameters
- $string: The original string that you want to modify.
- $replacement: The string that will replace a portion of the original string.
- $start: The position in the original string where the replacement will begin. A positive number starts from the beginning, while a negative number starts from the end.
- $length (optional): The number of characters in the original string to replace. If omitted, all characters from $start to the end of the string will be replaced.
Return Value
The function returns a new string with the specified replacements.
Practical Examples
Replacing a Substring
Let’s start with a basic example where we replace a portion of a string:
```php <?php $original = "Hello, world!"; $replacement = "PHP"; $result = substr_replace($original, $replacement, 7, 5); echo $result; // Outputs: "Hello, PHP!" ?> ```
In this example, the word “world” is replaced with “PHP”. The $start parameter is 7, and the $length is 5, which corresponds to the position and length of “world” in the original string.
Inserting a String
You can also use substr_replace() to insert a string at a specific position without removing any characters:
```php <?php $original = "Hello, !"; $replacement = "world"; $result = substr_replace($original, $replacement, 7, 0); echo $result; // Outputs: "Hello, world!" ?> ```
Here, the $length parameter is 0, indicating that no characters should be removed. Instead, “world” is inserted at position 7.
Replacing from the End of a String
The $start parameter can be negative, allowing you to count from the end of the string:
```php <?php $original = "This is a test."; $replacement = "simple"; $result = substr_replace($original, $replacement, -5, 4); echo $result; // Outputs: "This is a simple." ?> ```
In this case, -5 indicates the starting point is 5 characters from the end of the string. The length 4 specifies that “test” should be replaced with “simple”.
When to Use substr_replace()
The substr_replace() function is ideal for scenarios where you need to precisely control string replacements. It’s particularly useful when:
- Updating specific parts of a string: For example, replacing placeholders in a template.
- Sanitizing input: Replacing certain parts of user input to prevent security issues.
- Data formatting: Modifying specific sections of formatted data strings.
Conclusion
The substr_replace() function in PHP is a versatile tool for replacing parts of strings with ease and precision. Whether you’re working on data formatting, user input sanitization, or dynamic content generation, understanding how to leverage this function can greatly enhance your PHP development skills.
By practicing with the examples provided and experimenting with different scenarios, you can master the use of substr_replace() and incorporate it effectively into your projects.
Further Reading
Table of Contents
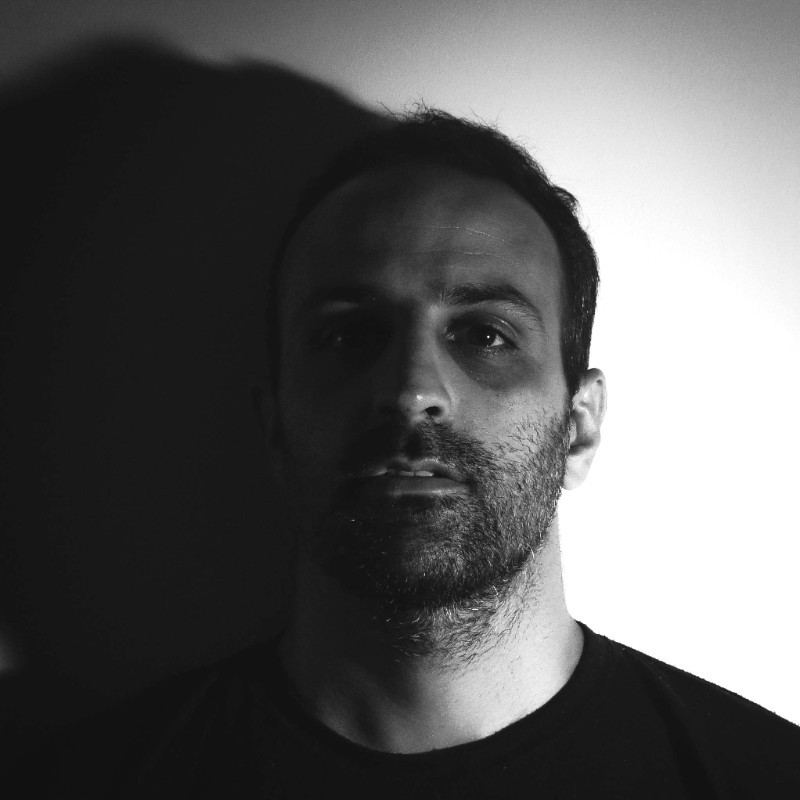
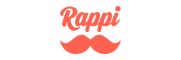