How to upload files?
Uploading files in PHP is a common requirement for many web applications, such as content management systems and file-sharing platforms. To allow users to upload files securely, follow these steps:
- HTML Form:
– Create an HTML form with the `enctype` attribute set to `”multipart/form-data”`. This attribute is essential for file uploads. Include an input field with `type=”file”` to allow users to select files for upload.
```html <form action="upload.php" method="POST" enctype="multipart/form-data"> <input type="file" name="fileToUpload" id="fileToUpload"> <input type="submit" value="Upload File" name="submit"> </form> ```
- PHP Handling:
– In your PHP script (e.g., `upload.php`), retrieve the uploaded file using the `$_FILES` super global array. Access the file using `$_FILES[“fileToUpload”]`, where “fileToUpload” should match the `name` attribute of your input field.
```php $file = $_FILES["fileToUpload"]; ```
- Validation:
– Always validate the uploaded file to ensure it meets your application’s requirements. You can check file types, sizes, and perform any other custom validations. This step is crucial to prevent security issues like uploading malicious files.
- Move Uploaded File:
– To save the uploaded file to your server, use the `move_uploaded_file()` function. Specify the temporary location of the file (usually in the PHP temporary directory) and the desired destination directory.
```php $targetDirectory = "uploads/"; $targetFile = $targetDirectory . basename($file["name"]); if (move_uploaded_file($file["tmp_name"], $targetFile)) { echo "File is uploaded successfully."; } else { echo "Sorry, there was an error uploading your file."; } ```
- Security Considerations:
– Ensure that uploaded files are not directly executable, restrict file types, and prevent overwriting existing files. Sanitize user input and avoid using user-submitted data for file names.
By following these steps and incorporating security measures, you can implement a secure and functional file upload feature in your PHP web application, allowing users to share files safely and efficiently.
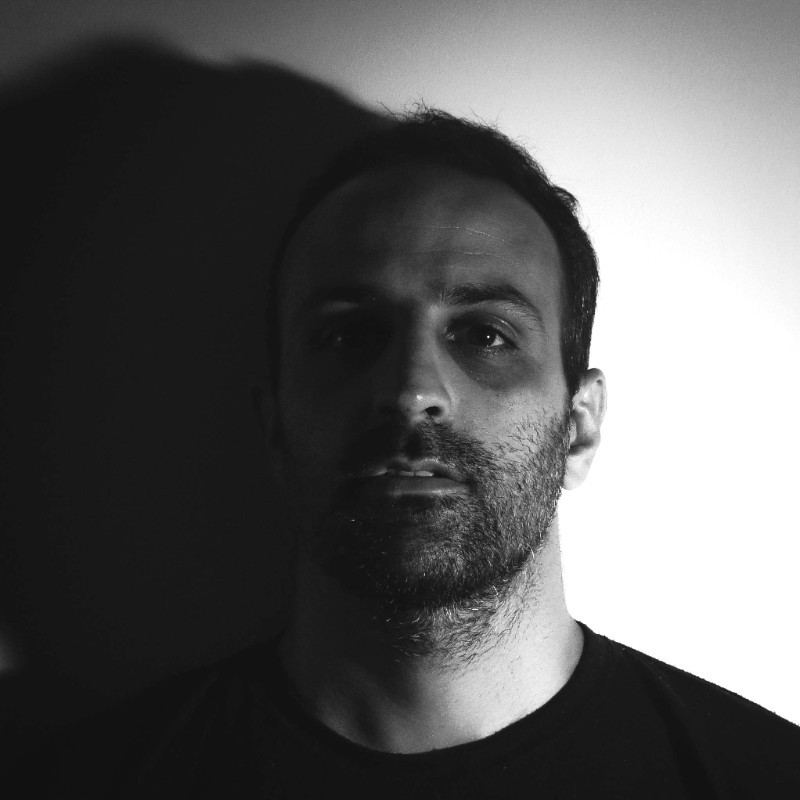
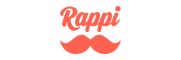