How to use websockets in Python?
WebSockets provide a persistent, low-latency bidirectional communication channel over a single TCP connection. They’re commonly used in applications that require real-time updates, such as chat applications or live sports updates.
- Using the `websocket` library:
For simple WebSocket clients, the `websocket` library is suitable. Install it with `pip install websocket-client`.
Example of a simple WebSocket client:
```python import websocket def on_message(ws, message): print(f"Received: {message}") ws = websocket.WebSocketApp("ws://example.com/websocket", on_message=on_message) ws.run_forever() ```
- Using `websockets` with `asyncio`:
For a more asynchronous approach, the `websockets` library, combined with Python’s native `asyncio`, is preferred. Install it with `pip install websockets`.
Example of an asynchronous WebSocket server:
```python import asyncio import websockets async def handler(websocket, path): message = await websocket.recv() print(f"Received: {message}") await websocket.send(f"Echo: {message}") start_server = websockets.serve(handler, "localhost", 8765) asyncio.get_event_loop().run_until_complete(start_server) asyncio.get_event_loop().run_forever() ```
- Integrating with Web Frameworks:
Popular Python web frameworks like Django (via Django Channels) and Flask (via Flask-SocketIO) offer WebSocket support, making it convenient to incorporate real-time features into web applications.
WebSockets are crucial for real-time communication in modern web applications. Python, with its diverse libraries and frameworks, offers robust tools for both WebSocket clients and servers. Whether you’re aiming for a simple real-time notification system or a fully-fledged chat application, Python has got you covered with its versatile WebSocket support. Ensure to choose the library or framework that best suits the needs and scale of your project.
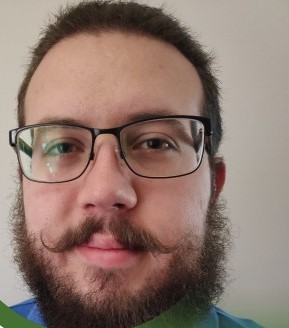
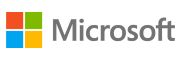