React Native and Apple Music: Integrating Music Playback
In the ever-evolving landscape of app development, React Native stands out as a powerful framework for building cross-platform mobile applications. Its ability to combine the efficiency of JavaScript with the performance of native code has made it a favorite among developers. When it comes to incorporating multimedia features like music playback into React Native apps, integration with popular platforms like Apple Music becomes crucial. In this guide, we’ll delve into the process of seamlessly integrating music playback functionality from Apple Music into React Native applications, along with practical examples and insights.
Understanding React Native and Apple Music Integration
Getting Started:
To kickstart our integration journey, ensure you have a basic understanding of React Native and its ecosystem. Install React Native if you haven’t already and set up your development environment.
Exploring Apple Music API:
Apple provides developers with comprehensive documentation and tools to integrate their services into third-party applications. The Apple Music API offers endpoints for accessing various features, including music catalog, playlists, and playback controls. Familiarize yourself with the API documentation to understand its capabilities and endpoints.
Implementation Steps
Authentication:
Before accessing Apple Music’s features, you need to authenticate users with their Apple Music accounts. Utilize Apple’s authentication mechanisms, such as Apple Music API’s JWT (JSON Web Token) authentication, to securely authenticate users and obtain necessary tokens for API requests.
Fetching Music Data:
Once authenticated, leverage the Apple Music API to fetch music data like songs, albums, artists, and playlists. Utilize endpoints like /v1/catalog/{storefront}/search to search for music based on specific criteria or /v1/me/library/songs to retrieve a user’s library.
Implementing Playback Controls:
Integrate playback controls into your React Native app using Apple Music API endpoints like /v1/me/player/play to start playback, /v1/me/player/pause to pause playback, and /v1/me/player/skip-next to skip to the next track. Design intuitive user interfaces for controlling playback within your app.
Example Code Snippets
Authentication Flow:
javascript // Sample code for Apple Music authentication using Apple's JWT mechanism const appleMusicAuth = async () => { // Implement authentication logic here const token = await fetchAppleMusicToken(); return token; };
Fetching Songs:
// Sample code for fetching songs from Apple Music catalog const fetchSongs = async () => { const response = await fetch(`${appleMusicAPI}/v1/catalog/{storefront}/search?q=${query}&limit=${limit}&types=songs`, { headers: { Authorization: `Bearer ${token}`, }, }); const data = await response.json(); return data.results.songs; };
Playback Controls:
// Sample code for controlling playback in React Native app const startPlayback = async (songId) => { await fetch(`${appleMusicAPI}/v1/me/player/play?ids=${songId}`, { method: 'POST', headers: { Authorization: `Bearer ${token}`, }, }); };
Conclusion
Integrating Apple Music’s extensive music catalog and playback capabilities into React Native apps opens up new avenues for creating engaging and immersive experiences for users. By following the steps outlined in this guide and leveraging the power of the Apple Music API, developers can seamlessly incorporate music playback functionality into their applications. Stay innovative, experiment with different features, and elevate your React Native apps with the harmonious sounds of Apple Music.
External Resources:
Table of Contents
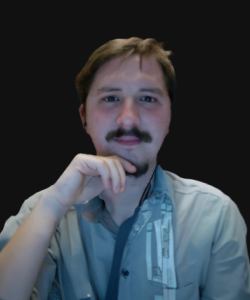
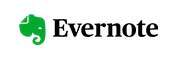