ReactJS and Gatsby: Building Static Site Generator Web Apps
In today’s web development landscape, performance and user experience are paramount. Static site generators (SSGs) like Gatsby, combined with ReactJS, offer a powerful solution for creating fast, modern web applications. This blog explores how to leverage these technologies to build static sites that are both scalable and performant.
What is Gatsby?
Gatsby is a static site generator that leverages ReactJS to create high-performance websites and applications. It pre-builds pages as static HTML files and loads only the necessary JavaScript on demand, leading to faster load times and improved SEO.
Key Features of Gatsby:
– Pre-rendering: Gatsby pre-renders pages at build time, resulting in quicker initial loads.
– GraphQL Data Layer: Gatsby uses GraphQL to pull in data from various sources and integrate it into your site.
– Plugin Ecosystem: With a robust plugin system, Gatsby allows for easy integration of third-party services and tools.
Why Use ReactJS with Gatsby?
ReactJS is a popular JavaScript library for building user interfaces. When combined with Gatsby, it enhances the static site with dynamic functionality while maintaining high performance.
Advantages of ReactJS in Gatsby Projects:
– Component-Based Architecture: React’s component-based approach allows for reusable UI elements and easier maintenance.
– Client-Side Interactivity: While Gatsby pre-renders pages, React enables interactive elements and client-side routing for a seamless user experience.
– Rich Ecosystem: React’s extensive ecosystem of libraries and tools can be integrated into Gatsby for added functionality.
Setting Up a Gatsby Project with ReactJS
Prerequisites
Before getting started, ensure you have Node.js and npm installed on your machine. You can check your installation by running:
```bash node -v npm -v ```
Creating a New Gatsby Site
1. Install Gatsby CLI:
```bash npm install -g gatsby-cli ```
2. Create a New Gatsby Project:
```bash gatsby new my-static-site ```
This command sets up a new Gatsby project in a folder named `my-static-site`.
3. Navigate to the Project Directory:
```bash cd my-static-site ```
4. Start the Development Server:
```bash gatsby develop ```
Visit `http://localhost:8000` in your browser to see your new Gatsby site in action.
Adding React Components
Gatsby uses React to build the user interface. To add a new component:
1. Create a New Component:
Create a new file `src/components/MyComponent.js`:
```jsx import React from 'react'; const MyComponent = () => { return ( <div> <h1>Hello from MyComponent!</h1> </div> ); }; export default MyComponent; ```
2. Use the Component in a Page:
Modify `src/pages/index.js` to include your new component:
```jsx import React from 'react'; import MyComponent from '../components/MyComponent'; const IndexPage = () => { return ( <div> <h1>Welcome to My Gatsby Site</h1> <MyComponent /> </div> ); }; export default IndexPage; ```
Deploying Your Gatsby Site
Once your site is ready, you can deploy it to various hosting platforms. For instance, Gatsby integrates seamlessly with Netlify, Vercel, and GitHub Pages.
Example: Deploying to Netlify
1. Install Netlify CLI:
```bash npm install -g netlify-cli ```
2. Deploy Your Site:
```bash netlify deploy ```
Follow the prompts to connect your Git repository and deploy your site.
Conclusion
Combining ReactJS with Gatsby allows you to build high-performance, static web applications that leverage the best of both technologies. With Gatsby’s static site generation and React’s dynamic capabilities, you can create fast, engaging websites that offer a superior user experience.
Further Reading
Table of Contents
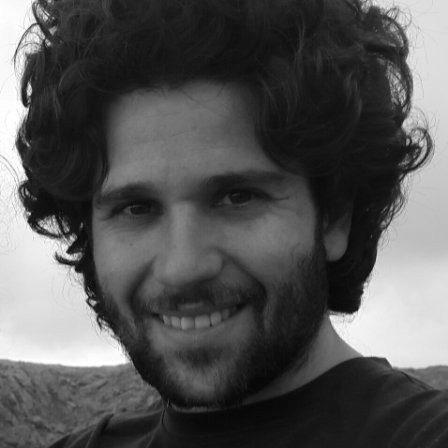
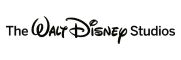