Powering User Feedback: Accessing Battery Status Seamlessly with ReactJS’s useBattery Hook
Building a modern web application comes with its own set of challenges. One unique challenge is efficiently managing power consumption, especially on mobile devices where battery life is crucial. This is where the Battery Status API steps in to allow developers to access information about the host device’s battery status.
But how do we integrate such functionality with a ReactJS application? This is where the custom hook, `useBattery`, comes into play. The `useBattery` hook is a function that we can use to incorporate the Battery Status API into a ReactJS component, and efficiently manage battery usage of our app. In this article, we’ll delve into how we can create and use the `useBattery` hook in our components.
Prerequisite
Before we dive into the code, ensure you have the following:
– Basic understanding of ReactJS and Hooks
– Node.js and npm/yarn installed on your machine
– A new or existing ReactJS application
Battery Status API
The Battery Status API, also known as the Battery API, provides information about the system’s battery charge level, whether the device is charging, how much time is left until the battery is fully discharged or charged.
Before using this API, it’s essential to check if the browser supports it since it’s not supported across all browsers.
if ('getBattery' in navigator) { // The Battery Status API is supported } else { // The Battery Status API is not supported }
Building the useBattery Hook
Now, let’s build our custom `useBattery` hook. The hook will return an object containing battery level, charging state, charging time, and discharging time.
import { useEffect, useState } from 'react'; const useBattery = () => { const [battery, setBattery] = useState({ level: 0, charging: false, chargingTime: 0, dischargingTime: 0 }); useEffect(() => { let batteryStatus; const updateBatteryStatus = (battery) => { setBattery({ level: battery.level, charging: battery.charging, chargingTime:battery.chargingTime, dischargingTime: battery.dischargingTime, }); }; if ('getBattery' in navigator) { navigator.getBattery().then((battery) => { // Updates immediately when the component mounts updateBatteryStatus(battery); // Update when battery status changes batteryStatus = battery; batteryStatus.onchargingchange = () => updateBatteryStatus(battery); batteryStatus.onlevelchange = () => updateBatteryStatus(battery); batteryStatus.ondischargingtimechange = () => updateBatteryStatus(battery); batteryStatus.onchargingtimechange = () => updateBatteryStatus(battery); }); } else { console.log('Battery Status API is not supported'); } battery.chargingTime, dischargingTime: battery.dischargingTime, }); }; // Cleanup function return () => { if (batteryStatus) { batteryStatus.onchargingchange = null; batteryStatus.onlevelchange = null; batteryStatus.ondischargingtimechange = null; batteryStatus.onchargingtimechange = null; } }; }, []); return battery; };
Here, `useEffect` listens for changes in the Battery Status API and updates the battery state whenever any change occurs. We also clean up our event listeners to avoid memory leaks.
Using the useBattery Hook in a Component
With our `useBattery` hook ready, let’s use it in a React component.
import React from 'react'; import useBattery from './useBattery'; const BatteryStatus = () => { const battery = useBattery(); return ( <div> <h2>Battery Status</h2> <p>Battery Level: {battery.level * 100}%</p> <p>Charging: {battery.charging ? 'Yes' : 'No'}</p> <p>Charging Time: {battery.chargingTime} seconds</p> <p>Discharging Time: {battery.dischargingTime} seconds</p> </div> ); }; export default BatteryStatus;
This `BatteryStatus` component will now display the current battery status and dynamically update as the status changes.
Conclusion
The Battery Status API is a powerful tool for building modern, efficient web applications. Coupling it with the flexibility of React Hooks allows us to build a better user experience by intelligently adjusting application behavior based on the host device’s battery status.
The `useBattery` hook showcased in this article is a basic implementation and serves as a foundation. Depending on your application needs, you could expand this hook to include more nuanced functionality, like alerting users when the battery level is critically low or changing app features to save battery when the device is not charging.
Remember, with great power comes great responsibility. While the Battery Status API allows us to enhance our web applications, it’s also essential to respect user privacy and not misuse the information available through this API.
By leveraging the `useBattery` hook, you’re on your way to creating more interactive and dynamic web applications with ReactJS. Keep coding and experimenting, the world of ReactJS hooks is your playground!
Table of Contents
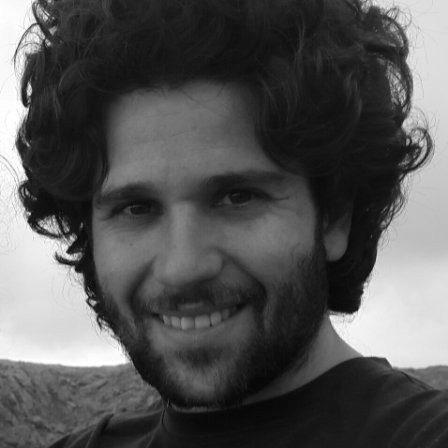
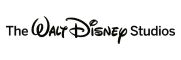