Perfecting Performance: Debugging with ReactJS’s useWhyDidYouUpdate Hook to Eliminate Unwanted Component Rerenders
In ReactJS, one of the trickiest aspects to deal with is component rerendering, especially when it’s unnecessary and affects the application’s performance negatively. Among the tools available to help debug such issues is a custom hook called `useWhyDidYouUpdate`.
In this blog post, we will delve into how to leverage `useWhyDidYouUpdate` to debug unnecessary rerenders in your ReactJS applications and improve their performance.
What is Unnecessary Rerendering?
ReactJS’s one-way data flow makes it relatively simple to track where changes occur in your application. However, sometimes components update even when there’s no apparent reason for them to do so. This is what we call unnecessary rerendering.
Unnecessary rerenders can be problematic because they can result in sluggish interfaces and wasted computational resources, particularly for larger scale applications. And these issues often go unnoticed during development, making them all the more troublesome.
Introducing useWhyDidYouUpdate
`useWhyDidYouUpdate` is a custom hook that helps to pinpoint unnecessary rerenders. It logs into the console where and why the unwanted rerenders occur, giving you a hint of what might be triggering them.
To use `useWhyDidYouUpdate`, you will need to wrap the component with the hook, and it will monitor and log any unnecessary rerenders.
How to use useWhyDidYouUpdate
Before we start, it’s essential to note that `useWhyDidYouUpdate` is meant for development and debugging purposes only. You should never include it in your production build.
Here’s a basic implementation of the `useWhyDidYouUpdate` hook:
import { useRef, useEffect } from 'react'; function useWhyDidYouUpdate(name, props) { const previousProps = useRef(); useEffect(() => { if (previousProps.current) { const allKeys = Object.keys({ ...previousProps.current, ...props }); const changesObj = {}; allKeys.forEach((key) => { if (previousProps.current[key] !== props[key]) { changesObj[key] = { from: previousProps.current[key], to: props[key] }; } }); if (Object.keys(changesObj).length) { console.log('[why-did-you-update]', name, changesObj); } } previousProps.current = props; }); }
This custom hook takes two arguments: the name of the component and the component’s props. It keeps track of the previous props and the current props, comparing them to see if any changes have occurred. If changes are detected, it logs them into the console.
To use this hook in a component, simply call it at the top of your functional component, like so:
const MyComponent = props => { useWhyDidYouUpdate('MyComponent', props); // component logic };
Understanding the Output
Once you’ve implemented `useWhyDidYouUpdate`, it will start to log any prop changes that cause your component to update. These will be logged in the format: `[why-did-you-update] componentName, changesObject`.
The `changesObject` will consist of the changed props, with the old and new values for each prop.
For instance, if a `count` prop changed from 1 to 2, you would see: `{ count: { from: 1, to: 2 } }`.
This information allows you to pinpoint the prop changes that caused an unnecessary rerender, helping you debug and optimize your components more effectively.
Taking it to the Next Level
While `useWhyDidYouUpdate` provides useful insights,
you can enhance its functionality even further. For instance, you can extend it to ignore certain props that you don’t care about, or to only trigger when certain props change. You could even combine it with other tools like `React.memo()` and `useCallback` to prevent unnecessary rerenders in the first place.
Remember, performance optimization is not just about reducing rerenders. It’s also about ensuring that when rerenders do happen, they’re as efficient as possible. The `useWhyDidYouUpdate` hook is a valuable tool in this process.
Conclusion
`useWhyDidYouUpdate` is a potent tool for debugging and optimizing ReactJS applications. By providing insights into unnecessary rerenders, it allows developers to better understand their components and identify potential performance bottlenecks.
Remember, unnecessary rerenders can significantly impact your application’s performance. Therefore, understanding and addressing them is crucial for a smoother and more responsive user experience. But also, keep in mind that premature optimization can be a pitfall, and not every rerender is a problem.
So, before you start optimizing, make sure you have a problem to solve. And once you identify the issues, tools like `useWhyDidYouUpdate` can be of great help.
Use it wisely, improve your app’s performance, and happy coding!
Table of Contents
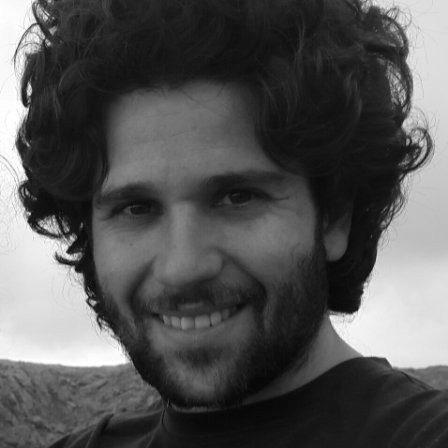
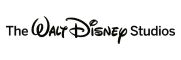