How to access command-line arguments in Ruby?
In Ruby, you can access command-line arguments passed to your script using the built-in `ARGV` array. This array contains all the arguments provided to your Ruby program when it is executed. Here’s how you can access and work with command-line arguments in Ruby:
- Accessing Command-Line Arguments:
The `ARGV` array holds the command-line arguments as strings. You can access individual arguments by their index in the array, starting from 0.
```ruby # Access the first command-line argument first_arg = ARGV[0] # Access the second command-line argument second_arg = ARGV[1] ```
For example, if you run your Ruby script with `ruby my_script.rb arg1 arg2`, `ARGV[0]` will contain `”arg1″` and `ARGV[1]` will contain `”arg2″`.
- Iterating Over Command-Line Arguments:
To process all the command-line arguments, you can use a loop or an iterator like `each`.
```ruby ARGV.each do |arg| puts "Argument: #{arg}" end ```
This code will print each command-line argument one by one.
- Parsing Command-Line Options:
For more complex command-line argument handling, you may want to use a dedicated gem like `OptionParser` to parse and validate options and arguments.
```ruby require 'optparse' options = {} OptionParser.new do |opts| opts.banner = "Usage: my_script.rb [options]" opts.on("-f", "--file FILE", "Specify a file") do |file| options[:file] = file end # Add more options here end.parse! puts "File option: #{options[:file]}" ```
The `OptionParser` allows you to define options with specific flags and descriptions, making it easier to handle various arguments and options gracefully.
Accessing command-line arguments in Ruby is straightforward using the `ARGV` array. It provides a way to interact with and process input provided by users when running your Ruby scripts, making your programs more versatile and adaptable to different scenarios.
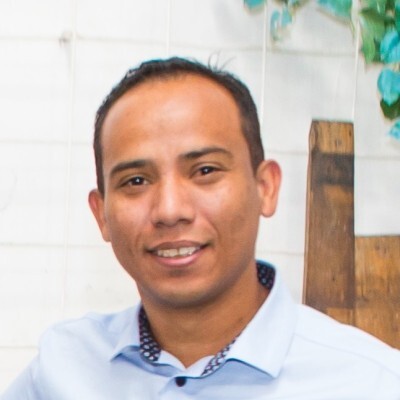
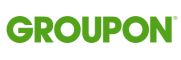