How do I access instance variables in Ruby?
In Ruby, instance variables are used to store and manage data within objects created from a class. To access instance variables, you typically define getter methods within the class. Getter methods allow you to retrieve the values of instance variables from outside the object. Here’s how you can access instance variables in Ruby:
- Define Getter Methods:
To access an instance variable, you define a getter method for that variable within the class. The getter method’s name usually matches the instance variable name, and it returns the value of the instance variable.
```ruby class Person def initialize(name, age) @name = name @age = age end def name @name end def age @age end end ```
In this example, we’ve defined two getter methods, `name` and `age`, to access the `@name` and `@age` instance variables, respectively.
- Accessing Instance Variables:
Once you’ve defined getter methods, you can access instance variables by calling these methods on objects created from the class.
```ruby person = Person.new("Alice", 30) puts person.name # Outputs: "Alice" puts person.age # Outputs: 30 ```
In this code, we create a `Person` object named `person`, and then we use the `name` and `age` getter methods to access the corresponding instance variables.
- Direct Access (Not Recommended):
While it is possible to access instance variables directly from outside the class, it is generally discouraged in Ruby. Accessing instance variables directly can break encapsulation and make your code less maintainable. Using getter methods provides a more controlled and encapsulated way to access object properties.
```ruby person = Person.new("Alice", 30) puts person.instance_variable_get("@name") # This is possible but not recommended ```
Directly accessing instance variables with `instance_variable_get` should be avoided in most cases to maintain good object-oriented programming practices.
Accessing instance variables in Ruby is typically done through getter methods defined within the class. This approach promotes encapsulation and abstraction, making your code more maintainable and following best practices in object-oriented programming.
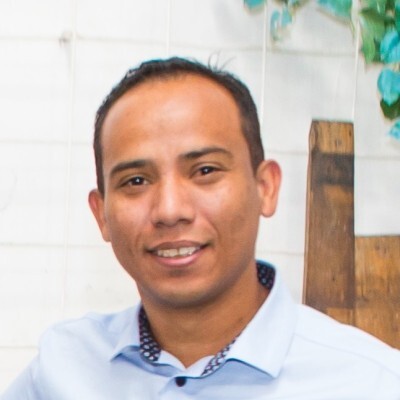
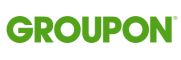