Ruby Q & A
How to add elements to an array in Ruby?
In Ruby, you can add elements to an array using several methods, depending on your specific needs. Here are some common approaches to adding elements to an array:
- Using the `<<` (Shovel) Operator: The `<<` operator is the most common and straightforward way to add an element to the end of an array. It appends the new element to the existing array, increasing its size by one.
```ruby my_array = [1, 2, 3] my_array << 4 # Adds 4 to the end of the array ```
- Using the `push` Method: The `push` method also adds one or more elements to the end of an array. You can pass multiple values as arguments to add them all at once.
```ruby my_array = [1, 2, 3] my_array.push(4) # Adds 4 to the end of the array ```
- Using the `concat` Method: The `concat` method combines two arrays, adding the elements from one array to the end of another. This can be used to add multiple elements from one array to another.
```ruby array1 = [1, 2] array2 = [3, 4] array1.concat(array2) # Adds elements from array2 to the end of array1 ```
- Using the `insert` Method: The `insert` method allows you to add an element at a specific index within the array. You provide the index where you want to insert the element and the value to be inserted.
```ruby my_array = [1, 2, 4] my_array.insert(2, 3) # Inserts 3 at index 2, shifting subsequent elements to the right ```
- Using the `unshift` Method: The `unshift` method adds elements to the beginning of an array, shifting existing elements to the right. You can pass one or more values as arguments.
```ruby my_array = [2, 3, 4] my_array.unshift(1) # Adds 1 to the beginning of the array ```
These methods provide flexibility in adding elements to an array, whether you want to append to the end, insert at a specific position, or prepend to the beginning. Choose the method that best suits your specific use case and desired behavior.
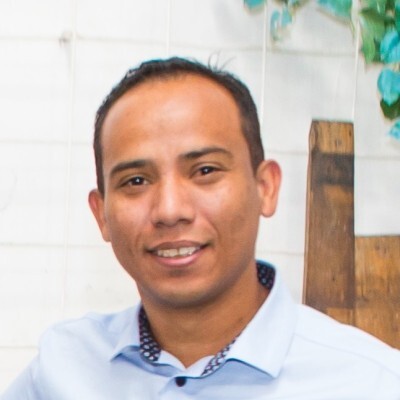
Previously at
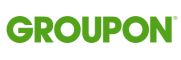
Experienced software professional with a strong focus on Ruby. Over 10 years in software development, including B2B SaaS platforms and geolocation-based apps.