What is the ‘ARGV’ array in Ruby?
In Ruby, the `ARGV` array is a built-in global variable that serves as a container for command-line arguments passed to a Ruby script when it is executed from the command line. It allows Ruby scripts to interact with and process arguments provided by users at runtime.
Here’s what you need to know about the `ARGV` array:
- Storage of Command-Line Arguments:
The `ARGV` array stores command-line arguments as strings. When you run a Ruby script from the command line and provide arguments, those arguments are automatically stored in the `ARGV` array, with each argument occupying a separate element in the array.
For example, if you run your script with `ruby my_script.rb arg1 arg2`, the `ARGV` array will contain `[“arg1”, “arg2”]`.
- Accessing Individual Arguments:
You can access specific command-line arguments by indexing into the `ARGV` array. The array is zero-indexed, so `ARGV[0]` represents the first argument, `ARGV[1]` represents the second argument, and so on.
```ruby first_arg = ARGV[0] # Accesses the first command-line argument second_arg = ARGV[1] # Accesses the second command-line argument ```
- Iterating Over All Arguments:
To process all the command-line arguments, you can use iterators like `each`.
```ruby ARGV.each do |arg| puts "Argument: #{arg}" end ```
This loop will iterate through each argument in the `ARGV` array, allowing you to perform actions or validations on each argument.
- Usage in Ruby Scripts:
The `ARGV` array is commonly used in Ruby scripts to make scripts more flexible and customizable. It enables users to provide input or options when running scripts from the command line. This is particularly useful for creating scripts that can handle different scenarios or configurations based on user-provided arguments.
The `ARGV` array in Ruby is a convenient way to access and work with command-line arguments, making Ruby scripts more versatile and adaptable to user input. It provides a means for scripts to accept input from users and respond accordingly, enhancing the usability and functionality of Ruby programs.
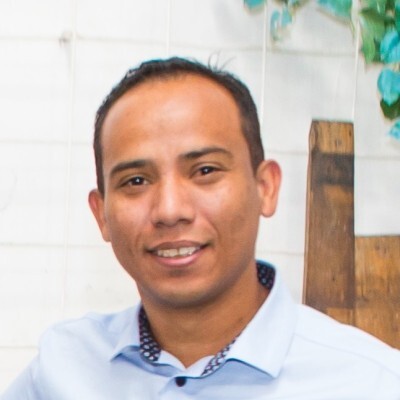
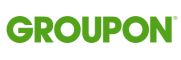