What is the difference between an array and a hash in Ruby?
In Ruby, both arrays and hashes are data structures used to store collections of data, but they have distinct characteristics and use cases.
- Array:
– Ordered Collection: An array is an ordered collection of elements. It maintains the order in which elements are added and allows access to elements by their index, starting from 0.
– Element Access: You can retrieve elements from an array using their index, e.g., `my_array[0]` would access the first element.
– Data Types: Arrays can contain elements of different data types, including numbers, strings, objects, or even other arrays.
– Iteration: Arrays are often used for tasks like looping through a set of items, as they provide methods for iteration such as `each` or `map`.
- Hash:
– Key-Value Pairs: A hash is an unordered collection of key-value pairs. Each element in a hash consists of a unique key and an associated value.
– Element Access: You can access values in a hash by their corresponding keys, not by index. Keys are typically unique identifiers.
– Data Types: While values in a hash can be of any data type, keys are usually symbols or strings. The keys must be unique within a hash.
– Use Cases: Hashes are commonly used for storing data with named attributes, such as representing entities like users or products with associated properties.
Here’s a visual representation of the difference:
Array:
``` [ "apple", "banana", "cherry" ] 0 1 2 ```
Hash:
``` { "name" => "Alice", "age" => 30, "city" => "New York" } key value key value key value ```
Arrays are used for ordered collections where elements are accessed by index, while hashes are used for storing data with named attributes, allowing access by keys. The choice between arrays and hashes depends on your specific data structure needs in a Ruby program.
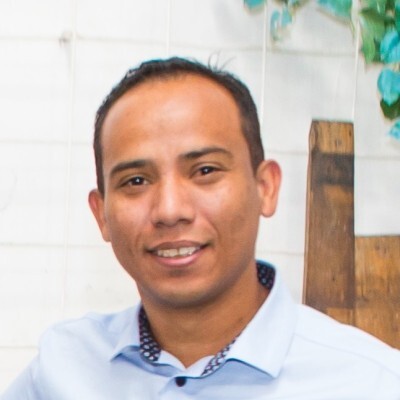
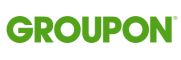