Creating Augmented Reality Apps with Ruby: Integrating AR Technologies
Augmented Reality (AR) has rapidly transformed from a futuristic concept into a practical technology that enhances our digital experiences. It overlays digital information onto the real world, opening up a multitude of opportunities across various industries, from gaming to education and beyond. If you’re an enthusiastic Ruby developer looking to dive into the exciting world of AR, you’re in the right place. In this guide, we’ll explore the process of creating Augmented Reality apps with Ruby and integrating AR technologies to bring your ideas to life.
Table of Contents
1. Understanding Augmented Reality and Its Applications
Before we delve into the technical aspects, let’s establish a solid understanding of Augmented Reality and its applications. AR technology enhances real-world environments by adding computer-generated information, such as images, sounds, or 3D models, to the user’s view. This can be achieved through devices like smartphones, tablets, smart glasses, or AR headsets.
1.1. Applications of Augmented Reality
- Gaming: AR has revolutionized gaming by blending virtual elements with the real world. Games like Pokémon GO have gained massive popularity, allowing players to catch virtual creatures in real-world locations.
- Education: AR enhances learning experiences by making educational content more interactive and engaging. Students can explore historical sites, view 3D models of complex structures, and more.
- Retail: Retailers use AR to enable customers to visualize products in their real-world environment before making a purchase. This technology helps bridge the gap between online and in-store shopping.
- Healthcare: Medical professionals use AR for surgical planning, training, and visualization of complex medical data. This technology aids in improving patient outcomes and safety.
2. Getting Started with Augmented Reality Development in Ruby
To begin creating Augmented Reality apps with Ruby, you’ll need a solid development environment and the right tools. Here’s a step-by-step guide to help you set up your development environment:
Step 1: Choose an AR Framework
Selecting the right AR framework is crucial to your development process. One popular choice is ARKit for iOS development, which provides tools for building AR experiences on Apple devices. For Android development, ARCore offers similar capabilities.
ruby # Gemfile # For ARKit gem 'arkit', '~> 0.4.0' # For ARCore gem 'arcore', '~> 0.3.0'
Step 2: Set Up Your Development Environment
Ensure you have Ruby installed on your system. You can use a version manager like rbenv or rvm for better control over Ruby versions. Next, install the required gems using Bundler:
bash $ bundle install
Step 3: Create Your AR Project
Use your chosen AR framework to create a new project. This might involve setting up a new Xcode project for iOS development or an Android Studio project for Android development.
3. Integrating AR Technologies into Your Ruby App
Now that you have your development environment set up and your project in place, it’s time to integrate AR technologies into your Ruby app. In this section, we’ll explore the key steps involved.
Step 1: Set Up AR Views
In your project, create views or view controllers dedicated to AR experiences. These views will be responsible for rendering the AR content and handling user interactions.
ruby # app/controllers/ar_controller.rb class ARController < ApplicationController def show # Initialize AR session @ar_session = ARSession.new end end
Step 2: Implement AR Interactions
Augmented Reality is all about user engagement. Implement interactions that allow users to interact with AR elements using gestures or touch.
ruby # app/controllers/ar_controller.rb class ARController < ApplicationController def handle_tap # Handle tap gesture on AR object @ar_object.rotate(30.degrees) end end
Step 3: Overlay Digital Content
Use your AR framework’s capabilities to overlay digital content onto the real world. This could include displaying 3D models, images, or textual information.
ruby # app/controllers/ar_controller.rb class ARController < ApplicationController def overlay_content # Load and display a 3D model @ar_scene.load_model('model.obj') @ar_scene.display_text('Welcome to the AR experience!', position: [0, 0, -1]) end end
Step 4: Handle AR Tracking
AR frameworks provide tracking functionality to anchor digital content to the real world accurately. Implement tracking to ensure AR objects remain in their intended positions.
ruby # app/controllers/ar_controller.rb class ARController < ApplicationController def track_object # Track and anchor AR object to a real-world feature @ar_object.track_with(feature: 'image_marker') end end
4. Enhancing Your AR App with Advanced Features
As you become more comfortable with integrating AR technologies into your Ruby app, you can explore advanced features to create richer AR experiences.
4.1. Spatial Mapping
Implement spatial mapping to understand the physical environment’s geometry. This allows your AR app to interact intelligently with real-world surfaces.
4.2. Gesture Recognition
Incorporate gesture recognition to enable users to control AR objects using hand gestures or motions, adding a layer of interactivity to your app.
4.3. Real-time Collaboration
Explore real-time collaboration features, allowing multiple users to experience the same AR environment simultaneously, opening up possibilities for multiplayer AR experiences.
Conclusion
Augmented Reality is a fascinating technology that holds immense potential for innovation. By integrating AR technologies into your Ruby app, you can create engaging and interactive experiences that bridge the gap between the digital and physical worlds. This guide has provided you with a foundational understanding of creating Augmented Reality apps with Ruby. As you continue your AR journey, don’t hesitate to explore more advanced features and push the boundaries of what’s possible in the realm of AR development. Get ready to bring your imaginative ideas to life through the exciting fusion of Ruby and Augmented Reality!
Table of Contents
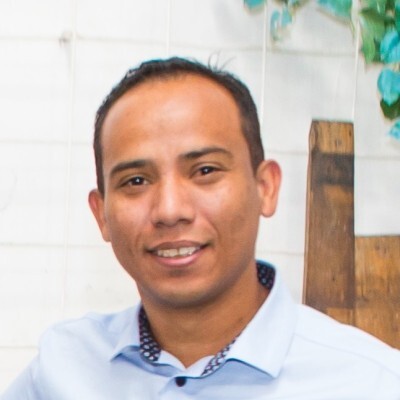
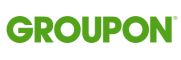