Secure Authentication and Authorization in Ruby Applications
In an increasingly interconnected digital world, ensuring the security of user data and system resources is of paramount importance. One of the foundational aspects of application security is implementing robust authentication and authorization mechanisms. Ruby, a versatile and dynamic programming language, offers a range of tools and libraries that empower developers to create secure applications. In this article, we’ll dive into the world of secure authentication and authorization in Ruby applications, exploring best practices, techniques, and code samples that can help you safeguard your users’ data and your application’s integrity.
Table of Contents
1. Understanding Authentication and Authorization:
1.1. Defining Authentication:
Authentication is the process of verifying the identity of a user, ensuring that they are who they claim to be. This typically involves validating their credentials, such as a username and password combination. In a Ruby application, proper authentication prevents unauthorized users from gaining access to sensitive data and functionalities.
1.2. Introducing Authorization:
Authorization, on the other hand, is the process of determining what actions an authenticated user is allowed to perform within the application. It involves enforcing access controls based on the user’s role, permissions, or other attributes. Authorization ensures that even authenticated users can only perform actions that are appropriate for their level of access.
2. The Importance of Secure Authentication:
2.1. Common Authentication Vulnerabilities:
Implementing weak or faulty authentication mechanisms can expose your application to a range of security vulnerabilities, including password brute-forcing, credential stuffing, and session hijacking. Attackers can exploit these vulnerabilities to gain unauthorized access to user accounts and sensitive data.
2.2. Mitigating Risks with Ruby:
Ruby provides developers with a suite of tools and libraries that can help mitigate these risks. By implementing secure authentication mechanisms, you can significantly reduce the chances of unauthorized access. Let’s delve into some best practices for implementing secure authentication in Ruby applications.
3. Implementing Authentication in Ruby Applications:
3.1. Hashing Passwords:
Storing plain text passwords in your database is a significant security risk. Instead, use cryptographic hashing algorithms like BCrypt to hash passwords before storing them. Here’s a simple example using the BCrypt gem:
ruby require 'bcrypt' password = "secure_password" hashed_password = BCrypt::Password.create(password)
3.2. Using Salt for Extra Security:
Salting involves adding a unique value to each password before hashing. This prevents attackers from using precomputed rainbow tables for cracking hashed passwords. BCrypt automatically handles salting, making it an excellent choice for password hashing in Ruby applications.
4. Authorization: Controlling Access in Ruby:
4.1. Role-Based Authorization:
Implementing role-based authorization allows you to assign specific roles to users and define what actions each role can perform. For instance, you might have roles like “user,” “admin,” and “moderator.” The CanCanCan gem simplifies role-based authorization by providing a DSL for defining authorization rules.
ruby # Define abilities in an Ability class class Ability include CanCan::Ability def initialize(user) user ||= User.new # guest user if user.admin? can :manage, :all else can :read, Article end end end
4.2. Attribute-Based Authorization:
Sometimes, access control depends on attributes associated with the user or resource. The Pundit gem allows you to define policies that grant or deny access based on specific attributes.
ruby # Define policies for Article model class ArticlePolicy < ApplicationPolicy def update? user.admin? || record.author == user end end
5. Leveraging Gems for Enhanced Security:
5.1. Devise: A Comprehensive Authentication Solution:
The Devise gem is a popular choice for adding authentication to Ruby applications. It provides ready-to-use controllers, views, and models for user management. Devise also supports features like password reset, account locking, and session management out of the box.
5.2. Pundit: Flexible Authorization Policies:
The Pundit gem complements Devise by offering a flexible authorization framework. It encourages you to define granular authorization policies using plain Ruby classes. This separation of concerns between authentication (Devise) and authorization (Pundit) enhances code clarity and maintainability.
6. Multi-Factor Authentication: Adding an Extra Layer of Security:
Multi-factor authentication (MFA) adds an extra layer of security by requiring users to provide multiple forms of verification before accessing their accounts. Implementing MFA in your Ruby application can significantly enhance its security posture. Libraries like “rotp” (Ruby One-Time Password) can help you integrate time-based one-time password (TOTP) or HOTP (HMAC-based One-Time Password) authentication.
7. Best Practices for Secure Authentication and Authorization:
7.1. Regularly Update Dependencies:
Keep your Ruby gems and dependencies up to date to ensure you’re protected against known security vulnerabilities.
7.2. Implementing Strong Password Policies:
Enforce strong password policies, including minimum length, complexity requirements, and regular password changes. This reduces the likelihood of successful brute-force attacks.
7.3. Logging and Monitoring for Suspicious Activity:
Implement logging and monitoring mechanisms to detect and respond to suspicious authentication and authorization attempts. Regularly review logs to identify potential security breaches.
Conclusion
In an era where data breaches and security threats are on the rise, securing your Ruby applications with robust authentication and authorization mechanisms is non-negotiable. By implementing best practices, leveraging gems, and staying informed about emerging security trends, you can fortify your application’s defenses and provide a safe environment for your users’ sensitive data. Remember that security is an ongoing process, so continuously monitor and update your authentication and authorization strategies to stay one step ahead of potential threats.
Table of Contents
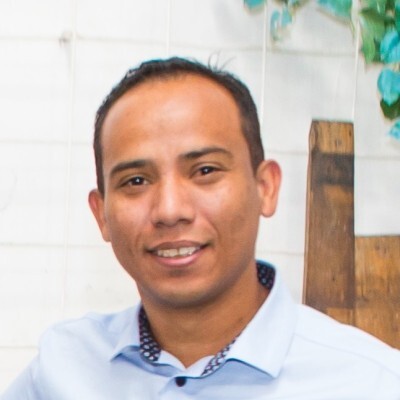
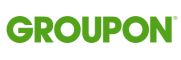