Automating Web Testing with Ruby: Tools and Best Practices
In today’s fast-paced development environment, ensuring the quality and functionality of web applications is of paramount importance. Manual testing can be time-consuming, error-prone, and inadequate for thoroughly testing complex web applications. This is where automated web testing comes into play, and Ruby has emerged as a popular choice for writing efficient and effective test scripts. In this blog post, we’ll dive into the world of automating web testing using Ruby, exploring various tools, best practices, and providing code samples to help you get started.
Table of Contents
1. Why Automate Web Testing?
Automated web testing offers a range of benefits that contribute to the overall success of your software development lifecycle. Some key advantages include:
- Time Efficiency: Manual testing can be time-consuming, especially when testing across different browsers and devices. Automated tests can be executed much faster, saving valuable time during the development process.
- Consistency: Automated tests ensure consistent test execution, reducing the risk of human errors and ensuring that the same tests are executed the same way every time.
- Reusability: Test scripts can be reused across different projects, reducing the effort required to create new tests from scratch.
- Scalability: As your application grows, the number of test cases also increases. Automating tests makes it easier to scale your testing efforts without exponentially increasing the testing time.
- Early Detection of Bugs: Automated tests can be integrated into the continuous integration/continuous deployment (CI/CD) pipeline, allowing for the early detection of bugs and issues as code is being developed.
2. Popular Ruby Tools for Web Testing
Several powerful tools and frameworks are available in the Ruby ecosystem to assist you in automating web testing. Let’s take a look at some of the most popular ones:
2.1. Capybara
Capybara is a versatile and user-friendly web testing framework that provides a simple DSL (Domain-Specific Language) for interacting with web pages. It supports various drivers for different browsers and allows you to write expressive and readable tests.
Installation:
ruby gem install capybara
Example Usage:
ruby require 'capybara' require 'capybara/dsl' Capybara.run_server = false Capybara.default_driver = :selenium_chrome # You can choose different drivers class MyTest include Capybara::DSL def run_test visit 'https://www.example.com' fill_in 'username', with: 'user123' fill_in 'password', with: 'pass456' click_button 'Login' expect(page).to have_content 'Welcome, User!' end end test = MyTest.new test.run_test
2.2. RSpec
RSpec is a popular testing framework for Ruby that provides a clean and readable syntax for writing tests. It’s often used in conjunction with Capybara to create powerful automated web tests.
Installation:
ruby gem install rspec
Example Usage:
ruby require 'rspec' require 'capybara/rspec' RSpec.configure do |config| config.include Capybara::DSL end describe 'Web Application' do it 'should display the homepage' do visit 'https://www.example.com' expect(page).to have_content 'Welcome to our website' end it 'should allow users to log in' do visit 'https://www.example.com/login' fill_in 'username', with: 'user123' fill_in 'password', with: 'pass456' click_button 'Login' expect(page).to have_content 'Welcome, User!' end end
2.3. Watir
Watir is another powerful web automation framework that allows you to control browsers using a friendly and intuitive API. It supports multiple browsers and provides a wide range of actions you can perform on web elements.
Installation:
ruby gem install watir
Example Usage:
ruby require 'watir' browser = Watir::Browser.new :chrome # You can choose different browsers browser.goto 'https://www.example.com' browser.text_field(id: 'username').set 'user123' browser.text_field(id: 'password').set 'pass456' browser.button(type: 'submit').click puts browser.title
3. Best Practices for Automated Web Testing with Ruby
While using the right tools is crucial, following best practices ensures that your automated web testing efforts are efficient, maintainable, and effective.
3.1. Use Page Objects
Page Objects are a design pattern that helps separate the test code from the structure of the web application. They provide a clear and maintainable way to interact with web pages, reducing duplication and improving readability.
ruby # Example Page Object class LoginPage include Capybara::DSL def visit visit '/login' end def fill_credentials(username, password) fill_in 'username', with: username fill_in 'password', with: password end def submit click_button 'Login' end end
3.2. Data-Driven Testing
Use different sets of test data to validate the behavior of your application under various scenarios. This helps uncover potential issues that might not be apparent with just a single set of data.
ruby # Example Data-Driven Test RSpec.describe 'Login functionality' do it 'should allow valid users to log in' do valid_users = [ { username: 'user123', password: 'pass456' }, { username: 'anotheruser', password: 'anotherpass' } ] valid_users.each do |user| login_page.visit login_page.fill_credentials(user[:username], user[:password]) login_page.submit expect(page).to have_content 'Welcome, User!' end end end
3.3. Maintain Test Suites
Organize your tests into logical test suites to make it easier to manage and run specific sets of tests. This is particularly important as your test suite grows over time.
3.4. Continuous Integration
Integrate your automated tests into your CI/CD pipeline to ensure that tests are run automatically whenever new code is pushed to the repository. This helps catch bugs early and maintains the overall quality of your application.
3.5. Regular Maintenance
Web applications evolve over time, and so should your automated tests. Regularly review and update your test scripts to ensure they remain relevant and accurate.
Conclusion
Automating web testing with Ruby offers a powerful way to ensure the quality and reliability of your web applications. By leveraging tools like Capybara, RSpec, and Watir, and following best practices such as using Page Objects, data-driven testing, and integrating with CI/CD pipelines, you can streamline your testing process and catch bugs before they reach your users. Embrace the world of automated web testing with Ruby, and watch your development process become more efficient and your applications more robust.
Table of Contents
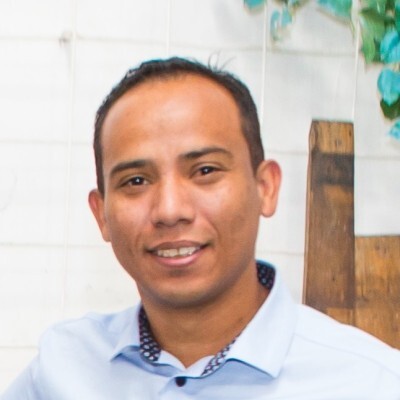
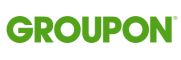