How to benchmark code performance in Ruby?
In Ruby, benchmarking code performance is essential to identify bottlenecks, optimize critical sections, and ensure that your applications run efficiently. There are several ways to benchmark code performance in Ruby, and one of the most commonly used methods is utilizing the `Benchmark` module, which is part of Ruby’s standard library.
Here’s a step-by-step guide on how to benchmark code performance in Ruby using the `Benchmark` module:
- Require the Benchmark Module:
First, you need to require the `Benchmark` module in your Ruby script or program:
```ruby require 'benchmark' ```
- Wrap the Code to Be Benchmarked:
Wrap the code you want to benchmark inside the `Benchmark.measure` block. This block will measure the execution time of the code
```ruby require 'benchmark' time = Benchmark.measure do # Code to be benchmarked end ```
- Print the Benchmark Results:
After measuring the code’s execution time, you can access various metrics, such as real time, user CPU time, and system CPU time, from the `time` object and print them:
```ruby puts "Execution Time: #{time.real} seconds" puts "User CPU Time: #{time.utime} seconds" puts "System CPU Time: #{time.stime} seconds" ```
– `time.real` represents the real time elapsed.
– `time.utime` represents the user CPU time.
– `time.stime` represents the system CPU time.
By following these steps, you can effectively benchmark the performance of your Ruby code. This allows you to identify performance bottlenecks and optimize critical sections for better efficiency. Benchmarking is a crucial practice in software development, especially when dealing with large-scale applications or performance-sensitive code, as it helps ensure that your Ruby programs run efficiently and meet performance requirements.
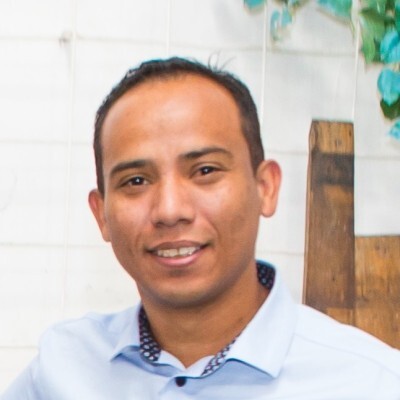
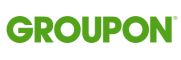