Building GraphQL APIs with Ruby: Leveraging Libraries like GraphQL-Ruby
In the world of modern web development, APIs are the backbone of applications, allowing them to communicate and exchange data seamlessly. GraphQL has emerged as a powerful alternative to traditional REST APIs, offering more flexibility and efficiency in data fetching. When it comes to building GraphQL APIs with Ruby, the GraphQL-Ruby library stands out as a prominent choice. In this article, we’ll embark on a journey to explore the ins and outs of building GraphQL APIs using GraphQL-Ruby, diving into key concepts, implementation steps, and best practices.
Table of Contents
1. Understanding GraphQL and Its Advantages
1.1. Dealing with Over-fetching and Under-fetching
Traditional REST APIs often suffer from the issues of over-fetching and under-fetching. Over-fetching occurs when an API returns more data than what the client needs, leading to wastage of resources. On the other hand, under-fetching happens when the API doesn’t provide enough information in a single request, forcing the client to make multiple requests. GraphQL addresses these problems by allowing clients to specify exactly what data they need, eliminating unnecessary data transfer.
1.2. Flexible Data Retrieval with GraphQL
GraphQL empowers clients to define the shape and structure of the data they require. This is achieved through queries, where clients request specific fields on specific types. This approach gives clients the ability to gather all the necessary data in a single request, enhancing efficiency. Moreover, GraphQL supports real-time data updates through subscriptions, making it an ideal choice for applications that require real-time interactions.
2. Getting Started with GraphQL-Ruby
2.1. Installation and Setup
To start building GraphQL APIs with Ruby, you’ll need to install the GraphQL-Ruby gem. Open your terminal and execute the following command:
ruby gem install graphql
Once the gem is installed, you can create a new Ruby project or navigate to your existing project’s directory.
2.2. Defining Types and Fields
In GraphQL, data is organized into types, and each type can have fields. Fields are the specific pieces of data that can be requested by clients. Let’s define a simple type and field using GraphQL-Ruby:
ruby class Types::QueryType < Types::BaseObject field :hello, String, null: false, description: "A simple greeting", resolve: ->(_obj, _args, _ctx) { "Hello, GraphQL-Ruby!" } end
In this example, we define a hello field of type String within the QueryType class. The resolve block contains the logic to provide the value for this field when it’s queried.
2.3. Creating the Schema
The schema acts as a contract between the client and the server, defining the types and queries available in your API. Here’s how you can create a schema using GraphQL-Ruby:
ruby class YourAppSchema < GraphQL::Schema query Types::QueryType end
In this code, we associate the YourAppSchema with the QueryType, which contains the initial query fields.
3. Writing Queries and Mutations
3.1. Querying Data
Queries in GraphQL-Ruby are analogous to GET requests in REST. Clients use queries to request specific data from the server. Let’s create a query to fetch the greeting we defined earlier:
ruby query { hello } When this query is executed, it will return the response: json Copy code { "data": { "hello": "Hello, GraphQL-Ruby!" } }
3.2. Mutation for Data Modification
Mutations in GraphQL-Ruby handle data modifications, such as creating, updating, or deleting records. Here’s an example of a simple mutation:
ruby class Types::MutationType < Types::BaseObject field :create_post, Types::PostType, null: true, description: "Create a new post", arguments: { title: String, content: String }, resolve: ->(_obj, args, _ctx) { Post.create(args) } end
In this case, we define a mutation create_post that takes title and content arguments and returns a new post object. The resolve block handles the creation of the post.
4. Advanced Concepts in GraphQL-Ruby
4.1. Input Types for Complex Mutations
For more complex mutations that involve nested or multiple inputs, it’s a good practice to use input types. An input type defines a structured input format for mutations. Here’s an example:
ruby class Types::CreatePostInput < Types::BaseInputObject argument :title, String, required: true argument :content, String, required: true end class Types::MutationType < Types::BaseObject field :create_post, Types::PostType, null: true, description: "Create a new post", argument: :input, Types::CreatePostInput, resolve: ->(_obj, args, _ctx) { Post.create(args[:input].to_h) } end
4.2. Resolvers: Bridging Queries and Data Sources
Resolvers in GraphQL-Ruby are responsible for fetching the actual data for a query or mutation. They bridge the gap between the schema and the data sources. Here’s a simplified example:
ruby class Types::QueryType < Types::BaseObject field :post, Types::PostType, null: true, description: "Find a post by ID", argument: :id, ID, resolve: ->(_obj, args, _ctx) { Post.find(args[:id]) } end
In this case, the resolver fetches a post from the database based on the provided ID.
4.3. Authentication and Authorization
GraphQL-Ruby integrates well with authentication and authorization mechanisms. You can utilize middleware to perform authentication checks before executing queries or mutations. This ensures that only authorized users can access certain parts of your API.
5. Best Practices for Building GraphQL APIs
5.1. Keep Your Schema Simple and Consistent
Maintaining a clear and concise schema is crucial for the long-term success of your API. Avoid unnecessary complexity and follow consistent naming conventions for types, fields, and arguments.
5.2. Versioning and Deprecation
GraphQL-Ruby allows you to version your schema to ensure compatibility as your API evolves. When making breaking changes, it’s a good practice to deprecate old fields or types to provide a smooth transition for clients.
5.3. Caching Strategies for GraphQL
Caching plays a significant role in optimizing GraphQL APIs. Since a single query can request various pieces of data, caching at the field level can be more effective than caching at the query level. Consider using caching libraries like graphql-cache.
6. Testing Your GraphQL API
6.1. Unit Testing for Resolvers
Writing unit tests for your resolvers ensures that they return the expected data. Tools like RSpec can be used for testing GraphQL-Ruby code.
6.2. Integration Testing for Queries and Mutations
Integration tests are essential to validate the behavior of your API as a whole. Tools like graphql-rspec make it easy to write integration tests for your queries and mutations.
Conclusion
With the GraphQL-Ruby library, building robust and efficient GraphQL APIs becomes an exciting endeavor. We’ve covered the basics of getting started, defining types and fields, writing queries and mutations, delving into advanced concepts like resolvers, authentication, and authorization, and discussed best practices and testing strategies.
As you continue your journey with GraphQL-Ruby, remember that practice is key. Experiment with different schema designs, explore the rich ecosystem of GraphQL-Ruby extensions, and stay updated with the latest developments. By leveraging the power of GraphQL and the capabilities of GraphQL-Ruby, you can create APIs that provide exactly the data your clients need, enhancing the performance and user experience of your applications.
Table of Contents
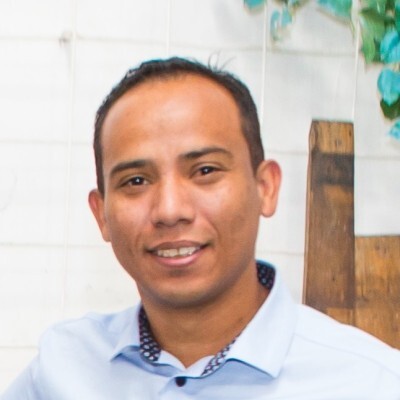
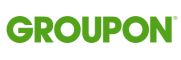