Building Microservices with Ruby: Scalable and Modular Architecture
In the modern era of software development, where agility, scalability, and maintainability are paramount, microservices architecture has emerged as a compelling solution. Microservices promote the decomposition of large, monolithic applications into smaller, independently deployable services. This architectural approach brings numerous benefits, such as improved scalability, fault isolation, and easier maintenance. In this blog, we’ll dive into the world of microservices with a focus on using Ruby, a dynamic and versatile programming language, to build scalable and modular microservices.
Table of Contents
1. Understanding Microservices Architecture
1.1. What are Microservices?
Microservices architecture is a software development approach where an application is decomposed into smaller, self-contained services. Each service represents a specific business capability and can be developed, deployed, and scaled independently. These services communicate over well-defined APIs, often using lightweight protocols like HTTP or message queues. This decoupled nature enables teams to work independently on different services, leading to faster development cycles and easier maintenance.
1.2. Benefits of Microservices Architecture
The adoption of microservices offers several advantages:
- Scalability: Individual microservices can be scaled horizontally to handle varying loads, ensuring efficient resource utilization.
- Fault Isolation: If a single microservice fails, it doesn’t bring down the entire application, as other services can continue functioning.
- Technology Diversity: Different services can be developed using different technologies, allowing teams to choose the best tools for specific tasks.
- Continuous Deployment: Microservices can be deployed independently, enabling faster release cycles and reduced risk during updates.
- Modularity: Services can be developed and maintained independently, making it easier to understand, test, and debug code.
- Improved Maintenance: Changes and updates can be isolated to specific services, reducing the risk of unintended consequences.
2. Why Ruby for Microservices?
2.1. Ruby’s Simplicity and Productivity
Ruby’s clean and elegant syntax, inspired by natural language, makes it a joy to work with. This simplicity leads to increased developer productivity, as code is easy to write, read, and maintain. When building microservices, where the focus is on rapid development and iteration, Ruby’s expressiveness can significantly speed up the process.
2.2. Rich Ecosystem and Libraries
Ruby boasts a vibrant ecosystem with a wide range of libraries and gems that can streamline microservices development. Frameworks like Sinatra and Ruby on Rails provide scaffolding for building web services, while tools like Sidekiq simplify asynchronous processing. Leveraging these resources, developers can focus on business logic rather than reinventing the wheel.
2.3. Metaprogramming Capabilities
Ruby’s powerful metaprogramming capabilities enable developers to write flexible and dynamic code. This is especially valuable in microservices architecture, where services often need to adapt to changing requirements and interfaces. Metaprogramming allows for more dynamic service discovery, data transformation, and protocol adaptation.
3. Designing Scalable Microservices with Ruby
3.1. Service Boundaries and Responsibilities
In microservices architecture, defining clear service boundaries and responsibilities is crucial. Each microservice should have a well-defined purpose and perform a specific business function. This ensures that services remain focused, making them easier to develop, test, and maintain. Using Ruby’s object-oriented programming paradigm, services can be encapsulated as classes, promoting modular and maintainable code.
3.2. Communication Between Services
Microservices interact through APIs, and communication between services can be synchronous or asynchronous. For synchronous communication, HTTP APIs are commonly used, and Ruby’s Sinatra framework provides an excellent foundation for building lightweight and RESTful APIs. Asynchronous communication, on the other hand, can be achieved using message queues like RabbitMQ or Kafka, combined with Ruby libraries such as Bunny or Poseidon.
3.3. Data Management and Databases
Each microservice typically manages its own database, ensuring data isolation and autonomy. Ruby’s ActiveRecord, a popular Object-Relational Mapping (ORM) library, simplifies database interactions. With ActiveRecord, developers can model data as objects and perform database operations using Ruby code, reducing the complexity of SQL queries.
4. Building Modular Microservices
4.1. Separation of Concerns
Modular microservices follow the principle of separation of concerns, where different parts of an application are isolated based on their functionality. In Ruby, this can be achieved through well-defined classes and modules. Each microservice should encapsulate its logic, minimizing dependencies on other services.
4.2. Dependency Management
Ruby’s package manager, Bundler, facilitates dependency management by allowing developers to specify required gems and libraries. This ensures that each microservice’s dependencies are well-defined and isolated, preventing conflicts between different services.
4.3. Shared Libraries and Gems
To further promote modularity, consider creating shared libraries or gems containing common functionality, such as authentication, logging, or error handling. These gems can be reused across multiple microservices, reducing duplication and maintaining consistency.
5. Implementing Microservices with Ruby
5.1. Service Creation and Structure
When creating a microservice with Ruby, start by structuring the service as a separate project. This can be a directory containing all the necessary files and folders. Each microservice can have its own Gemfile to manage dependencies.
5.2. RESTful APIs with Sinatra
Sinatra is a lightweight web framework for building APIs. It simplifies routing, request handling, and response generation. Here’s a basic example of creating a RESTful API endpoint for a microservice using Sinatra:
ruby require 'sinatra' get '/api/resource/:id' do # Fetch and return the resource with the specified ID end post '/api/resource' do # Create a new resource based on the request data end put '/api/resource/:id' do # Update the resource with the specified ID end delete '/api/resource/:id' do # Delete the resource with the specified ID end
5.3. Asynchronous Processing with Sidekiq
For background jobs and asynchronous processing, Sidekiq is a popular choice in the Ruby ecosystem. It allows you to perform tasks outside the scope of a user request, enhancing application responsiveness. Here’s a simple example of using Sidekiq to process a task asynchronously:
ruby class MyWorker include Sidekiq::Worker def perform(arg1, arg2) # Perform the asynchronous task with arg1 and arg2 end end # Enqueue the task MyWorker.perform_async(value1, value2)
6. Ensuring Robustness and Resilience
6.1. Error Handling and Fault Tolerance
In a microservices architecture, services should be designed to handle errors gracefully. Ruby’s exception handling mechanisms, such as begin-rescue blocks, can be used to capture and handle errors effectively. Implementing retries and fallback mechanisms can improve fault tolerance.
6.2. Load Balancing and Redundancy
To ensure high availability, microservices can be deployed across multiple instances or servers. Load balancers distribute incoming traffic across these instances, preventing any single point of failure. Ruby can work seamlessly in load-balanced environments, provided proper configuration.
6.3. Circuit Breakers and Retries
Implementing circuit breakers can prevent cascading failures by temporarily halting communication with a service experiencing issues. Ruby libraries like “circuit_breaker” can be integrated to automate this functionality. Additionally, retries with exponential backoff can improve the chances of a service recovering from temporary failures.
7. Testing and Deployment Strategies
7.1. Unit Testing and Service Isolation
Each microservice should have comprehensive unit tests to ensure its functionality works as expected. Mocking frameworks like RSpec and Mocha can facilitate isolating the service under test from its dependencies. This ensures that tests remain focused and reliable.
7.2. Continuous Integration and Deployment
Microservices development benefits from continuous integration and deployment (CI/CD) practices. Tools like Jenkins, Travis CI, or CircleCI can automate testing and deployment processes, ensuring that changes are thoroughly tested and deployed consistently.
7.3. Containerization with Docker
Docker provides a convenient way to package microservices and their dependencies into isolated containers. This ensures consistent environments across different stages of development, testing, and production. Ruby-based microservices can be containerized, allowing for easy deployment and scaling.
Conclusion
In conclusion, building microservices with Ruby offers a scalable and modular architecture that aligns well with the principles of agility and maintainability. The combination of Ruby’s simplicity, rich ecosystem, and metaprogramming capabilities empowers developers to create efficient and flexible microservices. By understanding the core concepts of microservices architecture, designing for scalability and modularity, and utilizing Ruby’s strengths, developers can embark on a journey to create robust and highly maintainable applications in the modern software landscape.
Table of Contents
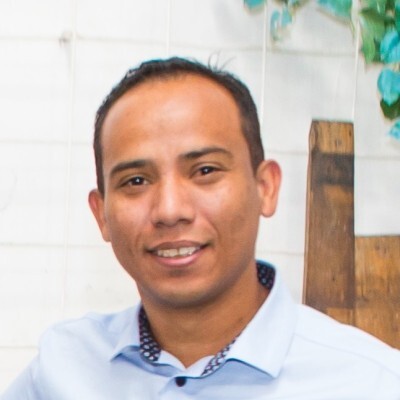
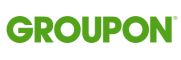