Building Neural Interfaces with Ruby: Brain-Computer Interaction and EEG
In the realm of technology, the intersection of neuroscience and programming has given rise to an exciting field known as Brain-Computer Interaction (BCI). BCI allows us to bridge the gap between the human brain and machines, opening doors to incredible possibilities. In this blog, we will explore the fascinating world of BCI and Electroencephalography (EEG) while delving into how Ruby, a versatile programming language, can be used to create neural interfaces.
Table of Contents
1. Understanding Brain-Computer Interaction (BCI)
1.1. What is BCI?
Brain-Computer Interaction is a groundbreaking technology that enables direct communication between the human brain and computers or other external devices. It holds immense potential in various domains, including healthcare, gaming, accessibility, and more. BCIs work by translating brain activity into actionable signals that machines can interpret and respond to.
1.2. Applications of BCI
BCI technology has the power to revolutionize numerous fields:
- Healthcare: BCI can be used to assist individuals with paralysis, allowing them to control robotic limbs or communicate using their thoughts.
- Gaming: Imagine playing video games with your mind! BCI can enhance the gaming experience by providing an entirely new level of interaction.
- Education: BCI can be used in educational settings to monitor students’ cognitive states and adapt learning materials accordingly.
- Accessibility: BCIs can provide a lifeline for individuals with severe physical disabilities, giving them the ability to control everyday devices and communicate more effectively.
- Research: Neuroscientists use BCIs to gain deeper insights into brain function and disorders, leading to advancements in our understanding of the human brain.
2. Introduction to EEG
2.1. What is EEG?
Electroencephalography (EEG) is a non-invasive technique used to record electrical activity in the brain. It involves placing electrodes on the scalp to measure the voltage fluctuations resulting from ionic current flows within the neurons of the brain. EEG is widely used in neuroscience and BCI research due to its portability and high temporal resolution.
2.2. EEG in BCI
EEG signals are a primary source of input data for many BCI applications. These signals can be used to detect brain patterns associated with specific mental states, such as motor imagery, concentration, or relaxation. By decoding these patterns, BCIs can perform various actions, like controlling a cursor on a computer screen or sending commands to external devices.
3. Building a Simple EEG Data Acquisition System in Ruby
Now that we have a basic understanding of BCI and EEG, let’s dive into building a simple EEG data acquisition system using Ruby. We’ll start with setting up the hardware and then move on to capturing and processing EEG data.
3.1. Prerequisites
Before we begin, make sure you have the following hardware and software:
- EEG Device: You’ll need an EEG headset that can interface with your computer. There are various options available, ranging from consumer-grade devices to research-grade systems.
- Ruby: Install Ruby on your computer if you haven’t already. You can download it from the official Ruby website.
- Ruby Gems: We’ll be using several Ruby gems, including serialport for serial communication and csv for data storage. Install them using gem install serialport csv.
3.2. Setting Up Serial Communication
We’ll establish a serial connection with the EEG device to receive real-time EEG data. Here’s a Ruby script to get you started:
ruby require 'serialport' # Define the serial port settings port = '/dev/ttyUSB0' # Replace with the appropriate port for your device baud_rate = 57600 # Create a SerialPort object serial_port = SerialPort.new(port, baud_rate) # Read and display EEG data loop do data = serial_port.gets.chomp puts "EEG Data: #{data}" end
In this code:
- We require the serialport gem to work with serial communication.
- Replace ‘/dev/ttyUSB0’ with the correct serial port for your EEG device. The baud rate may also vary depending on your device’s specifications.
- We continuously read data from the serial port and display it in the console. EEG data typically consists of voltage values from different electrodes.
- This is a basic setup for receiving EEG data. The format and content of the data may vary depending on your EEG device, so refer to its documentation for specific details.
3.3. Data Processing and Visualization
Once you can successfully receive EEG data, you can enhance your system by processing and visualizing it. Here’s an example of how to store EEG data in a CSV file for further analysis:
ruby require 'serialport' require 'csv' # Define the serial port settings port = '/dev/ttyUSB0' # Replace with the appropriate port for your device baud_rate = 57600 # Create a SerialPort object serial_port = SerialPort.new(port, baud_rate) # Create a CSV file for data storage csv_filename = 'eeg_data.csv' CSV.open(csv_filename, 'w') do |csv| csv << ['Timestamp', 'EEG Data'] # Read and write EEG data loop do data = serial_port.gets.chomp timestamp = Time.now.strftime('%Y-%m-%d %H:%M:%S') # Write data to CSV csv << [timestamp, data] puts "EEG Data: #{data}" end end
In this code:
- We require the csv gem to work with CSV files.
- We create a CSV file named ‘eeg_data.csv’ and define its headers.
- EEG data, along with timestamps, is continuously read from the serial port and written to the CSV file.
- This CSV file can be further analyzed using data science libraries or visualized using tools like matplotlib or gnuplot.
4. Integrating Machine Learning with EEG Data
To harness the true power of EEG data, we can integrate machine learning techniques to recognize patterns and make predictions based on brain activity. Ruby provides various libraries for machine learning, making it a suitable choice for this purpose.
4.1. Prerequisites
Before proceeding, make sure you have the following additional prerequisites:
- Ruby Machine Learning Libraries: Install Ruby machine learning libraries such as scikit-learn, tensorflow, or keras. You can install these libraries using gem install scikit-learn tensorflow keras.
- EEG Data Preprocessing: EEG data often requires preprocessing steps such as filtering, feature extraction, and normalization. Familiarize yourself with these techniques or consult with a domain expert to preprocess your data effectively.
4.2. EEG Data Preprocessing
Let’s start by loading the EEG data we collected in the previous section and performing some basic preprocessing. For this example, we’ll assume that your EEG data is stored in the ‘eeg_data.csv’ file.
ruby require 'csv' require 'scikit-learn' # Load EEG data from the CSV file csv_filename = 'eeg_data.csv' data = [] CSV.foreach(csv_filename, headers: true) do |row| data << [row['Timestamp'], row['EEG Data'].to_f] end # Preprocess EEG data (add your preprocessing steps here) # For example, you can filter the data, extract features, and normalize it.
In this code:
- We load the EEG data from the CSV file into a Ruby array.
- We use the scikit-learn gem for machine learning tasks. You can adapt this code to use other Ruby machine learning libraries if you prefer.
- The preprocessing step is a crucial part of working with EEG data. Depending on your application, you may need to apply filters, extract relevant features, and normalize the data.
4.3. Building a Machine Learning Model
Now that we have preprocessed EEG data, we can build a simple machine learning model for demonstration purposes. We’ll use a classification model as an example.
ruby require 'scikit-learn' # Prepare your preprocessed EEG data (X) and labels (y) # X should be a 2D array with each row representing a sample, and y should be a 1D array of labels. # Split the data into training and testing sets X_train, X_test, y_train, y_test = train_test_split(X, y, test_size: 0.2, random_state: 42) # Create a machine learning model (e.g., Support Vector Machine) model = SVC(kernel: 'linear') # Train the model model.fit(X_train, y_train) # Evaluate the model accuracy = model.score(X_test, y_test) puts "Model Accuracy: #{accuracy}"
In this code:
- We split the preprocessed EEG data into training and testing sets.
- We create a simple Support Vector Machine (SVM) classifier using the SVC class from scikit-learn. You can choose other classification algorithms based on your specific task.
- The model is trained using the training data, and its accuracy is evaluated on the testing data.
- This is a basic example to get you started with machine learning on EEG data. Depending on your project, you can explore more advanced techniques, such as deep learning or time-series analysis.
5. Real-World Applications
Now that you’ve learned how to acquire, preprocess, and analyze EEG data in Ruby, let’s explore some real-world applications of BCI and EEG technology.
5.1. Brain-Controlled Prosthetics
BCI technology has the potential to revolutionize the lives of individuals with limb disabilities. By interpreting brain signals, it’s possible to control prosthetic limbs, allowing users to perform intricate tasks with precision.
5.2. Cognitive Rehabilitation
EEG-based BCI systems are used in cognitive rehabilitation programs. These systems monitor patients’ cognitive states and provide real-time feedback and exercises tailored to their needs, aiding in recovery after brain injuries or strokes.
5.3. Mind-Enhanced Gaming
Imagine playing video games with your thoughts! BCI technology is making this a reality. Gamers can control characters, objects, or actions in games simply by thinking about them, providing a more immersive gaming experience.
5.4. Neurofeedback and Mental Health
Neurofeedback is a technique that uses EEG to provide individuals with real-time information about their brain activity. It’s used to help individuals manage stress, anxiety, and attention disorders by training them to modulate their brain activity consciously.
5.5. Brain-Computer Communication
BCI technology enables individuals with severe physical disabilities, such as ALS or locked-in syndrome, to communicate with the outside world. Users can compose messages, browse the internet, or control their environment using only their brain signals.
6. Challenges and Future Directions
While BCI and EEG technology hold immense promise, they also face several challenges:
6.1. Signal Quality
EEG signals can be sensitive to noise, which can affect the accuracy of BCI systems. Improving signal quality is an ongoing challenge in the field.
6.2. Training Time
Training BCI models often requires significant time and effort from both users and researchers. Reducing the training time and making BCIs more user-friendly is a priority.
6.3. Ethical Considerations
As BCIs advance, ethical questions about privacy, security, and potential misuse of neural data need to be addressed.
6.4. Accessibility
Making BCI technology accessible to a broader audience, including those with limited resources or technical expertise, remains a challenge.
The future of BCI and EEG technology looks promising. Researchers are exploring new frontiers, such as brain-to-brain communication and advanced neurofeedback techniques. As the technology becomes more widespread, we can expect to see even more innovative applications in various domains.
Conclusion
In this blog, we’ve embarked on an exciting journey into the world of Brain-Computer Interaction (BCI) and Electroencephalography (EEG). We’ve explored the fundamentals of BCI, the role of EEG in BCI systems, and how to build a simple EEG data acquisition system using Ruby.
We’ve also delved into the integration of machine learning with EEG data, highlighting the potential for pattern recognition and prediction. Real-world applications of BCI and EEG technology showcase the profound impact it can have on healthcare, gaming, mental health, and accessibility.
While challenges remain, the future of BCI and EEG technology holds promise for improving the lives of individuals with disabilities, enhancing gaming experiences, advancing neuroscience research, and opening up new avenues for human-machine interaction.
As you continue your journey into this fascinating field, remember that the possibilities are limited only by your imagination and the boundaries of technology. Whether you’re a developer, a researcher, or simply curious, the world of BCI and EEG awaits your exploration, and Ruby can be your gateway to unlocking its potential.
Table of Contents
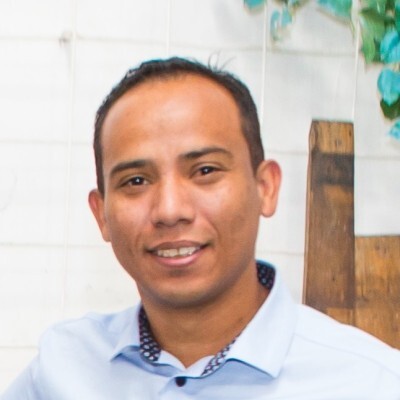
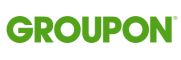