Building Recommendation Systems with Ruby: Collaborative Filtering and Personalization
In today’s digital age, recommendation systems have become an integral part of our online experiences. Whether it’s suggesting movies on Netflix, products on Amazon, or music on Spotify, these systems play a crucial role in enhancing user engagement and satisfaction. In this blog post, we’ll explore how to build recommendation systems with Ruby, focusing on collaborative filtering and personalization techniques. We’ll walk you through the concepts, provide step-by-step guidance, and include code samples to get you started on your recommendation system journey.
Table of Contents
1. Understanding Recommendation Systems
Before we dive into building recommendation systems with Ruby, let’s briefly understand what recommendation systems are and why they matter.
1.1. What Are Recommendation Systems?
Recommendation systems, often referred to as recommender systems, are a subclass of information filtering systems that predict a user’s preferences or interests and make personalized recommendations accordingly. These systems leverage historical user data and item data to provide relevant and tailored content to users.
1.2. Why Are Recommendation Systems Important?
Recommendation systems offer several benefits:
- Enhanced User Experience: Users are more likely to engage with a platform that provides personalized content, leading to increased user satisfaction.
- Increased User Retention: By suggesting relevant items, recommendation systems can keep users coming back for more, boosting user retention.
- Improved Conversions: In e-commerce, personalized product recommendations can lead to higher conversion rates and increased sales.
- Content Discovery: Recommendation systems help users discover new content they might not have found otherwise, promoting content diversity.
Now that we have a basic understanding of recommendation systems and their significance, let’s move on to building one using Ruby.
2. Collaborative Filtering: A Fundamental Recommendation Technique
Collaborative filtering is one of the most widely used techniques in recommendation systems. It relies on the idea that users who have interacted with items in similar ways in the past will continue to do so in the future. There are two main types of collaborative filtering: user-based and item-based. We’ll focus on user-based collaborative filtering in this tutorial.
2.1. User-Based Collaborative Filtering
User-based collaborative filtering involves finding users who are similar to the target user and recommending items that these similar users have liked. Here’s a high-level overview of the steps involved:
- User Similarity Calculation: Calculate the similarity between the target user and other users based on their interactions with items. Common similarity measures include cosine similarity and Pearson correlation.
- Neighbor Selection: Select a subset of users (neighbors) who are most similar to the target user.
- Item Recommendation: Recommend items that the target user’s neighbors have liked but the target user has not interacted with.
Now, let’s implement user-based collaborative filtering using Ruby.
ruby # Collaborative Filtering - User-Based # Sample user-item interactions data user_item_data = { 'User1': { 'Item1': 5, 'Item2': 4, 'Item3': 2 }, 'User2': { 'Item1': 3, 'Item2': 5, 'Item3': 4 }, 'User3': { 'Item1': 4, 'Item2': 3, 'Item4': 5 }, # ... Add more users and their interactions } # Calculate user similarity (Cosine Similarity) def cosine_similarity(user1, user2, data) common_items = data[user1].keys & data[user2].keys dot_product = common_items.reduce(0) do |sum, item| sum + data[user1][item] * data[user2][item] end magnitude_user1 = Math.sqrt(data[user1].values.map { |rating| rating**2 }.sum) magnitude_user2 = Math.sqrt(data[user2].values.map { |rating| rating**2 }.sum) dot_product / (magnitude_user1 * magnitude_user2) end # Find neighbors for the target user def find_neighbors(target_user, data) similarities = {} data.each do |user, _| next if user == target_user similarities[user] = cosine_similarity(target_user, user, data) end # Sort users by similarity in descending order similarities.sort_by { |_, similarity| -similarity } end # Recommend items to the target user def recommend_items(target_user, data) neighbors = find_neighbors(target_user, data) recommended_items = {} neighbors.each do |neighbor, similarity| data[neighbor].each do |item, rating| # Only recommend items the target user has not interacted with recommended_items[item] ||= 0 recommended_items[item] += similarity * rating if !data[target_user].key?(item) end end # Sort recommended items by score in descending order recommended_items.sort_by { |_, score| -score } end # Example usage target_user = 'User1' recommended_items = recommend_items(target_user, user_item_data) puts "Recommended items for #{target_user}:" recommended_items.each { |item, _| puts item }
In this code sample, we calculate user similarity using cosine similarity, find similar users (neighbors), and recommend items to the target user based on their neighbors’ preferences.
3. Personalization: Taking Recommendations to the Next Level
While collaborative filtering provides valuable recommendations based on user behavior patterns, personalization takes it a step further by considering individual user preferences and behavior history. Ruby offers various libraries and techniques for personalization, including content-based filtering and matrix factorization. Let’s explore content-based filtering as a personalization technique.
3.1. Content-Based Filtering
Content-based filtering recommends items to users based on the attributes of the items and the user’s past interactions. It focuses on understanding the content of the items and matching it with user preferences.
Here’s how content-based filtering works:
- Feature Extraction: For each item, extract relevant features or attributes. For movies, these attributes might include genre, director, and actors.
- User Profile: Create a user profile based on the items the user has interacted with. This profile includes the user’s preferences for different features.
- Item Recommendation: Recommend items that match the user’s profile by comparing the item’s features with the user’s preferences.
Let’s implement content-based filtering in Ruby using a simple example.
ruby # Content-Based Filtering # Sample item attributes data item_attributes = { 'Item1': { 'Genre': 'Action', 'Director': 'Director1', 'Actor': 'Actor1' }, 'Item2': { 'Genre': 'Drama', 'Director': 'Director2', 'Actor': 'Actor2' }, 'Item3': { 'Genre': 'Action', 'Director': 'Director3', 'Actor': 'Actor1' }, # ... Add more items and their attributes } # User preferences (example user) user_preferences = { 'Genre': 'Action', 'Actor': 'Actor1' } # Calculate item relevance to the user def calculate_item_relevance(user_preferences, item_attributes) relevance_scores = {} item_attributes.each do |item, attributes| relevance_scores[item] = 0 attributes.each do |attribute, value| if user_preferences.key?(attribute) && user_preferences[attribute] == value relevance_scores[item] += 1 end end end relevance_scores end # Recommend items based on relevance def recommend_items_personalized(user_preferences, item_attributes) relevance_scores = calculate_item_relevance(user_preferences, item_attributes) # Sort items by relevance in descending order relevance_scores.sort_by { |_, relevance| -relevance } end # Example usage recommended_items_personalized = recommend_items_personalized(user_preferences, item_attributes) puts "Personalized recommended items for the user:" recommended_items_personalized.each { |item, _| puts item }
In this code sample, we calculate the relevance of items to a user based on their preferences and attributes of the items. Items are then recommended based on their relevance scores.
4. Building a Complete Recommendation System
To build a complete recommendation system, you can combine collaborative filtering and personalization techniques. Here’s a high-level overview of how you can integrate both approaches:
- Collaborative Filtering: Use collaborative filtering to provide initial recommendations based on user behavior patterns and similarities with other users.
- Personalization: Apply personalization techniques, such as content-based filtering, to further refine the recommendations based on individual user preferences.
- Feedback Loop: Implement a feedback loop to continuously improve recommendations based on user feedback and interactions.
- Evaluation: Measure the performance of your recommendation system using metrics like accuracy, precision, and recall.
Remember that building recommendation systems is an iterative process, and there are various advanced techniques and libraries available to enhance their performance further.
Conclusion
In this blog post, we’ve explored the fundamentals of building recommendation systems with Ruby, focusing on collaborative filtering and personalization techniques. We’ve provided code samples and step-by-step guidance to help you get started on your recommendation system journey. Recommendation systems have a significant impact on user engagement and satisfaction, making them a valuable addition to any application or platform. By mastering these techniques in Ruby, you can create personalized experiences that keep users coming back for more. So, go ahead, dive into the code, and start building your own recommendation system today!
Table of Contents
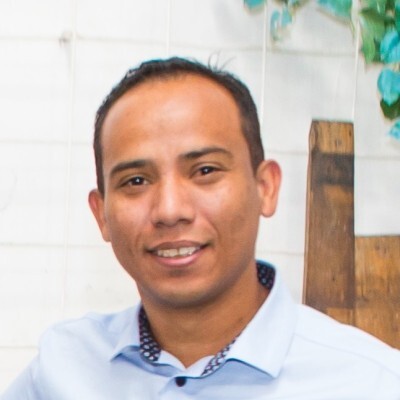
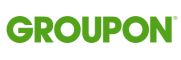