Building Web Scrapers with Ruby: Crawling and Extracting Information
In today’s data-driven world, the ability to extract valuable information from websites is crucial for businesses, researchers, and developers. Web scraping, the process of automatically gathering data from websites, has become an essential skill. Ruby, a versatile and elegant programming language, provides a robust framework for building web scrapers that can crawl websites and extract relevant data efficiently. In this guide, we’ll delve into the fundamentals of web scraping with Ruby, covering crawling techniques, data extraction methods, and best practices for creating effective and ethical web scrapers.
Table of Contents
1. Understanding Web Scraping
1.1. What is Web Scraping?
Web scraping involves extracting information from websites in an automated manner. This process allows you to gather data such as text, images, links, and more, which can then be used for analysis, research, or integration with other systems. Ruby’s simplicity and expressiveness make it an excellent choice for developing web scrapers that can navigate through web pages and retrieve desired content.
1.2. Ethics of Web Scraping
While web scraping offers incredible potential, it’s crucial to approach it ethically and responsibly. Always review a website’s terms of service and robots.txt file to ensure you’re not violating any rules. Additionally, avoid overloading servers with excessive requests, as this can lead to denial of service for other users. Prioritize selective and respectful scraping to maintain the integrity of both your scraper and the targeted websites.
2. Getting Started with Ruby Web Scraping
2.1. Installing Dependencies
Before you begin building a web scraper, ensure that you have Ruby installed on your system. You’ll also need the ‘nokogiri’ gem, a powerful HTML and XML parsing library for Ruby. Install it using the following command:
ruby gem install nokogiri
2.2. Navigating Web Pages
The cornerstone of web scraping is navigating web pages and extracting information. The ‘nokogiri’ gem simplifies this process by providing tools to parse and traverse HTML documents. Let’s see how to fetch a web page and extract its title using Ruby:
ruby require 'nokogiri' require 'open-uri' url = 'https://www.example.com' page = Nokogiri::HTML(URI.open(url)) title = page.css('title').text puts "Page title: #{title}"
2.3. Extracting Links
Extracting links from a web page is a common task in web scraping. The following code snippet demonstrates how to fetch all the links from a page:
ruby links = page.css('a').map { |link| link['href'] } puts "Links on the page: #{links}"
3. Crawling Websites with Ruby
3.1. Introduction to Crawling
Crawling involves systematically navigating through a website’s pages to gather information from multiple sources. This technique is useful when you want to collect data from various pages within a domain. A simple example of crawling involves recursively visiting each page and extracting relevant data.
3.2. Depth-First vs. Breadth-First Crawling
Depth-first crawling involves visiting a page and exploring its links before moving on to the next level. On the other hand, breadth-first crawling visits all the pages at the current level before descending to the next level. Choose the appropriate crawling strategy based on the structure of the website and the information you need.
Example: Depth-First Crawling
Let’s create a basic depth-first web crawler using Ruby. In this example, we’ll navigate through a website and extract the headings from each page:
ruby def crawl_page(url) page = Nokogiri::HTML(URI.open(url)) headings = page.css('h1, h2, h3').map(&:text) puts "Headings on #{url}:" puts headings links = page.css('a').map { |link| link['href'] } links.each do |link| absolute_link = URI.join(url, link).to_s crawl_page(absolute_link) if absolute_link.start_with?(url) end end starting_url = 'https://www.example.com' crawl_page(starting_url)
4. Data Extraction Techniques
4.1. Extracting Text and Attributes
Beyond simple navigation, web scraping often involves extracting specific data such as text and attributes from HTML elements. The ‘nokogiri’ gem provides various methods to retrieve these details. For instance:
ruby element = page.css('.element-class').first text = element.text attribute_value = element['attribute_name']
4.2. Handling Pagination
When dealing with websites that display data across multiple pages, pagination comes into play. To scrape data from multiple pages, you need to identify and iterate through the different pages, extracting the desired information. Here’s an example of scraping data from a paginated website:
ruby current_page = 1 base_url = 'https://www.example.com/page=' loop do url = "#{base_url}#{current_page}" page = Nokogiri::HTML(URI.open(url)) # Extract data from the current page # ... current_page += 1 break unless page.css('.next-page').any? end
4.3. Using APIs for Structured Data
In cases where a website provides an API to access structured data, using the API is often a more efficient and reliable approach than traditional scraping. APIs offer standardized endpoints to retrieve data in JSON or XML format, eliminating the need to parse HTML. However, APIs might not provide all the data available on the website.
5. Best Practices for Effective Web Scraping
5.1. Respect Robots.txt
Always honor a website’s robots.txt file, which provides guidelines on which pages can be crawled and which should be avoided. Adhering to these guidelines shows respect for the website owner’s wishes and helps you avoid legal issues.
5.2. Limit Requests
Avoid overwhelming servers with too many requests in a short period. Use throttling techniques to space out requests and prevent overloading the server. This approach also helps maintain a low profile and minimizes the chances of being blocked.
5.3. Use Custom Headers
Some websites might block or limit access to scrapers based on user agent strings. To overcome this, set a custom user agent header in your HTTP requests to mimic a regular browser’s behavior.
5.4. Handle Errors Gracefully
Network errors, timeouts, and unexpected HTML structures are common challenges in web scraping. Implement error-handling mechanisms to gracefully handle these situations, log errors, and continue scraping when possible.
5.5. Test Regularly
Website structures can change unexpectedly, causing your scraper to break. Regularly test your scraper to ensure it’s still functioning as expected. Implement monitoring to receive alerts when something goes wrong.
Conclusion
Web scraping with Ruby empowers developers to extract valuable information from websites efficiently and ethically. By combining the power of the ‘nokogiri’ gem with crawling techniques and data extraction strategies, you can create robust web scrapers that gather the data you need for your projects. Remember to approach web scraping responsibly, respecting website terms of service and robots.txt files. With the knowledge and skills gained from this guide, you’re ready to embark on your web scraping journey with Ruby. Happy scraping!
Table of Contents
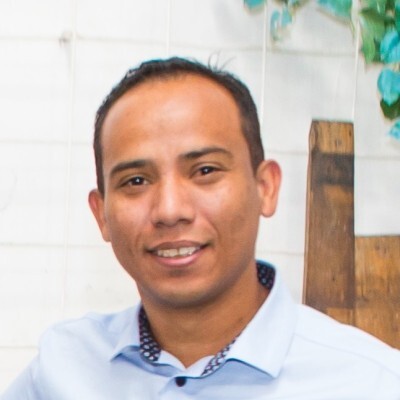
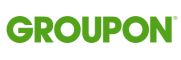