Ruby Q & A
How to check if a string contains a specific substring in Ruby?
In Ruby, you can check if a string contains a specific substring using various methods and techniques. Here are some common approaches:
- `include?` Method: You can use the `include?` method to check if a string contains a specific substring. This method returns `true` if the substring is found within the string and `false` otherwise.
```ruby text = "Hello, world!" substring = "world" if text.include?(substring) puts "The text contains the substring." else puts "The text does not contain the substring." end ```
In this example, the code will output “The text contains the substring.”
- Regular Expressions: Ruby supports regular expressions for more complex pattern matching. You can use the `=~` operator with a regular expression to check for the presence of a substring.
```ruby text = "Hello, world!" substring = /world/ if text =~ substring puts "The text contains the substring." else puts "The text does not contain the substring." end ```
This code will also output “The text contains the substring.”
- `index` Method: The `index` method can be used to find the starting position of a substring within a string. If the substring is not found, it returns `nil`.
```ruby text = "Hello, world!" substring = "world" if text.index(substring) puts "The text contains the substring." else puts "The text does not contain the substring." end ```
This code will yield “The text contains the substring.”
These methods provide different ways to check for the presence of a substring in a string, allowing you to choose the one that best suits your specific use case and coding style. Whether you need a simple check or more advanced pattern matching, Ruby provides the tools to accomplish the task efficiently.
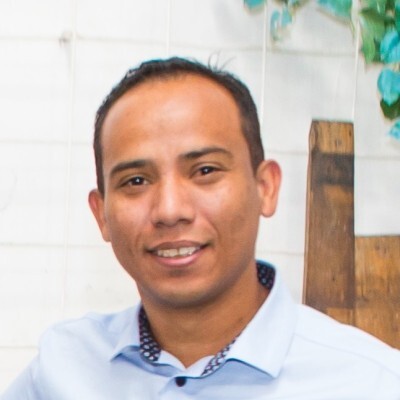
Previously at
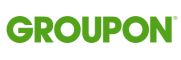
Experienced software professional with a strong focus on Ruby. Over 10 years in software development, including B2B SaaS platforms and geolocation-based apps.