How to define a class in Ruby?
In Ruby, defining a class is a fundamental concept in object-oriented programming. A class serves as a blueprint for creating objects, allowing you to encapsulate data and behavior into a single unit. Here’s how you define a class in Ruby:
- Using the `class` Keyword: To define a class in Ruby, you use the `class` keyword, followed by the class name (which should start with an uppercase letter) and a block of code enclosed by `do…end` or curly braces `{}`. Inside the class block, you can define attributes (instance variables) and methods (functions) that describe the behavior and properties of objects created from the class.
```ruby class Person def initialize(name, age) @name = name @age = age end def introduce puts "Hi, I'm #{@name}, and I'm #{@age} years old." end end ```
In this example, we’ve defined a `Person` class with two instance variables (`@name` and `@age`) and two methods (`initialize` and `introduce`). The `initialize` method is a special constructor method called when a new object is created from the class, while the `introduce` method prints a message.
- Creating Objects (Instances): Once you’ve defined a class, you can create objects (also known as instances) of that class. To create a new instance, use the class name followed by `.new` and provide any required arguments to the `initialize` method.
```ruby person1 = Person.new("Alice", 30) person2 = Person.new("Bob", 25) ```
Here, we’ve created two `Person` objects, `person1` and `person2`, with different name and age values.
- Accessing Attributes and Methods: You can access the attributes and methods of an object using dot notation (`.`). For example, to access the `introduce` method and print the introduction message:
```ruby person1.introduce # Outputs: Hi, I'm Alice, and I'm 30 years old. person2.introduce # Outputs: Hi, I'm Bob, and I'm 25 years old. ```
Defining classes in Ruby allows you to create organized, reusable, and modular code. Classes are the building blocks of object-oriented programming, enabling you to model real-world entities and their behaviors. They encapsulate data and methods, promoting code encapsulation and abstraction, which are fundamental principles of object-oriented design.
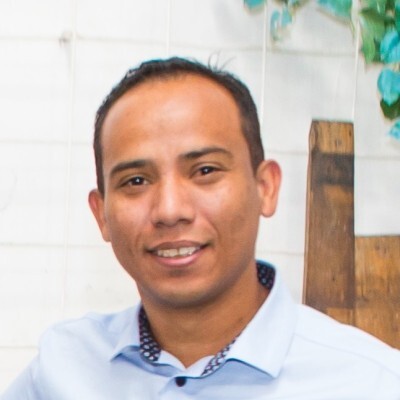
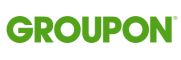