Explain the concept of variable scope in Ruby and the differences between local, instance, and global variables.
In Ruby, variable scope determines where in your code a variable can be accessed and modified. Understanding variable scope is crucial for writing maintainable and bug-free Ruby programs. Ruby has three main types of variable scope: local, instance, and global variables, each with its own rules and usage.
- Local Variables:
– Local variables have the most limited scope; they are confined to the specific block or method where they are defined.
– These variables begin with a lowercase letter or underscore (_).
– They are not accessible outside the block or method where they are defined.
– Example:
```ruby def my_method local_var = 42 end ```
In this example, `local_var` is a local variable within the `my_method` method and cannot be accessed from outside it.
- Instance Variables:
– Instance variables are prefixed with the `@` symbol and are accessible throughout an instance of a class.
– They have a broader scope than local variables but are limited to the specific instance of an object.
– Instance variables are used to store data that should be available across different methods within an object.
– Example:
```ruby class MyClass def set_value(value) @instance_var = value end def get_value @instance_var end end ```
Here, `@instance_var` is an instance variable that can be accessed and modified within any method of the `MyClass` instance.
- Global Variables:
– Global variables are prefixed with the `$` symbol and have the broadest scope, making them accessible from anywhere in your Ruby code.
– However, it’s essential to use global variables sparingly as they can lead to unintended side effects and make code less maintainable.
– Example:
```ruby $global_var = "I'm accessible everywhere!" def some_method puts $global_var end ```
In this example, `$global_var` can be accessed from both `some_method` and other parts of your code.
Local variables are confined to their defining blocks, instance variables are accessible throughout an object instance, and global variables can be accessed from anywhere in your code. It’s essential to choose the appropriate variable scope based on your program’s requirements to ensure clean and maintainable code while minimizing potential bugs and side effects.
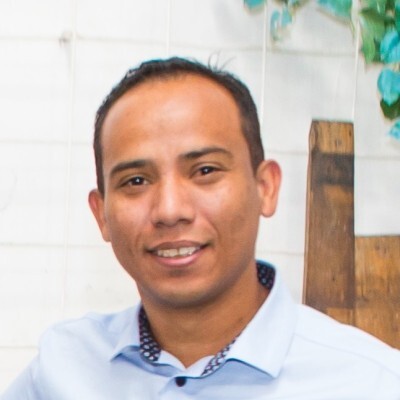
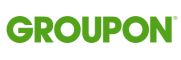