How to convert between different data types in Ruby?
In Ruby, you can convert between different data types using a variety of methods and operators. This flexibility allows you to manipulate data and perform type conversions as needed. Here are some common ways to convert between data types in Ruby:
- Type Casting: Ruby provides methods to explicitly cast data from one type to another. For example, you can use `.to_i` to convert a value to an integer, `.to_f` to convert to a floating-point number, and `.to_s` to convert to a string.
```ruby number = "42" integer = number.to_i floating_point = number.to_f ```
Here, `integer` will hold the integer `42`, and `floating_point` will contain the floating-point number `42.0`.
- String Interpolation: You can implicitly convert values to strings by using string interpolation within double-quoted strings.
```ruby age = 30 message = "I am #{age} years old." ```
In this case, `age` is automatically converted to a string and included in the `message`.
- Concatenation: When you concatenate a string with other data types using the `+` operator, Ruby automatically converts the non-string values to strings.
```ruby text = "The answer is: " + 42.to_s ```
Here, `42` is converted to a string and appended to the original string.
- Explicit Conversions: You can use explicit conversion methods like `.to_i`, `.to_f`, or `.to_s` to ensure the desired data type when converting.
- Array and Hash Conversions: Arrays and hashes can be converted to each other using methods like `.to_a` and `.to_h`.
```ruby array = { "key1" => "value1", "key2" => "value2" }.to_a hash = [["key1", "value1"], ["key2", "value2"]].to_h ```
These methods and techniques provide the flexibility to convert between different data types in Ruby, allowing you to work with data in the format that best suits your needs. Be aware of potential data loss or errors when performing conversions, especially for complex data structures.
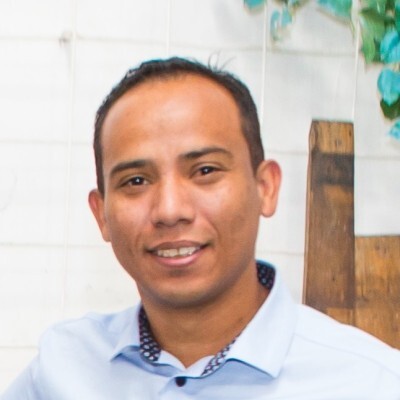
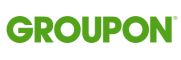