Ruby Q & A
How to create a simple command-line tool in Ruby?
Ruby is a versatile language for building command-line applications. To create a basic command-line tool, follow these steps:
- Set up Ruby: Ensure that you have Ruby installed on your system. You can check this by running `ruby -v` in your terminal. If it’s not installed, you can download it from the official Ruby website (https://www.ruby-lang.org/en/documentation/installation/) or use a package manager like `apt` or `brew`.
- Create a Ruby script: Start by creating a new Ruby file with a `.rb` extension. This file will contain your command-line tool’s code. For example, let’s create a file called `my_tool.rb`.
- Shebang line: Add a shebang line at the beginning of your Ruby script to specify the interpreter. In most cases, it will be `#!/usr/bin/env ruby`.
- Accept command-line arguments: To accept input from the command line, you can use the `ARGV` array, which stores the arguments passed to your script. For example, `ARGV[0]` will hold the first argument.
- Implement functionality: Write the code that your command-line tool should execute based on the provided arguments. You can use conditional statements and functions to perform specific actions.
- Display output: Use `puts` or `print` statements to display results or messages to the user.
- Make the script executable: Run `chmod +x my_tool.rb` to make your Ruby script executable.
- Test your tool: Execute your command-line tool by running `./my_tool.rb` with appropriate arguments to ensure it works as expected.
- Documentation: Consider adding comments or documentation within your script to explain its usage and functionality to users.
That’s it! You’ve created a simple command-line tool in Ruby. As you become more familiar with Ruby, you can explore libraries like OptionParser to handle command-line arguments more robustly and make your tool more user-friendly.
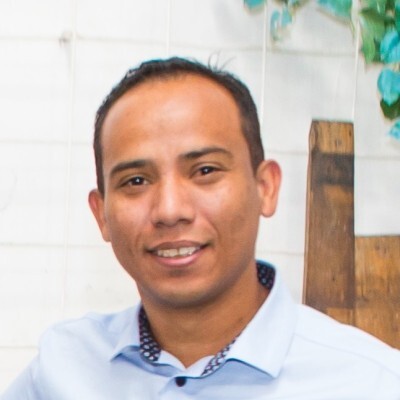
Previously at
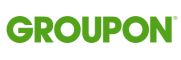
Experienced software professional with a strong focus on Ruby. Over 10 years in software development, including B2B SaaS platforms and geolocation-based apps.