How to create and use a custom exception in Ruby?
In Ruby, creating and using custom exceptions is a powerful way to handle exceptional cases in your code and provide clear and meaningful error messages to users. Custom exceptions allow you to define your own error types and provide context-specific information when an error occurs.
Here are the steps to create and use a custom exception in Ruby:
- Create a Custom Exception Class:
To create a custom exception, you define a new class that inherits from the built-in `Exception` or a more specific exception class like `StandardError`. You can add custom behavior and attributes to your exception class as needed.
```ruby class MyCustomError < StandardError def initialize(message) super(message) end end ```
In this example, we’ve created a custom exception class named `MyCustomError` that inherits from `StandardError` and accepts a custom error message.
- Raise the Custom Exception:
To raise your custom exception when an exceptional situation occurs in your code, you can use the `raise` keyword followed by an instance of your custom exception class. You can also provide an optional error message.
```ruby def my_method # Some code that may raise an error raise MyCustomError, "This is a custom error message." end ```
- Rescue and Handle the Custom Exception:
To catch and handle your custom exception or any other exception, you can use the `begin…rescue` block in your code. You specify the exception type you want to rescue, and then you can provide custom error-handling logic.
```ruby begin my_method rescue MyCustomError => e puts "Custom error occurred: #{e.message}" end ```
Here, we rescue the `MyCustomError` exception, display a custom error message, and handle the exceptional situation gracefully.
Using custom exceptions in Ruby helps you create more robust and maintainable code by clearly defining error conditions and providing informative error messages. It also makes your code easier to understand and debug, as it separates the normal code flow from error handling logic.
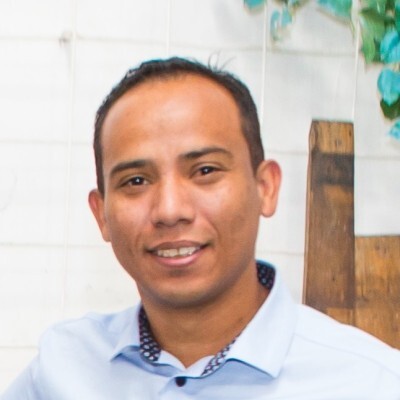
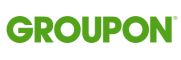