How do I create an object from a Ruby class?
In Ruby, creating an object from a class involves a straightforward process. Objects are instances of a class, and you can use the class’s constructor method to create them. Here’s how to create an object from a Ruby class:
- Define the Class: First, you need to define a class that serves as a blueprint for the type of objects you want to create. A class encapsulates data (attributes) and behavior (methods) related to a specific entity or concept. Here’s an example of a simple `Person` class:
```ruby class Person def initialize(name, age) @name = name @age = age end end ```
In this `Person` class, the `initialize` method is used as the constructor, setting the initial state of `name` and `age` attributes.
- Create an Object: To create an object from the class, you use the class name followed by `.new`, optionally passing any required arguments to the `initialize` method. The `.new` method initializes an instance of the class and returns it.
```ruby person1 = Person.new("Alice", 30) person2 = Person.new("Bob", 25) ```
In this example, we’ve created two `Person` objects, `person1` and `person2`, with different `name` and `age` values.
- Access Object Properties and Methods: Once you’ve created an object, you can access its properties (attributes) and methods using dot notation (`.`). For example, to access the `name` attribute and call the `initialize` method:
```ruby puts person1.name # Outputs: "Alice" person2.initialize("Charlie", 28) # Reinitializes person2 with new values ```
You can access and manipulate the object’s properties and invoke its methods to perform various tasks and operations.
Creating objects from classes is a fundamental concept in object-oriented programming, allowing you to model real-world entities and their behavior. Each object you create from a class is a unique instance with its own set of attributes and can respond to the methods defined in the class. This enables you to build modular and organized code that represents and interacts with different objects in your application.
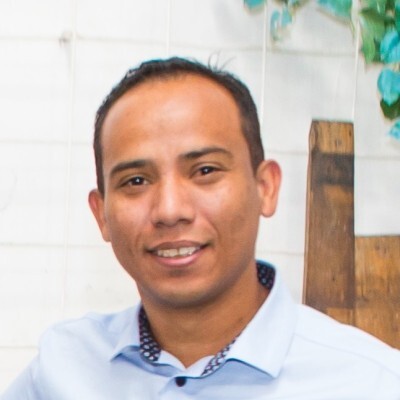
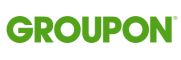