Creating Interactive Web Applications with Ruby and React.js
In today’s fast-paced digital landscape, creating interactive web applications is essential to engage users and deliver a seamless online experience. Ruby and React.js are two powerful technologies that, when combined, can help you build dynamic and user-friendly web applications. Ruby, with its elegant syntax and powerful frameworks like Ruby on Rails, is an excellent choice for building robust backends. React.js, on the other hand, is a JavaScript library for building user interfaces that are both dynamic and user-friendly.
Table of Contents
In this blog post, we will guide you through the process of creating interactive web applications by combining Ruby and React.js. We’ll start by setting up your development environment and building a backend using Ruby on Rails. Then, we’ll move on to creating the frontend with React.js, connecting the two parts, and even adding user authentication. Finally, we’ll discuss deployment options to make your web application accessible to the world.
1. Setting Up Your Development Environment
Before we dive into building our interactive web application, let’s make sure our development environment is properly set up. You’ll need:
- Ruby: Ensure you have Ruby installed on your system. You can use a version manager like RVM or rbenv for better control over Ruby versions.
- Node.js: React.js requires Node.js for development. Download and install Node.js from the official website.
- Text Editor/IDE: Choose a text editor or integrated development environment (IDE) of your choice. Popular options include Visual Studio Code, Sublime Text, and RubyMine.
- Git: Version control is crucial for collaborative development. Install Git if you haven’t already.
2. Building a Backend with Ruby
2.1. Setting Up Ruby on Rails
Ruby on Rails, often referred to as Rails, is a powerful web application framework that makes it easy to build robust backend services. To get started, open your terminal and run the following commands:
bash # Install Rails gem install rails # Create a new Rails application rails new my_ruby_backend # Move into the application directory cd my_ruby_backend
This will create a new Rails application with all the necessary files and configurations.
2.2. Creating a RESTful API
Now that we have our Rails application set up, let’s create a simple RESTful API. We’ll generate a resource called “todos” as an example. Run the following commands:
bash # Generate a Todo model and controller rails generate scaffold Todo title:string completed:boolean # Run database migrations rails db:migrate
This will generate a Todo model, a controller, and the necessary routes for handling CRUD (Create, Read, Update, Delete) operations on todos.
3. Creating the Frontend with React.js
3.1. Setting Up a React Application
With our backend in place, it’s time to create the frontend using React.js. We’ll use Create React App, a popular tool for setting up React projects quickly.
bash # Create a new React.js application npx create-react-app my_react_frontend # Move into the application directory cd my_react_frontend
Now, you have a basic React.js application ready to go.
3.2. Building Interactive Components
React.js is all about creating reusable and interactive components. Let’s create a simple Todo component that will fetch data from our Ruby backend and display it.
jsx import React, { useState, useEffect } from 'react'; function TodoList() { const [todos, setTodos] = useState([]); useEffect(() => { // Fetch todos from the backend fetch('/api/todos') .then((response) => response.json()) .then((data) => setTodos(data)); }, []); return ( <div> <h1>Todo List</h1> <ul> {todos.map((todo) => ( <li key={todo.id}>{todo.title}</li> ))} </ul> </div> ); } export default TodoList;
In this code, we’re using the useState and useEffect hooks to manage the state of our todos and fetch data from the backend API.
4. Connecting the Backend and Frontend
Now that we have our backend and frontend components ready, let’s connect them.
4.1. Making API Requests
In your React component, you can use the fetch API to make requests to your Ruby backend. We’re fetching the list of todos in our TodoList component, as shown above.
4.2. Handling Responses
To handle responses from the backend, you can use promises and the then method. In the example, we’re parsing the response as JSON and updating the component’s state with the retrieved data.
5. Adding User Authentication
User authentication is a critical feature for many web applications. You can add authentication to your Ruby on Rails backend using popular gems like Devise or Auth0. On the frontend, you can implement authentication flows with libraries like React Router and Redux.
6. Deploying Your Web Application
Once your web application is ready, it’s time to deploy it to a production environment. There are several hosting options available, including:
- Heroku: Heroku is a cloud platform that simplifies deployment and scaling. It supports both Ruby on Rails and React.js applications.
- Netlify: Netlify is a popular choice for deploying frontend applications. It offers continuous deployment and easy integration with Git repositories.
- AWS: Amazon Web Services provides a range of services for hosting web applications, including AWS Elastic Beanstalk for Ruby on Rails and AWS Amplify for React.js.
Choose a hosting platform that suits your project’s requirements and budget.
Conclusion
In this guide, we’ve explored how to create interactive web applications using Ruby and React.js. We started by setting up our development environment, building a Ruby on Rails backend, and creating a React.js frontend. We connected the two parts, added user authentication, and discussed deployment options.
By combining the power of Ruby and React.js, you can build modern and user-friendly web applications that meet the expectations of today’s users. Whether you’re creating a personal project or working on a professional application, these technologies will help you deliver a seamless online experience. So, roll up your sleeves, start coding, and bring your interactive web application to life!
Table of Contents
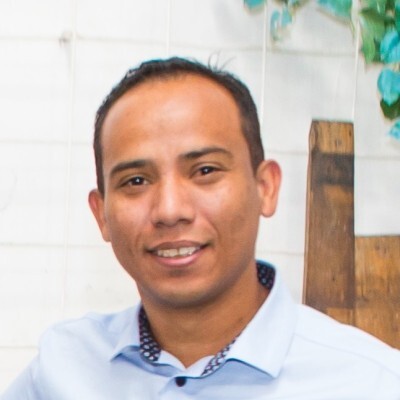
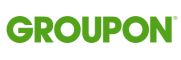