Creating Machine Learning Models with Ruby: Leveraging Libraries like SciRuby
Machine learning has emerged as a transformative technology with applications ranging from self-driving cars to medical diagnostics. While Python has traditionally been the dominant programming language for machine learning, developers and data scientists are increasingly exploring alternatives. One such alternative is Ruby, a versatile and elegant programming language known for its readability and expressiveness. In this blog post, we’ll delve into the world of creating machine learning models using Ruby, with a focus on leveraging libraries like SciRuby. We’ll cover the essential concepts, libraries, and provide code samples to help you get started on your journey.
Table of Contents
1. Why Ruby for Machine Learning?
Python has undoubtedly been the go-to language for machine learning due to its robust ecosystem of libraries like TensorFlow, scikit-learn, and PyTorch. However, Ruby, with its clean syntax and object-oriented design, also presents itself as a viable option. For developers already familiar with Ruby, the prospect of building machine learning models without switching languages is enticing. Additionally, Ruby’s focus on simplicity and readability aligns well with machine learning’s intricate concepts, making it an excellent choice for those new to the field.
2. Introducing SciRuby
When it comes to creating machine learning models with Ruby, one of the standout libraries is SciRuby. SciRuby is an ecosystem of Ruby gems designed to bring the power of scientific computing to Ruby. It includes tools for numerical computation, data visualization, and machine learning. Let’s explore some of the key components of SciRuby that will be instrumental in our machine learning journey.
2.1. Numo: Numerical Computing in Ruby
Numo, short for “Numerical Ruby,” is a fundamental component of SciRuby. It provides a suite of numerical computation capabilities akin to libraries like NumPy in Python. With Numo, you can effortlessly manipulate arrays, perform mathematical operations, and handle multi-dimensional data. Here’s a quick example of using Numo to perform a basic operation:
ruby require 'numo/narray' a = Numo::DFloat[1, 2, 3] b = Numo::DFloat[4, 5, 6] c = a + b puts c
In this code snippet, we import the Numo gem, create two arrays, and then add them element-wise to obtain a new array c. This simplicity and familiarity are what make Ruby a compelling choice for numerical computations.
2.2. Daru: Data Analysis and Manipulation
Daru is another gem within the SciRuby ecosystem that focuses on data analysis and manipulation. Similar to pandas in Python, Daru allows you to work with structured data using data frames. You can load data from various sources, clean and transform it, and perform exploratory data analysis. Here’s an example of loading data from a CSV file and performing basic operations using Daru:
ruby require 'daru' data_frame = Daru::DataFrame.from_csv('data.csv') puts data_frame.head(5) # Display the first 5 rows # Calculate the mean of a column mean_column = data_frame['column_name'].mean puts "Mean: #{mean_column}"
Daru’s intuitive API simplifies data handling tasks, enabling you to focus on the analysis itself.
2.3. SciKit-Learn for Ruby: Machine Learning Made Easy
Machine learning wouldn’t be complete without a robust library for model creation and training. SciKit-Learn for Ruby, inspired by scikit-learn in Python, fills this role in the SciRuby ecosystem. It provides a wide range of algorithms for classification, regression, clustering, and more. Let’s see how easy it is to create a simple classification model using SciKit-Learn for Ruby:
ruby require 'scikit-learn' # Load the dataset iris = Sklearn::Datasets.load_iris X = iris.data y = iris.target # Split the dataset into training and testing sets X_train, X_test, y_train, y_test = Sklearn::ModelSelection.train_test_split(X, y, test_size: 0.2) # Create a support vector machine classifier classifier = Sklearn::SVM::SVC.new classifier.fit(X_train, y_train) # Evaluate the model accuracy = classifier.score(X_test, y_test) puts "Accuracy: #{accuracy}"
In this snippet, we load the famous Iris dataset, split it into training and testing sets, create a support vector machine classifier, and evaluate its accuracy. SciKit-Learn for Ruby encapsulates complex machine learning processes into easy-to-use APIs.
3. Putting It All Together: A Simple Machine Learning Workflow
Now that we’ve explored the essential components of SciRuby, let’s walk through a simplified machine learning workflow using these tools.
Step 1: Data Preparation
Suppose we have a dataset containing information about houses, including their sizes and prices. We want to create a model that predicts house prices based on their sizes. First, we’ll use Daru to load and preprocess the data:
ruby require 'daru' data_frame = Daru::DataFrame.from_csv('house_data.csv') # Separate features (size) and target (price) features = data_frame['size'].to_a target = data_frame['price'].to_a
Step 2: Model Creation and Training
Next, we’ll use SciKit-Learn for Ruby to create and train a regression model:
ruby require 'scikit-learn' # Convert features and target to Numo arrays features = Numo::DFloat.cast(features) target = Numo::DFloat.cast(target) # Split the dataset X_train, X_test, y_train, y_test = Sklearn::ModelSelection.train_test_split(features, target, test_size: 0.2) # Create and train a linear regression model regressor = Sklearn::LinearModel::LinearRegression.new regressor.fit(X_train, y_train)
Step 3: Model Evaluation
Finally, we’ll evaluate the model’s performance:
ruby # Predict house prices on the test set predictions = regressor.predict(X_test) # Calculate the mean squared error mse = Sklearn::Metrics.mean_squared_error(y_test, predictions) puts "Mean Squared Error: #{mse}"
Conclusion
In this blog post, we’ve scratched the surface of creating machine learning models with Ruby by leveraging libraries like SciRuby. While Python remains a dominant player in the machine learning world, Ruby offers an elegant and accessible alternative. The SciRuby ecosystem, comprising gems like Numo, Daru, and SciKit-Learn for Ruby, equips developers with the tools needed to perform numerical computations, data analysis, and machine learning tasks seamlessly. Whether you’re a Ruby enthusiast or simply looking to expand your programming horizons, exploring machine learning with Ruby is an endeavor worth considering.
Remember that the examples provided here are just a glimpse of what’s possible. As you dive deeper into the world of machine learning with Ruby, you’ll discover a plethora of libraries and resources waiting to be explored. So, roll up your sleeves, fire up your favorite text editor, and start building your own machine learning models with Ruby!
Table of Contents
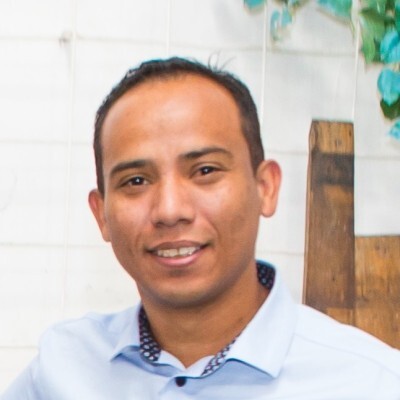
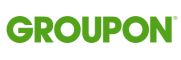