Creating RESTful APIs with Ruby: Building Robust Web Services
In today’s interconnected world, building robust and efficient web services has become essential for seamless data exchange and integration between applications. Representational State Transfer (REST) has emerged as a popular architectural style for designing scalable and maintainable web APIs. Ruby, a versatile and expressive programming language, offers a plethora of tools and libraries that make creating RESTful APIs a breeze.
Table of Contents
In this blog, we’ll delve into the world of RESTful APIs with Ruby, exploring best practices and showcasing code samples to build powerful web services. We’ll cover the fundamentals of REST, setting up a Ruby environment, handling HTTP methods, data serialization, authentication, and more. By the end of this guide, you’ll be equipped with the knowledge to create efficient and flexible APIs that can effortlessly interact with other services.
1. Understanding RESTful APIs
1.1. What is REST?
REST (Representational State Transfer) is an architectural style that provides a set of constraints and principles for designing networked applications. It emphasizes a stateless client-server communication model, where the server exposes resources, and clients perform actions on these resources using standard HTTP methods like GET, POST, PUT, and DELETE. RESTful APIs focus on resource-based URLs and use standard status codes for indicating the success or failure of a request.
1.2. Key Principles of REST
- Statelessness: Each client request to the server must contain all the information needed to understand and process it. The server should not store any client context between requests.
- Resource-Based URLs: RESTful APIs represent resources as unique URLs, making it easy to access and manipulate them using standard HTTP methods.
- HTTP Methods: RESTful APIs utilize HTTP methods, such as GET for reading data, POST for creating new resources, PUT for updating existing resources, and DELETE for removing resources.
- Uniform Interface: A uniform interface simplifies the interaction between clients and servers, promoting a consistent and predictable API design.
1.3. Advantages of RESTful APIs
- Scalability: RESTful APIs are designed to be scalable, allowing you to handle large numbers of concurrent requests efficiently.
- Interoperability: By using standard HTTP methods and data formats like JSON or XML, RESTful APIs can be easily consumed by a wide range of clients, regardless of their programming language or platform.
- Simplicity and Understandability: RESTful APIs follow a straightforward design philosophy, making them easy to understand and use for both developers and clients.
2. Setting Up the Ruby Environment
2.1. Installing Ruby
Before we start building RESTful APIs with Ruby, ensure you have Ruby installed on your system. You can download and install Ruby from the official website (https://www.ruby-lang.org/en/downloads/).
2.2. Choosing a Framework: Sinatra vs. Ruby on Rails
Ruby offers several frameworks to build web applications and APIs, but two of the most popular choices are Sinatra and Ruby on Rails.
Sinatra: Sinatra is a lightweight and flexible web framework that allows you to quickly create APIs with minimal boilerplate code. It is well-suited for small to medium-sized projects where simplicity is preferred.
Ruby on Rails: Ruby on Rails, often referred to as Rails, is a full-fledged web framework that provides a robust structure for building large-scale applications, including APIs. Rails follows the convention over configuration principle, which accelerates development by minimizing the number of decisions developers need to make.
For this blog, we’ll use Sinatra to showcase the essentials of building RESTful APIs. Sinatra’s simplicity will allow us to focus on the core concepts without getting lost in the details of a larger framework.
2.3. Bundler: Managing Dependencies
Bundler is a package manager for Ruby that helps manage gem dependencies for your projects. To set up Bundler for your API project, create a Gemfile in your project’s root directory and add the following content:
ruby source 'https://rubygems.org' gem 'sinatra' gem 'json'
Run the following command to install the dependencies:
bash $ bundle install
3. Handling HTTP Methods
3.1. The Role of HTTP Methods in REST
HTTP methods play a crucial role in defining the actions that can be performed on resources within a RESTful API.
- GET: Used to retrieve resource representations or collections of resources.
- POST: Used to create new resources. It often involves submitting data to be processed by the API.
- PUT: Used to update existing resources or create resources at a specific URL.
- DELETE: Used to remove resources.
3.2. Implementing GET, POST, PUT, and DELETE Requests
Let’s create a simple Sinatra application that demonstrates how to handle these HTTP methods.
ruby require 'sinatra' require 'json' # Sample data - Replace this with a database or data storage of your choice tasks = [ { id: 1, title: 'Buy groceries', completed: false }, { id: 2, title: 'Walk the dog', completed: true }, { id: 3, title: 'Write blog post', completed: false } ] # Get all tasks get '/tasks' do tasks.to_json end # Get a specific task get '/tasks/:id' do id = params[:id].to_i task = tasks.find { |t| t[:id] == id } if task task.to_json else status 404 { error: "Task with id #{id} not found." }.to_json end end # Create a new task post '/tasks' do data = JSON.parse(request.body.read) new_task = { id: tasks.length + 1, title: data['title'], completed: false } tasks << new_task status 201 new_task.to_json end # Update a task put '/tasks/:id' do id = params[:id].to_i task = tasks.find { |t| t[:id] == id } if task data = JSON.parse(request.body.read) task[:title] = data['title'] if data['title'] task[:completed] = data['completed'] unless data['completed'].nil? task.to_json else status 404 { error: "Task with id #{id} not found." }.to_json end end # Delete a task delete '/tasks/:id' do id = params[:id].to_i task_index = tasks.index { |t| t[:id] == id } if task_index tasks.delete_at(task_index) status 204 else status 404 { error: "Task with id #{id} not found." }.to_json end end
In this example, we have a collection of tasks stored in memory (for demonstration purposes). We define routes for each of the HTTP methods: GET, POST, PUT, and DELETE. The tasks array acts as our temporary data storage.
To run the application, save the code above to a file (e.g., app.rb) and execute the following command:
bash $ ruby app.rb
Now you can interact with the API using your preferred HTTP client or browser.
4. Data Serialization
4.1. Choosing the Right Data Format (JSON vs. XML)
Data serialization is the process of converting data objects into a specific format suitable for transmission or storage. JSON (JavaScript Object Notation) and XML (eXtensible Markup Language) are two common data formats used for APIs.
JSON is lightweight and human-readable, making it the preferred choice for most RESTful APIs due to its simplicity and ease of use. However, XML still has its place in certain enterprise environments and legacy systems.
4.2. Serializing Data Objects
To serialize Ruby objects into JSON, we can use the built-in to_json method provided by the json gem.
ruby require 'json' class Task attr_accessor :id, :title, :completed def initialize(id, title, completed) @id = id @title = title @completed = completed end def to_json(*options) { id: @id, title: @title, completed: @completed }.to_json(*options) end end # Usage task = Task.new(1, 'Sample Task', false) serialized_task = task.to_json puts serialized_task
The output will be a JSON representation of the task object:
json {"id":1,"title":"Sample Task","completed":false}
4.3. Deserializing Client Requests
To deserialize incoming JSON data in a POST or PUT request, we can use the JSON.parse method.
ruby # Example Sinatra route for creating a new task post '/tasks' do data = JSON.parse(request.body.read) # Now, 'data' is a Ruby hash containing the JSON data from the client request # ... end
5. Versioning Your APIs
5.1. Why API Versioning is Essential
As your API evolves and changes over time, you may need to introduce updates that could potentially break existing clients. API versioning is crucial to ensure backward compatibility while still allowing for improvements and new features.
5.2. URL-based Versioning vs. Request Header Versioning
There are several approaches to versioning RESTful APIs, but two common methods are URL-based versioning and request header versioning.
URL-based Versioning: In this approach, the API version is included in the URL itself, such as /v1/tasks and /v2/tasks. While straightforward, this method may clutter the API URLs and lead to maintenance challenges as the number of versions grows.
Request Header Versioning: In this approach, the client includes the API version in the request header, allowing for a cleaner and more maintainable URL structure. For example, the client may send a Accept-Version: v1 header with the request.
5.3. Managing Multiple API Versions
To demonstrate URL-based versioning, let’s modify our previous Sinatra application to include two versions of the /tasks route.
ruby # Sample data - Replace this with a database or data storage of your choice tasks_v1 = [ { id: 1, title: 'Buy groceries', completed: false }, # ... ] tasks_v2 = [ { id: 1, name: 'Buy groceries', done: false }, # ... ] # Version 1 - Get all tasks get '/v1/tasks' do tasks_v1.to_json end # Version 2 - Get all tasks get '/v2/tasks' do tasks_v2.to_json end
With this implementation, clients can choose the appropriate version of the API by selecting the corresponding URL.
6. Authentication and Security
6.1. Implementing API Key Authentication
API key authentication is a simple way to control access to your API. In this method, clients must include an API key with each request to prove their identity.
ruby # Simple API key authentication middleware class ApiKeyAuthentication API_KEY = 'YOUR_API_KEY' # Replace this with your actual API key def initialize(app) @app = app end def call(env) if env['HTTP_X_API_KEY'] == API_KEY @app.call(env) else [401, { 'Content-Type' => 'application/json' }, [{ error: 'Unauthorized' }.to_json]] end end end # Usage in Sinatra use ApiKeyAuthentication
Clients need to include the X-API-KEY header with their requests:
vbnet GET /tasks X-API-KEY: YOUR_API_KEY
6.2. Token-Based Authentication with JSON Web Tokens (JWT)
JSON Web Tokens (JWT) are a popular choice for token-based authentication. They are compact, URL-safe, and can securely carry user information between client and server.
To implement JWT authentication, you’ll need the jwt gem. Add it to your Gemfile:
ruby gem 'jwt'
Now, you can use JWT to generate and verify tokens in your Sinatra application.
ruby require 'jwt' # Helper method to generate a JWT token def generate_token(data) JWT.encode(data, 'YOUR_SECRET_KEY', 'HS256') end # Helper method to verify a JWT token def verify_token(token) JWT.decode(token, 'YOUR_SECRET_KEY', true, algorithm: 'HS256') end # Example usage in a Sinatra route post '/login' do # Assuming you have a user authentication logic here user = authenticate_user(params[:username], params[:password]) if user token = generate_token(user_id: user.id) { token: token }.to_json else status 401 { error: 'Invalid credentials' }.to_json end end
In this example, we generate and return a JWT token after successful user authentication. Clients can include this token in subsequent requests’ Authorization header to access protected routes.
6.3. Securing Your API with SSL/TLS
Securing your API with SSL/TLS (HTTPS) is crucial to protect data during transmission. To enable SSL in your Sinatra application, you need to have a valid SSL certificate and private key.
ruby # Enable SSL in Sinatra set :bind, '0.0.0.0' set :port, 443 set :server_settings, { SSLEnable: true, SSLCertName: 'your_domain.crt', SSLPrivateKey: 'your_domain.key' }
Ensure you replace ‘your_domain.crt’ and ‘your_domain.key’ with the paths to your SSL certificate and private key files.
7. Pagination and Filtering
Efficiently Handling Large Data Sets
Pagination is essential when dealing with large data sets to improve API performance and prevent overwhelming the client with too much data at once. It allows clients to request a limited number of records per page and navigate through the data gradually.
7.1. Implementing Pagination
Let’s enhance our /tasks endpoint to include pagination support.
ruby # Sample data - Replace this with a database or data storage of your choice tasks = [ # ... ] # Get all tasks with pagination support get '/tasks' do page = params.fetch('page', 1).to_i per_page = params.fetch('per_page', 10).to_i paginated_tasks = tasks.slice((page - 1) * per_page, per_page) total_pages = (tasks.length.to_f / per_page).ceil { tasks: paginated_tasks, total_pages: total_pages, current_page: page }.to_json end
Clients can specify the desired page and the number of tasks per page as query parameters:
bash GET /tasks?page=2&per_page=5
The response will include the requested tasks for the given page, the total number of pages, and the current page number.
7.2. Filtering Resources with Query Parameters
Filtering allows clients to request specific data from the API based on certain criteria. Let’s enhance our /tasks endpoint to include filtering support by task completion status.
ruby # Get all tasks with filtering support get '/tasks' do completed_filter = params['completed'] filtered_tasks = if completed_filter.nil? tasks else tasks.select { |task| task[:completed] == (completed_filter == 'true') } end paginated_tasks = filtered_tasks.slice((page - 1) * per_page, per_page) total_pages = (filtered_tasks.length.to_f / per_page).ceil { tasks: paginated_tasks, total_pages: total_pages, current_page: page }.to_json end
Clients can filter tasks based on completion status using the completed query parameter:
bash GET /tasks?completed=true
This will return only completed tasks. If the completed parameter is not provided, all tasks will be returned.
8. Error Handling and Status Codes
8.1. Common HTTP Status Codes
RESTful APIs use standard HTTP status codes to communicate the success or failure of a request. Some common status codes include:
- 200 OK: The request was successful, and the server returns the requested data.
- 201 Created: The request was successful, and a new resource has been created.
- 204 No Content: The request was successful, but there is no additional information to send (e.g., in response to a successful DELETE request).
- 400 Bad Request: The request could not be understood or was malformed.
- 401 Unauthorized: The request requires user authentication or the provided credentials are invalid.
- 403 Forbidden: The server understood the request, but it refuses to authorize it.
- 404 Not Found: The requested resource could not be found on the server.
- 500 Internal Server Error: A generic error message returned when an unexpected condition was encountered.
8.2. Proper Error Responses in JSON Format
When returning errors in a RESTful API, it’s essential to provide informative and consistent error responses. Here’s an example of how to structure error responses in JSON format:
ruby # Helper method for rendering error responses def render_error(status, message) status status { error: message }.to_json end # Usage in a Sinatra route get '/tasks/:id' do id = params[:id].to_i task = tasks.find { |t| t[:id] == id } if task task.to_json else render_error(404, "Task with id #{id} not found.") end end
By using the render_error helper method, we ensure that error responses adhere to a consistent structure across all routes.
Conclusion
Building RESTful APIs with Ruby provides developers with powerful tools to create robust and scalable web services. We’ve covered the essential concepts of REST, setting up the Ruby environment, handling HTTP methods, data serialization, authentication, versioning, pagination, filtering, error handling, and status codes.
With this knowledge, you have a solid foundation to start building your own RESTful APIs with Ruby, whether you choose Sinatra for simplicity or Ruby on Rails for larger-scale projects. Remember to follow best practices, keep your API secure, and adhere to the principles of REST for a seamless integration experience with other web services.
Happy coding!
Table of Contents
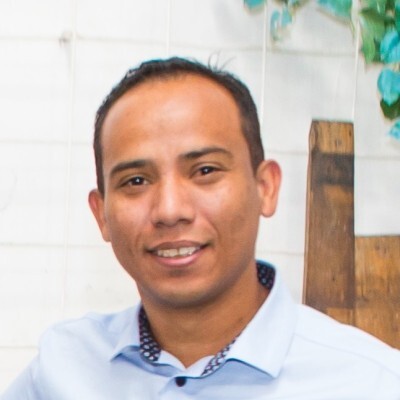
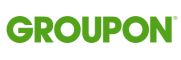