Creating Voice Applications with Ruby: Integrating Speech Recognition and Synthesis
Voice technology has rapidly evolved in recent years, transforming the way we interact with devices and applications. From voice assistants to smart home devices, speech recognition and synthesis have become integral parts of modern user experiences. Ruby, a dynamic and versatile programming language, provides developers with a solid foundation for creating voice applications that can understand and generate human-like speech. In this tutorial, we’ll explore how to harness the power of speech recognition and synthesis in Ruby to build interactive and engaging voice applications.
Table of Contents
1. Introduction
Voice technology has revolutionized the way we interact with our devices and applications. Whether it’s asking a voice assistant for weather updates or controlling smart home devices with voice commands, speech recognition and synthesis have become essential components of modern user interfaces. In this tutorial, we’ll delve into the world of voice applications and learn how to integrate speech recognition and synthesis capabilities into Ruby projects.
2. Setting Up Your Environment
Before we dive into creating voice applications, let’s ensure our development environment is properly set up.
2.1. Installing Required Gems
Ruby offers a variety of gems that simplify working with voice technology. We’ll start by installing the necessary gems using the following commands:
ruby gem install speech_recognition gem install festival
2.2. Configuring API Keys
To access speech recognition and synthesis services, you’ll often need API keys. Sign up for the respective services and obtain API keys. Store these keys securely as environment variables in your project. You can access them in your code using ENV[‘API_KEY’].
3. Speech Recognition
Speech recognition allows applications to understand spoken language and convert it into text. The speech_recognition gem provides an interface to easily integrate this capability into your Ruby projects.
3.1. Using the SpeechRecognition Gem
First, let’s require the gem and set up the recognition engine:
ruby require 'speech_recognition' recognizer = SpeechRecognition::Recognizer.new
3.2. Capturing and Interpreting Speech
To capture speech and convert it to text, use the following code:
ruby audio = recognizer.capture_audio text = recognizer.recognize(audio) puts "You said: #{text}"
This captures audio from the microphone, processes it, and displays the recognized text.
4. Text-to-Speech Synthesis
Text-to-speech synthesis involves converting text into natural-sounding speech. The festival gem allows us to accomplish this with ease.
4.1. Utilizing the Festival Gem
Begin by requiring the gem and configuring the synthesis engine:
ruby require 'festival' festival = Festival::Client.new
4.2. Converting Text to Natural Speech
To synthesize speech from text, use the following code:
ruby text = "Hello, welcome to the voice application tutorial." audio = festival.text_to_wave(text) audio.save("welcome.wav")
This converts the provided text into audio and saves it as a WAV file.
5. Building a Voice-Enabled Application
Now that we understand how to perform speech recognition and synthesis, let’s create a simple voice-enabled application.
5.1. Designing the Application Flow
For our example, let’s build a basic voice-controlled calculator. The user will speak a mathematical expression, the application will evaluate it, and then respond with the result.
5.2. Integrating Speech Recognition and Synthesis
Here’s a snippet demonstrating the integration of speech recognition and synthesis in our calculator application:
ruby # ... (previous code) puts "Please speak a mathematical expression:" audio = recognizer.capture_audio expression = recognizer.recognize(audio) begin result = eval(expression) response = "The result of #{expression} is #{result}" rescue response = "Sorry, I couldn't understand the expression." end festival.text_to_wave(response).play # ... (remaining code)
This code captures the user’s spoken expression, evaluates it, and responds with the result in synthesized speech.
6. Enhancing User Experience
To create effective voice applications, it’s crucial to enhance the user experience through proper error handling and user feedback.
6.1. Error Handling and Feedback
Incorporate error handling to gracefully manage situations where speech recognition fails or mathematical expressions are invalid. Provide meaningful feedback to the user to guide them through the process.
6.2. Implementing Voice Commands
Expand your application by implementing specific voice commands. For instance, you could allow the user to ask for the weather or set reminders using voice inputs.
7. Real-World Applications
The possibilities with voice applications are vast. Here are a couple of real-world application ideas to inspire you:
7.1. Voice-Controlled Virtual Assistant
Create a virtual assistant similar to popular voice assistants. Users can ask questions, set reminders, check their schedule, and even control smart devices using voice commands.
7.2. Interactive Storytelling App
Build an app that narrates interactive stories based on user choices. The app can dynamically generate and speak storylines, making the storytelling experience immersive and engaging.
Conclusion
Ruby provides a solid foundation for creating voice applications that integrate speech recognition and synthesis capabilities. By following this guide, you’ve learned how to capture and interpret speech, synthesize natural-sounding speech, and build voice-enabled applications. The world of voice technology is constantly evolving, and by leveraging the power of Ruby, you can create innovative and captivating voice applications that enhance user experiences across various domains.
Table of Contents
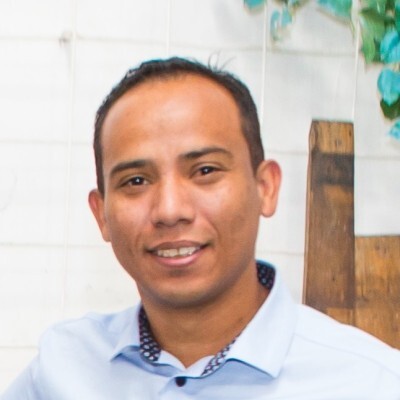
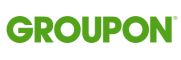