Exploring Ruby’s Cryptography Functions: Encrypting and Decrypting Data
In today’s digital age, data security has become a paramount concern. Whether you’re working with sensitive user information, financial records, or any other confidential data, protecting it from unauthorized access is crucial. This is where cryptography comes into play, and Ruby provides a robust set of functions for encrypting and decrypting data. In this blog post, we’ll delve into the world of cryptography in Ruby, exploring how to leverage its capabilities to safeguard your information.
Table of Contents
1. Understanding Cryptography and Encryption
1.1. What is Cryptography?
Cryptography is the practice of secure communication techniques that safeguard information from adversaries. It involves transforming plaintext into ciphertext using cryptographic algorithms and keys. The process of encryption ensures that even if unauthorized parties gain access to the encrypted data, they won’t be able to understand or utilize it without the appropriate decryption key.
1.2. The Importance of Encryption
Encryption plays a vital role in maintaining data confidentiality and integrity. It’s used in various scenarios, such as online transactions, sensitive communication, password storage, and more. Without encryption, sensitive data would be susceptible to interception, unauthorized access, and tampering. By employing encryption techniques, businesses and individuals can ensure that their data remains protected, even if it falls into the wrong hands.
2. Introducing Ruby’s Cryptography Libraries
Ruby offers several libraries that provide robust cryptographic functions, making it easier to implement encryption and decryption in your applications. Some of the notable libraries include:
2.1. OpenSSL
OpenSSL is a widely-used open-source library that provides various cryptographic services, including SSL/TLS protocols, digital signatures, and encryption. Ruby’s OpenSSL module allows you to harness the power of OpenSSL’s encryption capabilities within your Ruby code.
ruby require 'openssl' data = 'sensitive information' password = 'secretpassword' cipher = OpenSSL::Cipher.new('AES-256-CFB') cipher.encrypt cipher.key = Digest::SHA256.digest(password) encrypted_data = cipher.update(data) + cipher.final puts "Encrypted data: #{encrypted_data}"
2.2. Digest
The Digest module in Ruby is primarily used for generating hash values (message digests) of data. While not for encryption itself, it’s an essential part of cryptographic applications, especially for verifying data integrity and creating secure password hashes.
ruby require 'digest' password = 'secretpassword' hashed_password = Digest::SHA256.hexdigest(password) puts "Hashed password: #{hashed_password}"
2.3. SecureRandom
The SecureRandom module provides methods for generating cryptographically secure random numbers and strings. This is particularly useful for generating encryption keys and initialization vectors.
ruby require 'securerandom' encryption_key = SecureRandom.random_bytes(32) iv = SecureRandom.random_bytes(16) puts "Encryption Key: #{encryption_key.unpack('H*').first}" puts "Initialization Vector: #{iv.unpack('H*').first}"
3. Encrypting Data with Ruby
3.1. Using Symmetric Encryption
Symmetric encryption involves using a single secret key for both encryption and decryption. The same key is used to transform plaintext into ciphertext and back again.
ruby require 'openssl' data = 'sensitive information' encryption_key = 'supersecretkey' cipher = OpenSSL::Cipher.new('AES-256-CFB') cipher.encrypt cipher.key = encryption_key encrypted_data = cipher.update(data) + cipher.final puts "Encrypted data: #{encrypted_data}"
3.2. Implementing Asymmetric Encryption
Asymmetric encryption, also known as public-key cryptography, utilizes a pair of keys: a public key for encryption and a private key for decryption. Data encrypted with the public key can only be decrypted with the corresponding private key, providing an extra layer of security.
ruby require 'openssl' data = 'sensitive information' private_key = OpenSSL::PKey::RSA.generate(2048) public_key = private_key.public_key encrypted_data = public_key.public_encrypt(data) # Simulating transmission and decryption decrypted_data = private_key.private_decrypt(encrypted_data) puts "Decrypted data: #{decrypted_data}"
4. Decrypting Data with Ruby
4.1. Symmetric Decryption
Decrypting symmetrically encrypted data requires the same key used for encryption. This key should be kept secret to maintain the confidentiality of the data.
ruby require 'openssl' encrypted_data = '...' # Encrypted data obtained from encryption process encryption_key = 'supersecretkey' cipher = OpenSSL::Cipher.new('AES-256-CFB') cipher.decrypt cipher.key = encryption_key decrypted_data = cipher.update(encrypted_data) + cipher.final puts "Decrypted data: #{decrypted_data}"
4.2. Asymmetric Decryption
To decrypt data that has been asymmetrically encrypted, you need the corresponding private key.
ruby require 'openssl' encrypted_data = '...' # Encrypted data obtained from encryption process # Assuming you have the private key object private_key = OpenSSL::PKey::RSA.new(File.read('private_key.pem')) decrypted_data = private_key.private_decrypt(encrypted_data) puts "Decrypted data: #{decrypted_data}"
5. Best Practices for Cryptographic Usage
While Ruby provides powerful tools for cryptography, using them correctly is crucial to ensure the security of your data. Here are some best practices to keep in mind:
5.1. Key Management
- Keep encryption keys secret and well-protected.
- Use strong, random keys to resist brute-force attacks.
- Regularly rotate keys to mitigate potential breaches.
5.2. Secure Storage
- Store keys and sensitive data in secure locations.
- Avoid hardcoding keys directly into your code.
- Utilize secure key management systems.
5.3. Regular Updates
- Keep your Ruby environment and cryptographic libraries up to date.
- Follow industry best practices and guidelines.
- Stay informed about any security vulnerabilities in the libraries you use.
Conclusion
In conclusion, understanding and implementing cryptography in Ruby empowers you to secure your data effectively. Whether you’re encrypting sensitive information symmetrically or asymmetrically, Ruby’s cryptography functions provide the tools you need to safeguard your data from unauthorized access. By following best practices and staying informed about the latest developments in the field, you can ensure the confidentiality and integrity of your data in today’s ever-evolving digital landscape.
Table of Contents
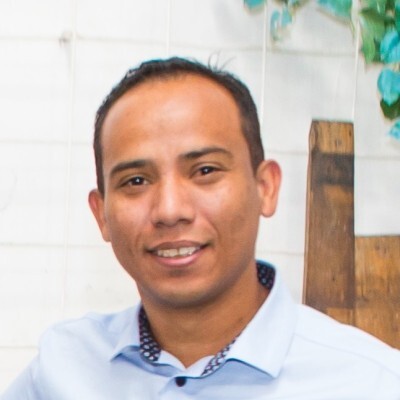
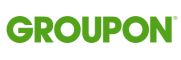