Ruby for Cybersecurity: Protecting Applications and Data
In an increasingly interconnected world, where digital threats are ever-evolving, cybersecurity has become an essential aspect of software development. As applications handle sensitive data and perform critical operations, the need for robust security measures cannot be overstated. Ruby, a versatile and elegant programming language, might not be the first choice that comes to mind when discussing cybersecurity. However, its unique features and extensive libraries make it a valuable ally in fortifying applications and safeguarding data from potential vulnerabilities.
Table of Contents
1. Understanding the Role of Ruby in Cybersecurity
1.1. Ruby’s Versatility and Power
Ruby is renowned for its simplicity and readability, allowing developers to create clean and concise code. This simplicity, combined with the language’s dynamic nature, enables rapid development and iteration. While these traits make Ruby an attractive choice for developers, they also hold immense value in the realm of cybersecurity.
When securing applications and data, quick identification and resolution of vulnerabilities is crucial. Ruby’s dynamic nature allows developers to modify and patch code on the fly, facilitating rapid responses to emerging threats. This agility is a significant advantage in combating potential security breaches.
1.2. The Ruby Community and Security Collaboration
One of Ruby’s strengths lies in its passionate and collaborative community. This community actively contributes to the development of security tools, libraries, and best practices. Rubyists often work together to identify and address security vulnerabilities promptly, making the language a safer choice for building robust applications.
2. Strategies for Using Ruby in Cybersecurity
2.1. Input Validation and Sanitization
Unvalidated and unsanitized user inputs are common entry points for attacks like SQL injection and cross-site scripting (XSS). Ruby provides a variety of methods and libraries to validate and sanitize inputs effectively.
ruby # Example: Input validation using regular expressions input = params[:user_input] if input.match?(/\A\d+\z/) # Input is a valid integer # Proceed with processing else # Handle invalid input end
2.2. Secure Authentication and Authorization
Proper authentication and authorization mechanisms are pivotal in preventing unauthorized access to sensitive resources. Ruby’s gems like Devise and CanCanCan simplify user authentication and authorization, enabling developers to enforce security measures seamlessly.
ruby # Example: Using Devise for user authentication class UsersController < ApplicationController before_action :authenticate_user! def show # Fetch user data end end
2.3. Encryption and Hashing
Data encryption and hashing are essential for protecting sensitive information, such as passwords and personal data. Ruby offers libraries like OpenSSL for encryption and BCrypt for hashing.
ruby # Example: Using BCrypt for password hashing and verification require 'bcrypt' password = 'secretpassword' hashed_password = BCrypt::Password.create(password) # Store 'hashed_password' in the database # For password verification stored_hashed_password = BCrypt::Password.new(hashed_password) if stored_hashed_password == entered_password # Passwords match else # Passwords do not match end
2.4. Regular Updates and Patch Management
Keeping dependencies and frameworks up-to-date is crucial for maintaining a secure application. Ruby’s package manager, RubyGems, streamlines the process of updating and managing dependencies.
ruby # Update a gem to the latest version gem update gem_name # Update all gems in the system gem update --system
2.5. Secure Coding Practices
Adhering to secure coding practices is fundamental in preventing vulnerabilities. Ruby developers should follow principles like the principle of least privilege, avoiding hardcoded secrets, and using prepared statements to prevent SQL injection.
3. Leveraging Ruby Gems for Enhanced Security
3.1. Brakeman
Brakeman is a static analysis tool specifically designed for Ruby on Rails applications. It scans the codebase for potential security vulnerabilities and provides detailed reports. By integrating Brakeman into the development workflow, developers can catch security issues early in the development process.
ruby # Install Brakeman gem install brakeman # Run Brakeman on a Rails app brakeman
3.2. SecureHeaders
SecureHeaders is a gem that helps in setting security-related HTTP headers for Ruby applications. It assists in preventing various types of attacks, including XSS and clickjacking, by ensuring that the appropriate security headers are included in HTTP responses.
ruby # Install SecureHeaders gem install secure_headers # Configure SecureHeaders in a Rails app SecureHeaders::Configuration.default do |config| config.csp = { default_src: %w['self'], img_src: %w['self' img.example.com], # Other CSP directives } end
Conclusion
In the ever-evolving landscape of cybersecurity, Ruby emerges as a potent tool for protecting applications and data. Its versatility, community support, and array of libraries contribute to its effectiveness in addressing security challenges. By implementing input validation, secure authentication, encryption, and adhering to best coding practices, developers can harness Ruby’s capabilities to build robust and secure software.
Whether you’re developing a web application, a command-line tool, or any other software, Ruby’s unique features can enhance your cybersecurity efforts. As threats continue to evolve, leveraging Ruby’s strengths can help you stay one step ahead in the ongoing battle to secure digital landscapes. So, explore Ruby’s potential, collaborate with the community, and ensure that your applications and data remain shielded from potential vulnerabilities.
Table of Contents
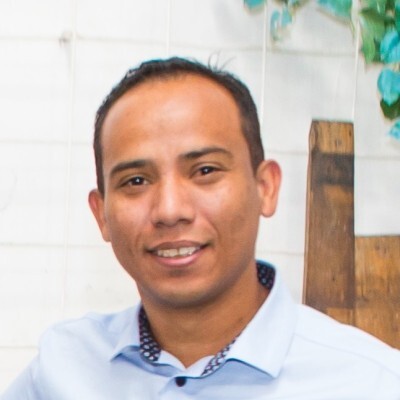
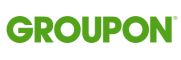