Debugging Ruby Applications: Techniques and Tools for Troubleshooting
Debugging is an essential skill for any developer, as it allows you to identify and fix issues in your code. Ruby, a dynamic and elegant programming language, is widely used for web development, automation, and more. However, like any other language, Ruby applications can encounter bugs and errors. In this article, we’ll dive into various techniques and tools that can help you effectively debug Ruby applications and streamline your troubleshooting process.
Table of Contents
1. Understanding Common Bugs in Ruby
Before we delve into debugging techniques, let’s take a look at some common types of bugs you might encounter when working with Ruby applications:
1.1. Syntax Errors
Syntax errors occur when your code violates the rules of the Ruby language. These errors are often detected by the interpreter and can prevent your code from running altogether. An example of a syntax error:
ruby puts "Hello, World!
1.2. Logic Errors
Logic errors, also known as bugs, occur when your code does not produce the expected output due to flawed logic. These errors can be more challenging to identify, as the code might run without any error messages. Example:
ruby def calculate_total(items) total = 0 items.each do |item| total += item end total * 2 # Incorrect logic, should be 'total' end
1.3. Nil and Undefined Method Errors
Nil errors occur when you attempt to call a method on a nil value, while undefined method errors happen when you try to call a method that doesn’t exist. Example:
ruby name = nil name.downcase # NoMethodError numbers = [1, 2, 3] numbers.nonexistent_method # NoMethodError
2. Techniques for Effective Debugging
Now that we’ve covered some common bugs, let’s explore techniques that can help you effectively debug your Ruby applications.
2.1. Print Debugging
Print debugging is a simple yet powerful technique. By strategically placing puts or p statements in your code, you can output variable values and messages to the console, allowing you to understand the flow of your program and the values of variables at different points. Example:
ruby def calculate_total(items) puts "Calculating total for items: #{items}" total = 0 items.each do |item| total += item end puts "Total: #{total}" total end
2.2. Using binding.pry
The pry gem is a popular debugging tool for Ruby. It provides an interactive console that allows you to pause your code’s execution at a specific point and explore the current state of variables. To use it, you need to install the gem and add binding.pry in your code where you want to inspect. Example:
ruby require 'pry' def find_element(array, target) binding.pry array.index(target) end
While your code is paused at the binding.pry line, you can interact with variables, test expressions, and identify issues.
2.3. Stack Traces and Error Messages
When your Ruby application encounters an error, it often provides a stack trace and an error message. The stack trace shows the sequence of method calls that led to the error, helping you trace back to the problematic code. Understanding stack traces can significantly assist in pinpointing the root cause of the issue.
3. Tools for Ruby Debugging
In addition to techniques, several tools can aid in debugging Ruby applications more efficiently.
3.1. Pry Debugger
The Pry gem not only provides an interactive console but also offers a full-fledged debugger. You can set breakpoints, step through code, and evaluate expressions using this debugger. Example:
ruby require 'pry' def calculate_discounted_total(items, discount) total = 0 binding.pry # Breakpoint items.each do |item| total += item end total - discount end
3.2. Byebug
Byebug is another popular gem that offers a feature-rich debugger. It allows you to step through your code, set breakpoints, and examine variables at different points. To use Byebug, you’ll need to install the gem and add the byebug statement where you want to pause execution. Example:
ruby require 'byebug' def process_data(data) result = [] byebug # Pause execution data.each do |item| result << item * 2 end result end
3.3. Better Errors
Better Errors is a gem that enhances error pages in web applications, providing more detailed information about the error, along with a REPL console for interactive debugging. It’s especially useful for identifying issues in web applications. Just add the gem to your project’s Gemfile and follow the setup instructions.
4. Best Practices for Debugging Ruby Applications
To make your debugging process more efficient and productive, consider the following best practices:
4.1. Use Version Control
Version control systems like Git can be a lifesaver when debugging. By maintaining a history of changes, you can revert to a working state if a bug is introduced, making it easier to identify when the bug was introduced.
4.2. Write Unit Tests
Unit tests can help you catch bugs early in the development process. When you encounter a bug, you can create a test case that reproduces the issue. Fixing the bug becomes a matter of making the test pass.
4.3. Keep Code Simple and Modular
Complex code can be difficult to debug. Aim for simplicity and modularity in your codebase. Break down your code into small, manageable functions or methods. This not only makes debugging easier but also enhances the overall maintainability of your code.
Conclusion
Debugging is an essential skill for every Ruby developer. By understanding common bugs, using effective techniques, leveraging debugging tools, and following best practices, you can streamline your debugging process and maintain the quality and reliability of your Ruby applications. Remember, debugging is not just about finding and fixing errors; it’s also about enhancing your problem-solving skills and becoming a more proficient programmer. Happy debugging!
Table of Contents
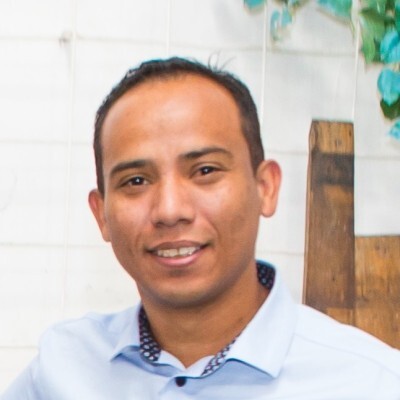
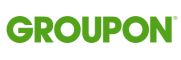