What is the ‘do…end’ block in Ruby?
In Ruby, the `do…end` block is a construct used to define a block of code that can be executed independently or passed as an argument to a method. It’s a way to group multiple statements together and execute them as a single unit. This block syntax is often used for multi-line code blocks, especially when you want to maintain a clean and readable structure.
Here’s how the `do…end` block works:
- Defining a Block:
You can define a block using the `do` keyword followed by a block of code and then ending with the `end` keyword. The code within the block is enclosed within these keywords. Here’s an example:
```ruby 5.times do puts "Hello, Ruby!" end ```
In this example, the `do…end` block contains a `puts` statement that prints “Hello, Ruby!” five times.
- Using a Block with Methods:
Blocks are often used as arguments to methods. A method can yield control to the block using the `yield` keyword. The code within the block is executed when the method reaches the `yield` statement. For example:
```ruby def greet puts "Hello!" yield puts "Goodbye!" end greet do puts "Ruby" end ```
Here, the `greet` method yields control to the block between `do` and `end`, which prints “Ruby” when executed.
- Block Scope:
Variables defined within a `do…end` block are scoped to that block and are not accessible outside of it. This means you can use local variables within a block without worrying about name conflicts in the outer scope.
```ruby x = 10 5.times do x = x + 1 end puts x # Output: 15 ```
In this example, `x` is scoped to the block and is separate from any `x` variable defined in the outer scope.
The `do…end` block is a fundamental construct in Ruby, enabling you to create reusable and organized code blocks for various purposes, such as iteration, conditional execution, and custom behavior within methods. It promotes readability and maintainability by encapsulating related code within a clear and defined structure.
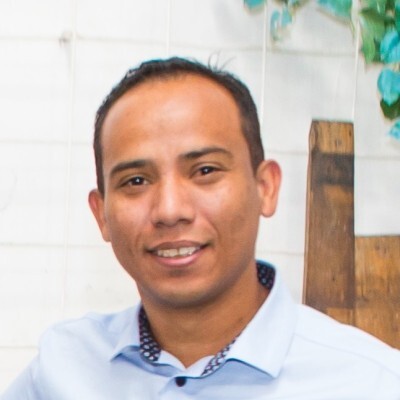
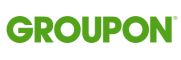