How to define a constant in Ruby?
In Ruby, you can define a constant using the `const` keyword or by simply assigning a value to a variable name that starts with an uppercase letter. Constants are used to store values that should not be changed once they are defined. They are typically used for storing configuration settings, mathematical constants, or any value that remains constant throughout the execution of your program.
Here’s how you can define a constant in Ruby:
- Using the `const` Keyword:
You can explicitly use the `const` keyword to define a constant. This is less common and usually used when you need to access constants dynamically or within a particular scope.
```ruby MyConstant = 42 ```
In this example, `MyConstant` is defined as a constant with the value `42`. Constants in Ruby are conventionally written in all uppercase letters, but it’s not a strict requirement.
- Using Uppercase Variable Names:
A more common and conventional way to define constants in Ruby is by assigning a value to a variable name that starts with an uppercase letter. This is widely accepted and used in Ruby code.
```ruby MY_CONSTANT = 3.14159 ```
Here, `MY_CONSTANT` is defined as a constant with the value `3.14159`. It’s good practice to use uppercase letters and underscores to separate words in constant names for readability.
- Accessing Constants:
Once a constant is defined, you can access it throughout your program. Constants have global scope, so they can be accessed from anywhere within your code.
```ruby puts MY_CONSTANT # Output: 3.14159 ```
You can use constants in expressions, assignments, and as arguments to methods, just like regular variables.
It’s important to note that while Ruby allows you to change the value of a constant, it will generate a warning, and the value will not be truly constant. It’s a convention and best practice to treat constants as immutable and refrain from changing their values during program execution.
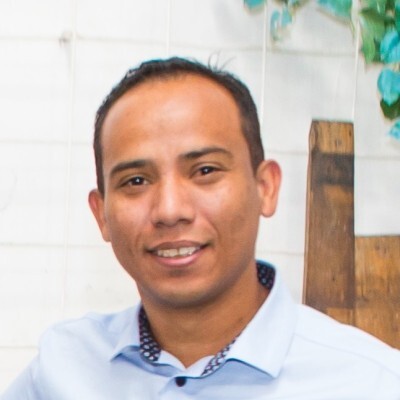
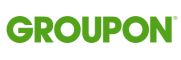