How to define class methods in Ruby?
In Ruby, you can define class methods by prefixing a method definition with the `self` keyword within the class definition. Class methods are called on the class itself, rather than on instances of the class. They are useful for defining behavior that is related to the class as a whole, rather than specific instances of the class.
Here’s how you define and use class methods in Ruby:
```ruby class MyClass # This is a class method def self.my_class_method puts "This is a class method." end # Other instance methods can also be defined here end # Calling the class method MyClass.my_class_method # Output: This is a class method. ```
In the example above, `my_class_method` is a class method because it is defined with `self` in front of its name within the class definition. To call the class method, you use the class name (`MyClass`) followed by the method name (`my_class_method`).
Class methods are often used for utility functions that are related to the class but do not depend on the state of specific instances. They can also be used to create factory methods for creating instances of the class, or to provide convenient ways to access class-level data or configuration settings.
It’s important to note that class methods are different from instance methods, which are called on instances of the class. Class methods operate on the class itself and can be called without creating an instance of the class. This makes them a valuable tool for defining behavior and functionality that is intrinsic to the class as a whole.
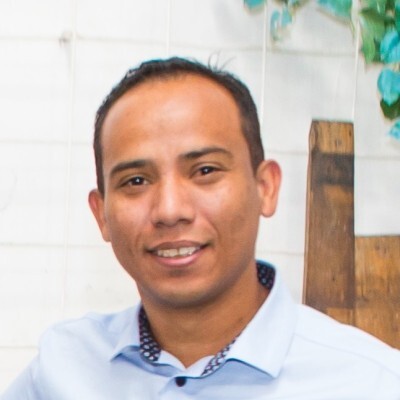
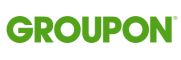