How to define a method in Ruby?
In Ruby, defining methods is a fundamental aspect of organizing and encapsulating code. Methods are blocks of code that perform specific tasks or actions and can be reused throughout your program. Here’s how you define a method in Ruby:
- Using the `def` Keyword: To define a method, you use the `def` keyword, followed by the method name, a pair of parentheses for method arguments (if any), and then the method body enclosed in a `do…end` or curly braces `{}` block. Here’s a basic method definition:
```ruby def greet(name) puts "Hello, #{name}!" end ```
In this example, we’ve defined a method called `greet` that takes one argument `name` and prints a greeting message with the provided name.
- Calling the Method: Once a method is defined, you can call it by using its name, followed by parentheses containing the required arguments (if any). Here’s how you call the `greet` method:
```ruby greet("Alice") # Outputs: "Hello, Alice!" ```
- Return Values: Methods in Ruby can return values using the `return` keyword. If no `return` statement is specified, the method returns the value of the last evaluated expression by default. For example:
```ruby def add(a, b) result = a + b return result end ```
Alternatively, you can simplify this to:
```ruby def add(a, b) a + b # The result of this expression is automatically returned end ```
- Method Visibility: By default, methods in Ruby are public, meaning they can be called from anywhere in your program. You can specify visibility using the `public`, `private`, or `protected` keywords to control the accessibility of methods within classes.
```ruby class MyClass def public_method # This method is public end private def private_method # This method is private end end ```
Defining and using methods is a fundamental concept in Ruby, allowing you to encapsulate logic, promote code reuse, and make your programs more organized and maintainable. Understanding how to create and call methods is essential for effective Ruby programming.
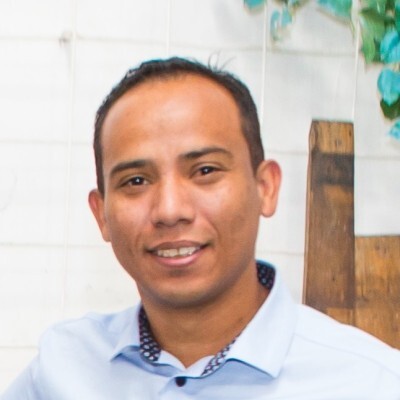
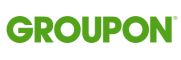