What is the ‘each’ method in Ruby?
In Ruby, the each method is an important and commonly used iterator that belongs to the Enumerable module. It is used to iterate over elements of a collection, such as an array or a hash, and execute a block of code for each element.
Here’s a basic syntax for using each:
collection.each do |element| # code to be executed for each element end
Here, collection can be an array, a range, a hash, or any other object that includes the Enumerable module. The block of code inside the do…end is executed once for each element in the collection, with the current element passed as a block parameter (in this case, element).
Here’s an example using an array:
numbers = [1, 2, 3, 4, 5] numbers.each do |number| puts "Current number is #{number}" end
This would output:
Current number is 1 Current number is 2 Current number is 3 Current number is 4 Current number is 5
You can also use each with other data structures, like hashes:
hash = { one: 1, two: 2, three: 3 } hash.each do |key, value| puts "Key: #{key}, Value: #{value}" end
This would output:
Key: one, Value: 1 Key: two, Value: 2 Key: three, Value: 3
The each method is versatile and can be used with various data structures, making it a fundamental part of Ruby programming for iterating over collections.
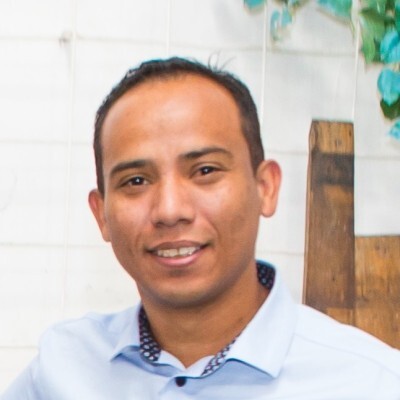
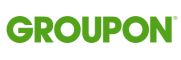