What is the ‘elsif’ statement in Ruby?
In Ruby, the `elsif` statement is an extension of the `if` statement and is used to handle multiple conditions sequentially within the same control flow structure. It’s particularly helpful when you have more than two possible outcomes based on different conditions. The `elsif` statement allows you to evaluate a series of conditions one by one until a true condition is encountered, at which point the corresponding block of code is executed. Here’s how the `elsif` statement works:
- Syntax: The `elsif` statement follows an initial `if` statement. If the condition in the `if` statement is false, Ruby checks the condition in the first `elsif` statement. If that condition is true, the code block associated with the `elsif` statement is executed. If it’s false, Ruby moves on to the next `elsif` statement and continues checking conditions until either a true condition is found, or all conditions are evaluated as false.
```ruby temperature = 25 if temperature > 30 puts "It's hot outside." elsif temperature > 20 puts "It's warm outside." elsif temperature > 10 puts "It's cool outside." else puts "It's cold outside." end ```
In this example, Ruby evaluates the conditions sequentially and prints “It’s warm outside.” because `temperature` (25) satisfies the condition in the second `elsif` statement.
- Multiple `elsif` Statements: You can use multiple `elsif` statements after the initial `if` statement to accommodate various conditions. Ruby will stop evaluating conditions as soon as it encounters a true condition and executes the corresponding block. If none of the conditions are true, the code within the `else` block (if present) will be executed as a fallback.
- No Obligation for `elsif` or `else`: You are not obligated to include `elsif` or `else` clauses. If you only need to evaluate a single condition and provide an alternative when it’s false, you can use just `if` and `else`. If you need to evaluate multiple conditions, you can use `if` and `elsif` without an `else`.
The `elsif` statement is a powerful tool for handling complex decision-making in Ruby programs, allowing you to create conditional logic that adapts to various scenarios. It provides a structured and readable way to handle multiple conditions within the same code block.
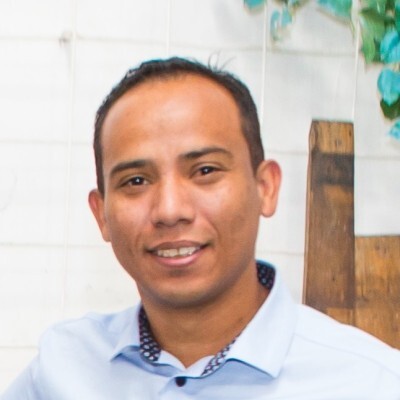
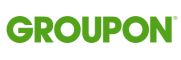