How to use environment variables in Ruby?
Using environment variables in Ruby is essential for securely storing sensitive information, configuration settings, and other variables that your application might need. Here’s a guide on how to use environment variables in Ruby:
- Setting Environment Variables:
Environment variables are typically set outside of your Ruby code to keep sensitive information like API keys, database credentials, or configuration settings secure. You can set environment variables in various ways:
– In Your Shell: If you’re working in a Unix-like environment, you can set environment variables in your shell or terminal session like this:
```bash export MY_API_KEY=your_api_key_here ```
You can then access this variable in your Ruby code.
– Using a `.env` File: For development purposes, you can use a `.env` file in your project directory to store environment variables. You’ll need a gem like `dotenv` to load these variables into your environment:
```ruby # Gemfile gem 'dotenv', '~> 3.2', require: 'dotenv/load' ```
Create a `.env` file and define your variables:
```env MY_API_KEY=your_api_key_here ```
Then, in your Ruby code, you can access them like this:
```ruby api_key = ENV['MY_API_KEY'] ```
- Accessing Environment Variables:
In your Ruby code, you can access environment variables using the `ENV` object. It behaves like a hash where the keys are variable names, and the values are their corresponding values. For example:
```ruby api_key = ENV['MY_API_KEY'] ```
This retrieves the value of the ‘MY_API_KEY’ environment variable and assigns it to the `api_key` variable.
- Using Environment Variables Securely:
Environment variables are a secure way to store sensitive information, but you should still exercise caution. Never commit your `.env` file with sensitive data to a version control system. Instead, include it in your `.gitignore` file to keep it private. On production servers, configure environment variables in your hosting environment or use a service like Heroku’s Config Vars.
By using environment variables in Ruby, you can separate configuration and sensitive information from your code, making it more secure and adaptable to different environments. This practice also ensures that your code remains consistent across development, testing, and production environments.
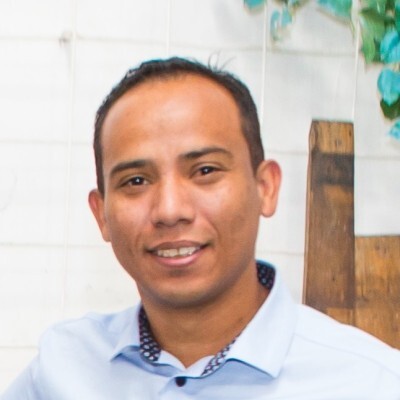
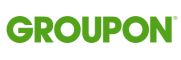