Ruby for Financial Analysis: Analyzing Market Data and Making Predictions
In today’s fast-paced financial markets, data-driven decisions are crucial for success. Whether you’re an individual investor or a financial professional, having the ability to analyze market data and make predictions can give you a competitive edge. One programming language that might not immediately come to mind for financial analysis is Ruby, but its simplicity, flexibility, and powerful libraries make it a viable choice. In this article, we’ll delve into how Ruby can be used for financial analysis, from collecting and processing market data to creating predictive models.
Table of Contents
1. Understanding the Role of Ruby in Financial Analysis
Ruby is renowned for its elegant syntax and developer-friendly features, which make it an attractive choice for a wide range of applications. While it might not be the first language that financial analysts think of, Ruby’s versatility can be harnessed effectively for financial tasks. From data scraping to statistical analysis and even machine learning, Ruby’s ecosystem is equipped with the tools you need.
2. Collecting Market Data
The first step in financial analysis is gathering reliable market data. Ruby offers a variety of libraries and tools that can assist in data collection. One popular choice is the nokogiri gem, which is excellent for web scraping. Let’s say you want to extract stock prices from a financial news website:
ruby require 'nokogiri' require 'open-uri' url = 'https://www.examplefinance.com/stocks' page = Nokogiri::HTML(URI.open(url)) stock_prices = [] page.css('.stock-price').each do |price| stock_prices << price.text.to_f end puts stock_prices
This code fetches the HTML content of the specified URL using open-uri and then uses nokogiri to parse the HTML and extract stock prices by targeting the appropriate CSS selector. It’s important to note that web scraping should always be done in compliance with the website’s terms of use.
3. Data Preprocessing and Analysis
Once you’ve collected the necessary data, it’s time to preprocess and analyze it. Ruby provides various tools for data manipulation and analysis, making it possible to perform tasks such as calculating moving averages, identifying trends, and calculating statistical measures.
ruby require 'csv' require 'statistics2' data = CSV.read('stock_prices.csv', headers: true) closing_prices = data['Close'].map(&:to_f) # Calculate simple moving average def simple_moving_average(data, window) data.each_cons(window).map { |window_data| window_data.sum / window_data.length } end sma_50 = simple_moving_average(closing_prices, 50) sma_200 = simple_moving_average(closing_prices, 200) # Calculate mean and standard deviation mean = Statistics2.mean(closing_prices) std_dev = Statistics2.standard_deviation(closing_prices) puts "50-day SMA: #{sma_50}" puts "200-day SMA: #{sma_200}" puts "Mean: #{mean}" puts "Standard Deviation: #{std_dev}"
In this example, we’re using the CSV library to read historical stock prices from a CSV file. We calculate the 50-day and 200-day simple moving averages and also compute the mean and standard deviation of the closing prices using the Statistics2 gem.
4. Creating Predictive Models
One of the most intriguing aspects of financial analysis is making predictions about future market movements. Ruby might not be the first choice that comes to mind for machine learning, but it’s more capable than you might think. The scikit-learn library provides various machine learning algorithms that can be used with Ruby, making it possible to create predictive models.
ruby require 'scikit-learn' # Sample data: features and labels features = [[2.5, 3.7], [1.5, 2.5], [3.8, 6.2], [4.5, 7.8], [6.1, 8.6]] labels = [1, 0, 1, 1, 0] # Create a Support Vector Machine classifier classifier = Scikit::Learn::SVM::SVC.new # Train the classifier classifier.fit(features, labels) # Make predictions new_data = [[3.0, 4.0], [5.0, 7.0]] predictions = classifier.predict(new_data) puts "Predictions: #{predictions}"
In this example, we’re using the scikit-learn library through the scikit-learn gem to create a Support Vector Machine classifier. We train the classifier on sample data and then use it to make predictions on new data.
5. Visualization with Ruby
Visualizing data is essential for understanding patterns and trends. Ruby offers libraries that enable you to create visually appealing graphs and charts to better comprehend your financial analysis results. One such library is gruff, which provides an easy way to generate various types of graphs.
ruby require 'gruff' # Sample data months = ['Jan', 'Feb', 'Mar', 'Apr', 'May'] revenues = [12000, 15000, 18000, 14000, 16000] # Create a bar graph bar_graph = Gruff::Bar.new bar_graph.title = 'Monthly Revenues' bar_graph.labels = { 0 => 'Jan', 1 => 'Feb', 2 => 'Mar', 3 => 'Apr', 4 => 'May' } bar_graph.data('Revenues', revenues) bar_graph.write('revenues.png')
In this example, we’re using the gruff gem to create a bar graph representing monthly revenues. The generated graph is saved as an image file named ‘revenues.png’.
Conclusion
While Ruby might not be the first language that comes to mind for financial analysis, its versatility and rich ecosystem of libraries make it a capable tool for collecting, processing, and analyzing market data. From web scraping and data preprocessing to building predictive models and creating visualizations, Ruby can empower you to make informed decisions in the complex world of financial markets. So, whether you’re an individual investor or a financial professional, consider adding Ruby to your toolkit for comprehensive and effective financial analysis.
Table of Contents
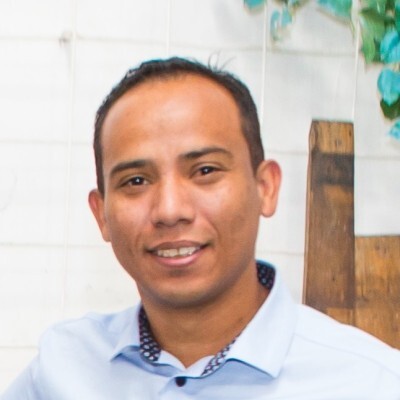
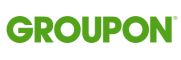