How to find the length of a string in Ruby?
In Ruby, finding the length of a string is a straightforward task, and you can achieve it using several methods:
- Using the `.length` Method:
Ruby provides a built-in method called `.length` that allows you to determine the length of a string. You can simply call this method on a string to obtain its length.
```ruby text = "Hello, World!" length = text.length ```
The variable `length` will contain the value `13`, which is the length of the string `text`.
- Using the `.size` Method:
Another built-in method, `.size`, can be used interchangeably with `.length` to find the length of a string.
```ruby text = "Hello, World!" size = text.size ```
The variable `size` will also contain the value `13`.
- Using String Indexing:
You can find the length of a string by iterating through its characters and counting them.
```ruby text = "Hello, World!" length = 0 text.each_char { length += 1 } ```
The variable `length` will store the length of the string.
All of these methods are effective in determining the length of a string in Ruby. The `.length` and `.size` methods are the most commonly used and are preferred for their simplicity and readability. Using these methods is a standard practice in Ruby for finding the length of strings and other enumerable objects. Depending on your coding style and requirements, you can choose the method that best suits your needs.
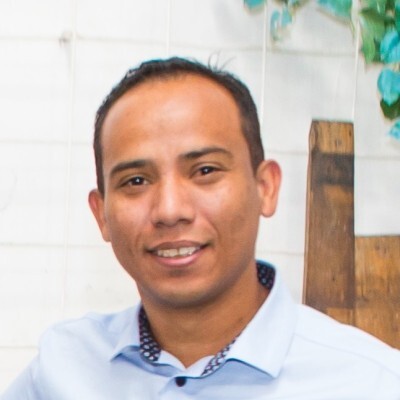
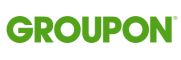