Ruby Q & A
How to generate documentation for Ruby code?
Generating documentation for Ruby code is essential for maintaining and sharing your codebase effectively. Documentation provides insights into how your code works, making it easier for developers (including yourself) to understand, use, and maintain your Ruby projects. Ruby offers several tools and conventions for generating documentation:
- RDoc: RDoc is a built-in documentation generator for Ruby. You can add comments directly to your Ruby code using special formatting conventions, such as `#` for comments and `#:nodoc:` to exclude specific items from documentation. To generate documentation, run `rdoc` from the command line in your project directory. This will create HTML documentation files that you can browse to understand your code better.
```ruby # Example documentation in Ruby code # # This class represents a person with a name and age. class Person # Create a new Person object. # # @param name [String] the person's name # @param age [Integer] the person's age def initialize(name, age) @name = name @age = age end end ```
- YARD: YARD (Yet Another Ruby Documentation) is a popular alternative to RDoc. It provides more extensive features and flexibility for documenting Ruby code. YARD uses special tags and conventions to document code. To generate documentation with YARD, you need to install the YARD gem and use the `yardoc` command. YARD can generate HTML documentation and supports additional output formats.
```ruby # Example documentation using YARD # # @!attribute [r] name # @return [String] the person's name # @!attribute [r] age # @return [Integer] the person's age class Person attr_reader :name, :age # Create a new Person object. # # @param name [String] the person's name # @param age [Integer] the person's age def initialize(name, age) @name = name @age = age end end ```
- GitHub and GitLab Integration: If your Ruby project is hosted on GitHub or GitLab, you can leverage their built-in documentation features. By adding README files and using Markdown or other supported markup languages, you can create comprehensive project documentation that is easy to access and update.
Generating and maintaining documentation for your Ruby code is essential for enhancing codebase readability and maintainability. It helps both you and other developers understand the purpose, functionality, and usage of your code. Whether you choose RDoc, YARD, or platform-specific documentation tools, documenting your code is a best practice that contributes to the success of your Ruby projects.
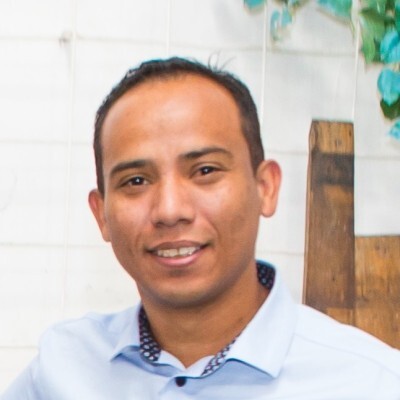
Previously at
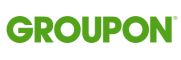
Experienced software professional with a strong focus on Ruby. Over 10 years in software development, including B2B SaaS platforms and geolocation-based apps.